C++如何判断文件读入内容是中文回文
时间: 2024-10-11 18:11:03 浏览: 23
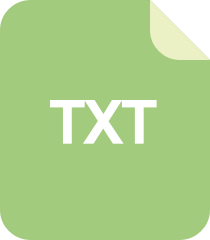
回文素数.txt
在C++中,判断文件输入内容是否为中文回文需要先读取文件到字符串,然后对每个字符进行分析。因为中文字符通常不是单字节,而是由多个UTF-8或UTF-16编码的字节组成的,所以我们需要处理多字节字符。
以下是一个基本的步骤示例:
1. 打开并读取文件:
```cpp
std::ifstream file("input.txt", std::ios::binary);
if (!file.is_open()) {
// 文件打开失败,处理错误
}
std::string content((std::istreambuf_iterator<char>(file)), {});
file.close();
```
2. 将多字节字符转换为宽字符(例如对于UTF-16),方便后续处理:
```cpp
std::wstring_convert<std::codecvt_utf16<wchar_t>> convert;
std::wstring wstr = convert.from_bytes(content);
```
3. 检查回文:
```cpp
bool is_palindrome = true;
size_t length = wstr.length();
for (size_t i = 0; i < length / 2; ++i) {
if (wstr[i] != wstr[length - i - 1]) {
is_palindrome = false;
break;
}
}
```
4. 输出结果:
```cpp
if (is_palindrome) {
std::wcout << L"文件内容是中文回文。\n";
} else {
std::wcout << L"文件内容不是中文回文。\n";
}
```
阅读全文
相关推荐
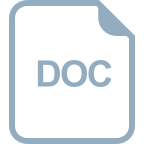
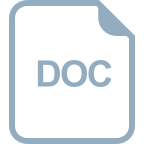
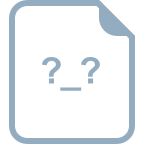














