怎么让该棋子被点击后,获取其他类里面声明的movablecellprotected void paintComponent(Graphics g) { try { if (owner.getColor() == Color.BLUE) { File file = new File("src/view/bluedog.png"); imagedog = ImageIO.read(file); } else if (owner.getColor() == Color.RED) { File file = new File("src/view/reddog.png"); imagedog = ImageIO.read(file); } } catch(IOException e){ e.printStackTrace(); } super.paintComponent(g); Graphics2D g2 = (Graphics2D) g; g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); if (imagedog != null) { // 判断是否有导入的图片 g2.drawImage(imagedog, 0, 0, getWidth(), getHeight(), null); } if (isSelected()) { // Highlights the model if selected. draw(g); } }
时间: 2024-01-02 10:04:32 浏览: 71
可以在该棋子的监听器类中,调用该棋子的paintComponent方法,并传入Graphics对象,例如:
```
public class ChessPieceListener implements MouseListener {
private ChessPiece chessPiece;
// 其他代码
@Override
public void mouseClicked(MouseEvent e) {
// 获取其他类里面声明的movablecell
List<MovableCell> movableCells = chessPiece.getOwner().getMovableCells(chessPiece);
// 处理点击事件
// ...
// 调用paintComponent方法,并传入Graphics对象
chessPiece.paintComponent(chessPiece.getGraphics());
}
}
```
需要注意的是,调用paintComponent方法时,需要确保该棋子已经被添加到了容器中。
相关问题
怎么修改这段代码,使其能用GUI导入图片代替文字“猫”protected void paintComponent(Graphics g) { super.paintComponent(g); Graphics2D g2 = (Graphics2D) g; g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); Font font = new Font("楷体", Font.PLAIN, getWidth() / 2); g2.setFont(font); g2.setColor(owner.getColor()); g2.drawString("猫", getWidth() / 4, getHeight() * 5 / 8); // FIXME: Use library to find the correct offset. if (isSelected()) { // Highlights the model if selected. g.setColor(Color.RED); g.drawOval(0, 0, getWidth() , getHeight()); } }
可以将文字“猫”替换为导入的图片。具体步骤如下:
1. 在类的属性中添加一个 BufferedImage 类型的变量 image,用于存储导入的图片。
2. 在类的构造函数或初始化方法中,使用 ImageIO.read() 方法加载图片并赋值给 image 变量。
3. 在 paintComponent() 方法中,使用 g2.drawImage() 方法绘制图片,替代原来的绘制文字的代码。具体代码如下:
```
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2 = (Graphics2D) g;
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
if (image != null) { // 判断是否有导入的图片
g2.drawImage(image, 0, 0, getWidth(), getHeight(), null);
}
if (isSelected()) { // Highlights the model if selected.
g.setColor(Color.RED);
g.drawOval(0, 0, getWidth(), getHeight());
}
}
```
注意:这段代码中使用了 getWidth() 和 getHeight() 方法获取组件的宽度和高度,所以在使用前需要确保组件已经被添加到容器中并且已经设置了正确的大小。
public void paintComponent(Graphics g) { super.paintComponent(g); // 绘制图片 g.drawImage(image, x, y, null); }中Graphics g是什么
Graphics g 是 Java AWT(Graphics Abstract Window Toolkit) 绘图的一个抽象类,它提供了一组绘制图形的方法,用于在 Java GUI 程序中绘制图形。在这里,我们使用 Graphics 对象的 drawImage() 方法绘制图像。当我们需要绘制图形时,可以通过重写 paintComponent() 方法,使用 Graphics 对象进行绘制。在这个方法中,g 参数就是一个 Graphics 对象,我们可以通过这个对象进行图形的绘制。
阅读全文
相关推荐
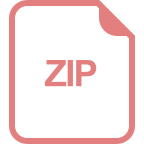
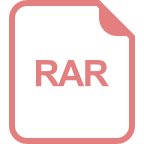














