class MLP(nn.Module): def __init__(self, input_dim, fc_dims, dropout_p=0.4, use_batchnorm=False): super(MLP, self).__init__() if isinstance(fc_dims, Iterable): fc_dims = list(fc_dims) assert isinstance(fc_dims, (list, tuple)), 'fc_dims must be either a list or a tuple, but got {}'.format( type(fc_dims)) layers = [] for dim in fc_dims: layers.append(nn.Linear(input_dim, dim)) if use_batchnorm and dim != 1: layers.append(nn.BatchNorm1d(dim)) if dim != 1: layers.append(nn.ReLU(inplace=True)) if dropout_p is not None and dim != 1: layers.append(nn.Dropout(p=dropout_p)) input_dim = dim self.fc_layers = nn.Sequential(*layers) def forward(self, input): return self.fc_layers(input)
时间: 2024-04-16 22:25:54 浏览: 9
这是一个多层感知机(MLP)的定义,它是一个继承自`nn.Module`的类。MLP是一种前馈神经网络,由多个全连接层组成。
在构造函数`__init__`中,我们接受输入维度`input_dim`、隐藏层维度列表`fc_dims`、dropout概率`dropout_p`和是否使用批归一化`use_batchnorm`作为参数。
我们首先判断`fc_dims`是否是可迭代的对象,如果是,则将其转换为列表。然后,我们定义一个空的列表`layers`来存储网络的层。
接下来,我们遍历隐藏层维度列表`fc_dims`,对于每个维度,我们创建一个全连接层,并将其添加到`layers`列表中。如果使用批归一化且维度不为1,则添加一个批归一化层。然后,我们添加一个ReLU激活函数层(除非维度为1)。如果指定了dropout概率且维度不为1,则添加一个dropout层。最后,将当前维度更新为下一个维度。
最后,我们使用`nn.Sequential`将所有层组合成一个顺序模型,并将其赋值给类的属性`self.fc_layers`。
在前向传播函数`forward`中,我们将输入通过所有的全连接层,然后返回输出。
相关问题
class Mlp(nn.Module): def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.): super().__init__() out_features = out_features or in_features hidden_features = hidden_features or in_features self.fc1 = nn.Linear(in_features, hidden_features) self.act = act_layer() self.fc2 = nn.Linear(hidden_features, out_features) self.drop = nn.Dropout(drop)是什么意思
这段代码定义了一个多层感知机(Multi-Layer Perceptron,MLP)的神经网络模型。下面是每个部分的解释:
- `class Mlp(nn.Module):`:定义了一个名为`Mlp`的类,并继承自`nn.Module`,这意味着它是一个PyTorch模型。
- `def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.):`:定义了类的初始化函数,用于初始化模型的参数。
- `super().__init__()`:调用父类(`nn.Module`)的初始化函数。
- `out_features = out_features or in_features`和`hidden_features = hidden_features or in_features`:如果未指定输出特征和隐藏特征的数量,则将它们设置为输入特征的数量。
- `self.fc1 = nn.Linear(in_features, hidden_features)`:定义了一个全连接层(`nn.Linear`),它将输入特征映射到隐藏特征。
- `self.act = act_layer()`:定义激活函数层,这里使用的是`act_layer`参数指定的激活函数(默认为`nn.GELU`)。
- `self.fc2 = nn.Linear(hidden_features, out_features)`:定义了另一个全连接层,它将隐藏特征映射到输出特征。
- `self.drop = nn.Dropout(drop)`:定义了一个Dropout层,用于在训练过程中随机丢弃一部分神经元,以减少过拟合风险。
这段代码的作用是创建一个MLP模型,并定义了模型的结构和参数。具体的使用方式需要根据实际情况进行调用和训练。
class Mlp(nn.Module): def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.): super().__init__() out_features = out_features or in_features hidden_features = hidden_features or in_features self.fc1 = nn.Linear(in_features, hidden_features) self.act = act_layer() self.fc2 = nn.Linear(hidden_features, out_features) self.drop = nn.Dropout(drop) def forward(self, x): x = self.fc1(x) x = self.act(x) x = self.drop(x) x = self.fc2(x) x = self.drop(x) return x
这是一个 PyTorch 中定义的简单多层感知机(MLP)模型。下面是对这段代码的解释:
- `class Mlp(nn.Module):`:定义了一个名为 Mlp 的类,继承自 nn.Module,即是一个 PyTorch 模型。
- `def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.):`:定义了 Mlp 类的构造函数,其中 `in_features` 表示输入特征的大小,`hidden_features` 表示隐藏层的大小(默认为 `in_features`),`out_features` 表示输出特征的大小(默认为 `in_features`),`act_layer` 表示激活函数(默认为 GELU),`drop` 表示 dropout 的概率(默认为 0)。
- `super().__init__()`:调用父类 nn.Module 的构造函数。
- `out_features = out_features or in_features`:如果没有指定 `out_features`,则将其设为 `in_features`。
- `hidden_features = hidden_features or in_features`:如果没有指定 `hidden_features`,则将其设为 `in_features`。
- `self.fc1 = nn.Linear(in_features, hidden_features)`:定义一个全连接层,输入大小为 `in_features`,输出大小为 `hidden_features`。
- `self.act = act_layer()`:定义一个激活函数,使用 `act_layer`。
- `self.fc2 = nn.Linear(hidden_features, out_features)`:定义一个全连接层,输入大小为 `hidden_features`,输出大小为 `out_features`。
- `self.drop = nn.Dropout(drop)`:定义一个 dropout 层,概率为 `drop`。
- `def forward(self, x):`:定义了模型的前向传播函数。
- `x = self.fc1(x)`:输入特征经过第一个全连接层。
- `x = self.act(x)`:激活函数。
- `x = self.drop(x)`:dropout。
- `x = self.fc2(x)`:输入特征经过第二个全连接层。
- `x = self.drop(x)`:dropout。
- `return x`:返回最终的输出特征。
相关推荐
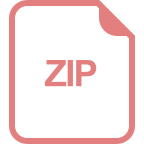
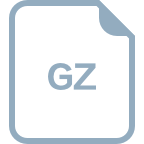













