用QT实现棋盘覆盖算法
时间: 2023-08-15 14:26:14 浏览: 162
好的,下面是一个用QT实现棋盘覆盖算法的示例代码。在这个示例中,我们使用了一个QGraphicsScene和一个QGraphicsView来绘制棋盘。
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QGraphicsRectItem>
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 创建一个QGraphicsScene对象
QGraphicsScene *scene = new QGraphicsScene(this);
scene->setSceneRect(0, 0, 600, 600);
// 创建一个QGraphicsView对象,并将其设置为主窗口的中心控件
QGraphicsView *view = new QGraphicsView(scene, this);
view->setRenderHint(QPainter::Antialiasing);
view->setViewportUpdateMode(QGraphicsView::FullViewportUpdate);
setCentralWidget(view);
// 调用绘制棋盘的函数
drawChessBoard(scene, 0, 0, 600, 600, 3, 2);
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::drawChessBoard(QGraphicsScene *scene, int x, int y, int width, int height, int special_x, int special_y, int size)
{
if (size == 1) {
// 如果棋盘大小为1,直接绘制一个黑色方块
QGraphicsRectItem *rect = new QGraphicsRectItem(x, y, width, height);
rect->setBrush(Qt::black);
scene->addItem(rect);
return;
}
// 计算出子棋盘的大小
int subWidth = width / 2;
int subHeight = height / 2;
// 覆盖特殊方块所在的子棋盘
int sub_x = x + subWidth * special_x;
int sub_y = y + subHeight * special_y;
// 左上子棋盘
if (special_x < subWidth && special_y < subHeight) {
drawChessBoard(scene, x, y, subWidth, subHeight, special_x, special_y, size / 2);
} else {
QGraphicsRectItem *rect = new QGraphicsRectItem(x, y, subWidth, subHeight);
rect->setBrush(Qt::black);
scene->addItem(rect);
}
// 右上子棋盘
if (special_x >= subWidth && special_y < subHeight) {
drawChessBoard(scene, x + subWidth, y, subWidth, subHeight, special_x - subWidth, special_y, size / 2);
} else {
QGraphicsRectItem *rect = new QGraphicsRectItem(x + subWidth, y, subWidth, subHeight);
rect->setBrush(Qt::black);
scene->addItem(rect);
}
// 左下子棋盘
if (special_x < subWidth && special_y >= subHeight) {
drawChessBoard(scene, x, y + subHeight, subWidth, subHeight, special_x, special_y - subHeight, size / 2);
} else {
QGraphicsRectItem *rect = new QGraphicsRectItem(x, y + subHeight, subWidth, subHeight);
rect->setBrush(Qt::black);
scene->addItem(rect);
}
// 右下子棋盘
if (special_x >= subWidth && special_y >= subHeight) {
drawChessBoard(scene, x + subWidth, y + subHeight, subWidth, subHeight, special_x - subWidth, special_y - subHeight, size / 2);
} else {
QGraphicsRectItem *rect = new QGraphicsRectItem(x + subWidth, y + subHeight, subWidth, subHeight);
rect->setBrush(Qt::black);
scene->addItem(rect);
}
}
```
在这个示例中,我们通过调用drawChessBoard函数来绘制棋盘,该函数接受棋盘的位置、大小、特殊块的位置以及棋盘的大小等参数。在函数中,我们通过递归调用来绘制棋盘,直到棋盘被完全覆盖。在每次递归调用中,我们需要根据特殊块的位置来决定需要覆盖的子棋盘。最终,我们通过QGraphicsView来显示绘制好的棋盘。
阅读全文
相关推荐
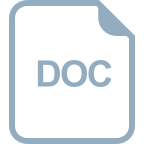
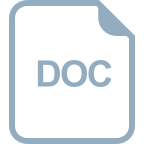
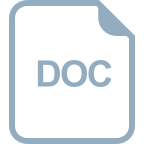





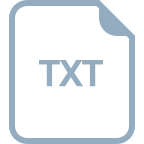

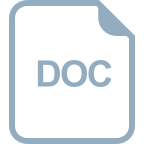
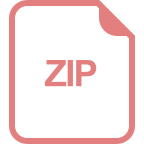
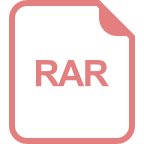
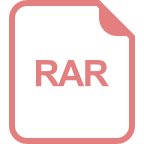

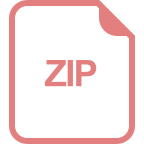
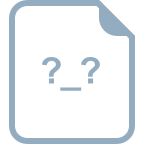
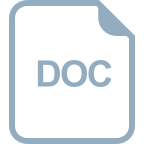
