机械臂路径规划走圆代码
时间: 2024-05-06 07:13:34 浏览: 12
机械臂路径规划是指在给定起始点和目标点的情况下,通过算法确定机械臂的运动轨迹,使其能够从起始点平滑地移动到目标点。下面是一个简单的机械臂路径规划走圆的代码示例:
```python
import numpy as np
def circular_path_planning(start_point, center_point, radius, num_points):
# 计算圆心到起始点的向量
start_to_center = center_point - start_point
# 计算起始点到圆心的距离
distance = np.linalg.norm(start_to_center)
# 计算起始点到圆心的单位向量
start_to_center_unit = start_to_center / distance
# 计算起始点到圆心的垂直向量
start_to_center_perpendicular = np.array([-start_to_center_unit, start_to_center_unit])
# 计算每个角度对应的点
angles = np.linspace(0, 2*np.pi, num_points)
points = []
for angle in angles:
# 计算当前角度对应的点在圆上的位置
point_on_circle = center_point + radius * (np.cos(angle) * start_to_center_unit + np.sin(angle) * start_to_center_perpendicular)
points.append(point_on_circle)
return points
# 示例使用
start_point = np.array([0, 0])
center_point = np.array([1, 1])
radius = 0.5
num_points = 10
path_points = circular_path_planning(start_point, center_point, radius, num_points)
for point in path_points:
print(point)
```
这段代码实现了一个简单的机械臂路径规划走圆的功能。它通过给定起始点、圆心、半径和点的数量,计算出沿着圆形轨迹的一系列点,并将其打印出来。
相关推荐
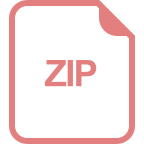
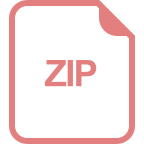
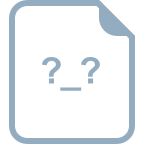














