构造一个顺序表实现构造,查找,插入,删除等功能c++
时间: 2024-09-18 09:15:29 浏览: 48
在C++中,我们可以使用数组或者动态内存管理的方式来实现一个简单的顺序表。这里我会提供一个基于数组的示例,因为数组构造简单并且易于理解。这个顺序表将包含一个整数数组以及对应的方法来操作数据。
```cpp
template <typename T>
class SequentialList {
private:
T* elements; // 存储元素的指针
int size; // 当前元素的数量
int capacity; // 容量(最大存储元素数量)
public:
// 构造函数,初始化一个空列表
SequentialList(int capacity = 10) : elements(new T[capacity]), size(0), capacity(capacity) {}
~SequentialList() { // 析构函数,释放内存
delete[] elements;
}
// 查找元素
bool find(T value) const {
for (int i = 0; i < size; ++i) {
if (elements[i] == value) return true;
}
return false;
}
// 插入元素
void insert(int index, const T& value) {
if (index >= 0 && index <= size) {
std::memmove(&elements[index + 1], &elements[index], sizeof(T) * (size - index));
elements[index] = value;
size++;
} else {
throw std::out_of_range("Index out of range");
}
}
// 删除元素
void remove(const T& value) {
for (int i = 0; i < size; ++i) {
if (elements[i] == value) {
std::copy(&elements[i+1], &elements[size], &elements[i]);
elements[size-1] = nullptr; // 或者设置为默认值
size--;
break;
}
}
}
// 显示列表内容
void display() const {
std::cout << "Elements in the list: ";
for (int i = 0; i < size; ++i) {
std::cout << elements[i] << " ";
}
std::cout << std::endl;
}
};
// 使用示例
int main() {
SequentialList<int> list(5);
list.insert(0, 10);
list.insert(2, 20);
list.display(); // 输出: Elements in the list: 10 0 20
if (list.find(20)) {
std::cout << "Found 20" << std::endl;
}
list.remove(20);
list.display(); // 输出: Elements in the list: 10 0
return 0;
}
```
阅读全文
相关推荐
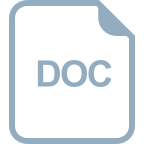
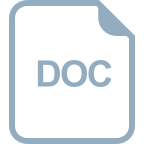
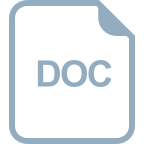
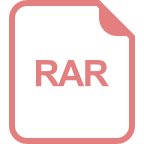
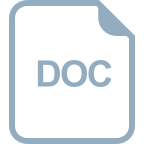
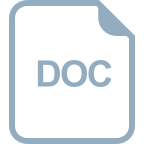












