class Transformer(nn.Module): def __init__(self, dim, depth, heads, dim_head, mlp_dim, dropout = 0.): super().__init__() self.layers = nn.ModuleList([]) for _ in range(depth): self.layers.append(nn.ModuleList([ PreNorm(dim, Attention(dim, heads = heads, dim_head = dim_head, dropout = dropout)), PreNorm(dim, FeedForward(dim, mlp_dim, dropout = dropout)) ])) def forward(self, x): for attn, ff in self.layers: x = attn(x) + x x = ff(x) + x return x 每句语句什莫意思
时间: 2023-03-09 13:02:59 浏览: 359
Transformer 是一种神经网络模型,用于处理自然语言处理任务,它的架构可以提供有效的语言表示。它的核心是一系列的注意力机制,它们可以捕捉不同输入之间的关联,并使用多头注意力和前馈网络来更新输入的表示。
相关问题
Swin Transformer model代码
以下是Swin Transformer的PyTorch代码实现,包括Swin Transformer的模型定义和训练过程:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class SwinBlock(nn.Module):
"""Swin Transformer Block"""
def __init__(self, dim, num_heads, window_size=7, shift_size=0):
super().__init__()
self.norm1 = nn.LayerNorm(dim)
self.attn = nn.MultiheadAttention(dim, num_heads)
self.norm2 = nn.LayerNorm(dim)
self.mlp = nn.Sequential(
nn.Linear(dim, dim * 4),
nn.GELU(),
nn.Linear(dim * 4, dim),
)
self.window_size = window_size
self.shift_size = shift_size
if window_size == 1 and shift_size == 0:
self.window_attn = None
else:
self.window_attn = nn.MultiheadAttention(dim, num_heads)
def forward(self, x):
res = x
x = self.norm1(x)
if self.window_attn is not None:
b, n, d = x.shape
assert n % self.window_size == 0, "sequence length must be divisible by window size"
x = x.reshape(b, n // self.window_size, self.window_size, d)
x = x.permute(0, 2, 1, 3)
x = x.reshape(b * self.window_size, n // self.window_size, d)
window_res = x
x = self.window_attn(x, x, x)[0]
x = x.reshape(b, self.window_size, n // self.window_size, d)
x = x.permute(0, 2, 1, 3)
x = x.reshape(b, n, d)
x += window_res
x = x + self.attn(x, x, x)[0]
x = res + x
res = x
x = self.norm2(x)
x = x + self.mlp(x)
x = res + x
if self.shift_size > 0:
x = F.pad(x, (0, 0, 0, 0, self.shift_size, 0))
x = x[:, :-self.shift_size, :]
return x
class SwinTransformer(nn.Module):
"""Swin Transformer Model"""
def __init__(self, img_size, patch_size, in_chans, num_classes, embed_dim, depths, num_heads, window_size=7, shift_size=0):
super().__init__()
assert img_size % patch_size == 0, "image size must be divisible by patch size"
num_patches = (img_size // patch_size) ** 2
patch_dim = in_chans * patch_size ** 2
self.patch_size = patch_size
# Patch Embeddings
self.patch_embed = nn.Conv2d(in_chans, embed_dim, kernel_size=patch_size, stride=patch_size)
self.pos_embed = nn.Parameter(torch.zeros(1, num_patches, embed_dim))
# Stages
self.stages = nn.ModuleList([
nn.Sequential(*[
SwinBlock(embed_dim, num_heads, window_size, shift_size)
for _ in range(depth)
])
for depth in depths
])
# Classifier Head
self.norm = nn.LayerNorm(embed_dim)
self.avgpool = nn.AdaptiveAvgPool2d(1)
self.fc = nn.Linear(embed_dim, num_classes)
def forward(self, x):
x = self.patch_embed(x)
b, c, h, w = x.shape
x = x.flatten(2).transpose(1, 2)
x = x + self.pos_embed
x = self.norm(x)
for stage in self.stages:
x = stage(x)
x = self.avgpool(x.transpose(1, 2).reshape(b, c, h // self.patch_size, w // self.patch_size))
x = x.flatten(1)
x = self.fc(x)
return x
```
训练过程:
```python
import torch.optim as optim
# Define Model
model = SwinTransformer(
img_size=224,
patch_size=4,
in_chans=3,
num_classes=10,
embed_dim=96,
depths=[2, 2, 6, 2],
