print(test.a) AttributeError: module 'test' has no attribute 'a'
时间: 2023-11-11 16:05:22 浏览: 154
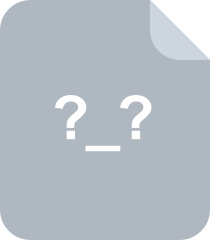
AttributeError: module 'tensorflow.compat.v1' has no attribute '
这个错误提示表明在模块 test 中没有名为 a 的属性。可能的原因是在 test 模块中没有定义 a,或者 a 是一个私有属性,无法从外部访问。
如果想要访问 test 模块中的属性,可以使用以下语法:
```
import test
print(test.attribute_name)
```
其中 attribute_name 是要访问的属性名称。
阅读全文
相关推荐
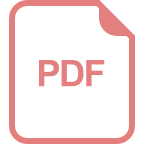
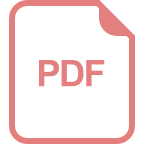















