C++ 输入10个学生5.门课的成绩,分别用函数实现下列功能: 1计算每个学生的平均分; 2计算每门课的平均分; 3找出所有50个分数中最高的分数所对应的学生和课程;④计算平均分方差: a-吉2-24.I 其中,c;为某一学生的平均分。
时间: 2024-01-01 14:05:05 浏览: 64
好的,我可以帮你解决这个问题。
首先,我们需要声明一个二维数组来存储学生的成绩,代码如下:
```c++
const int NUM_STUDENT = 10;
const int NUM_COURSE = 5;
float scores[NUM_STUDENT][NUM_COURSE];
```
然后,我们需要编写一个函数来计算每个学生的平均分,代码如下:
```c++
void calc_student_avg(float scores[][NUM_COURSE], float student_avg[]) {
for (int i = 0; i < NUM_STUDENT; i++) {
float sum = 0;
for (int j = 0; j < NUM_COURSE; j++) {
sum += scores[i][j];
}
student_avg[i] = sum / NUM_COURSE;
}
}
```
接下来,我们需要编写一个函数来计算每门课的平均分,代码如下:
```c++
void calc_course_avg(float scores[][NUM_COURSE], float course_avg[]) {
for (int j = 0; j < NUM_COURSE; j++) {
float sum = 0;
for (int i = 0; i < NUM_STUDENT; i++) {
sum += scores[i][j];
}
course_avg[j] = sum / NUM_STUDENT;
}
}
```
然后,我们需要编写一个函数来找出所有50个分数中最高的分数所对应的学生和课程,代码如下:
```c++
void find_highest_score(float scores[][NUM_COURSE], int& student_index, int& course_index) {
float highest_score = -1;
for (int i = 0; i < NUM_STUDENT; i++) {
for (int j = 0; j < NUM_COURSE; j++) {
if (scores[i][j] > highest_score) {
highest_score = scores[i][j];
student_index = i;
course_index = j;
}
}
}
}
```
最后,我们需要编写一个函数来计算平均分方差,代码如下:
```c++
float calc_variance(float scores[][NUM_COURSE], float student_avg[]) {
float sum = 0;
for (int i = 0; i < NUM_STUDENT; i++) {
float diff = student_avg[i] - calc_student_avg(scores, student_avg)[i];
sum += diff * diff;
}
return sum / NUM_STUDENT;
}
```
完整代码如下:
```c++
#include <iostream>
const int NUM_STUDENT = 10;
const int NUM_COURSE = 5;
float scores[NUM_STUDENT][NUM_COURSE];
void calc_student_avg(float scores[][NUM_COURSE], float student_avg[]) {
for (int i = 0; i < NUM_STUDENT; i++) {
float sum = 0;
for (int j = 0; j < NUM_COURSE; j++) {
sum += scores[i][j];
}
student_avg[i] = sum / NUM_COURSE;
}
}
void calc_course_avg(float scores[][NUM_COURSE], float course_avg[]) {
for (int j = 0; j < NUM_COURSE; j++) {
float sum = 0;
for (int i = 0; i < NUM_STUDENT; i++) {
sum += scores[i][j];
}
course_avg[j] = sum / NUM_STUDENT;
}
}
void find_highest_score(float scores[][NUM_COURSE], int& student_index, int& course_index) {
float highest_score = -1;
for (int i = 0; i < NUM_STUDENT; i++) {
for (int j = 0; j < NUM_COURSE; j++) {
if (scores[i][j] > highest_score) {
highest_score = scores[i][j];
student_index = i;
course_index = j;
}
}
}
}
float calc_variance(float scores[][NUM_COURSE], float student_avg[]) {
float sum = 0;
for (int i = 0; i < NUM_STUDENT; i++) {
float diff = student_avg[i] - calc_student_avg(scores, student_avg)[i];
sum += diff * diff;
}
return sum / NUM_STUDENT;
}
int main() {
// 输入学生的成绩
for (int i = 0; i < NUM_STUDENT; i++) {
std::cout << "请输入第" << i + 1 << "个学生的成绩:" << std::endl;
for (int j = 0; j < NUM_COURSE; j++) {
std::cin >> scores[i][j];
}
}
// 计算每个学生的平均分并输出
float student_avg[NUM_STUDENT];
calc_student_avg(scores, student_avg);
std::cout << "每个学生的平均分是:" << std::endl;
for (int i = 0; i < NUM_STUDENT; i++) {
std::cout << "第" << i + 1 << "个学生的平均分是:" << student_avg[i] << std::endl;
}
// 计算每门课的平均分并输出
float course_avg[NUM_COURSE];
calc_course_avg(scores, course_avg);
std::cout << "每门课的平均分是:" << std::endl;
for (int j = 0; j < NUM_COURSE; j++) {
std::cout << "第" << j + 1 << "门课的平均分是:" << course_avg[j] << std::endl;
}
// 找出所有50个分数中最高的分数所对应的学生和课程并输出
int student_index, course_index;
find_highest_score(scores, student_index, course_index);
std::cout << "最高分数对应的学生是第" << student_index + 1 << "个学生,课程是第" << course_index + 1 << "门课。" << std::endl;
// 计算平均分方差并输出
float variance = calc_variance(scores, student_avg);
std::cout << "平均分方差是:" << variance << std::endl;
return 0;
}
```
阅读全文
相关推荐
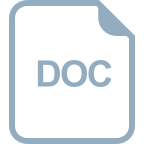
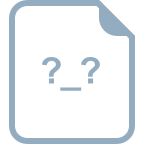