num_heads=6,
window_size=7,
shift_size=2,
)
model.cuda()
# Define Loss and Optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01, momentum=0.9)
# Train Model
for epoch in range(num_epochs):
running_loss = 0.0
for i, data in enumerate(trainloader, 0):
inputs, labels = data
inputs, labels = inputs.cuda(), labels.cuda()
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch+1}: Training Loss = {running_loss/len(trainloader)}")
```
编写pytorch代码搭建Vision Transformer模型
好的,关于Vision Transformer模型的搭建,我可以给你一些指导。
首先,你需要导入PyTorch和一些其他的必要库。下面是一个简单的示例:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from einops.layers.torch import Rearrange
```
接下来,你需要定义模型的各个模块。一个标准的Vision Transformer模型由多个Transformer层组成,每个Transformer层包含了多头自注意力机制、前馈网络和残差连接。下面是一个简单的Transformer层的示例:
```python
class TransformerBlock(nn.Module):
def __init__(self, embed_dim, num_heads, dropout=0.1):
super().__init__()
self.attention = nn.MultiheadAttention(embed_dim, num_heads)
self.norm1 = nn.LayerNorm(embed_dim)
self.dropout1 = nn.Dropout(dropout)
self.fc = nn.Sequential(
nn.Linear(embed_dim, 4 * embed_dim),
nn.GELU(),
nn.Linear(4 * embed_dim, embed_dim),
nn.Dropout(dropout)
)
self.norm2 = nn.LayerNorm(embed_dim)
self.dropout2 = nn.Dropout(dropout)
def forward(self, x):
attn_output, _ = self.attention(x, x, x)
x = self.norm1(x + self.dropout1(attn_output))
fc_output = self.fc(x)
x = self.norm2(x + self.dropout2(fc_output))
return x
```
接下来,你需要将多个Transformer层堆叠起来组成一个完整的Vision Transformer模型。这个示例中,我们还添加了一个可训练的分类头用于图像分类任务:
```python
class VisionTransformer(nn.Module):
def __init__(self, num_classes, image_size=224, patch_size=16, embed_dim=768, depth=12, num_heads=12, mlp_ratio=4., dropout=0.1):
super().__init__()
assert image_size % patch_size == 0, "Image size must be divisible by patch size."
num_patches = (image_size // patch_size) ** 2
patch_dim = 3 * patch_size ** 2
self.patch_embedding = nn.Sequential(
Rearrange('b c (h p1) (w p2) -> b (h w) (p1 p2 c)', p1=patch_size, p2=patch_size),
nn.Linear(patch_dim, embed_dim),
nn.Dropout(dropout)
)
self.positional_encoding = nn.Parameter(torch.zeros(1, num_patches + 1, embed_dim))
self.cls_token = nn.Parameter(torch.zeros(1, 1, embed_dim))
self.transformer_blocks = nn.ModuleList([
TransformerBlock(embed_dim, num_heads, dropout) for _ in range(depth)
])
self.mlp_head = nn.Sequential(
nn.LayerNorm(embed_dim),
nn.Linear(embed_dim, int(embed_dim * mlp_ratio)),
nn.GELU(),
nn.Dropout(dropout),
nn.Linear(int(embed_dim * mlp_ratio), num_classes)
)
def forward(self, x):
b = x.shape[0]
x = self.patch_embedding(x)
cls_tokens = self.cls_token.expand(b, -1, -1)
x = torch.cat((cls_tokens, x), dim=1)
x += self.positional_encoding[:, :(x.shape[1]), :]
for transformer_block in self.transformer_blocks:
x = transformer_block(x)
x = x.mean(dim=1)
x = self.mlp_head(x)
return x
```
最后,你可以实例化该模型并传递输入数据来进行推理或训练:
```python
model = VisionTransformer(num_classes=10)
input_data = torch.randn((1, 3, 224, 224))
output = model(input_data)
```
希望这能够帮助到你。
阅读全文
相关推荐
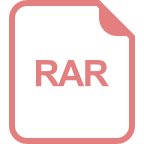
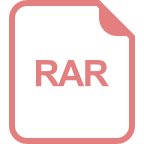
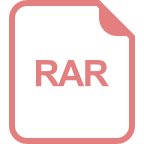
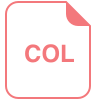
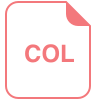
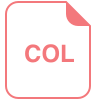
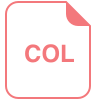
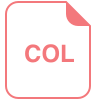


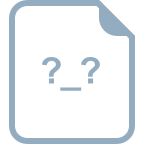