要求:已知plant.txt的路径为“data_edit/plant.txt”,从plant.txt中读取植物的基本信息,创建一个植物信息的链表,基于该链表,实现植物基本信息的增加功能。请你给出c语言代码
时间: 2024-02-07 14:02:49 浏览: 41
好的,根据您的要求,我可以为您提供以下 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Plant {
char name[50];
char family[50];
char genus[50];
char species[50];
char description[200];
struct Plant* next;
};
struct Plant* create_plant(char name[], char family[], char genus[], char species[], char description[]) {
struct Plant* new_plant = (struct Plant*)malloc(sizeof(struct Plant));
strcpy(new_plant->name, name);
strcpy(new_plant->family, family);
strcpy(new_plant->genus, genus);
strcpy(new_plant->species, species);
strcpy(new_plant->description, description);
new_plant->next = NULL;
return new_plant;
}
void add_plant(struct Plant** head, char name[], char family[], char genus[], char species[], char description[]) {
struct Plant* new_plant = create_plant(name, family, genus, species, description);
new_plant->next = *head;
*head = new_plant;
}
void print_plant(struct Plant* plant) {
printf("Plant Name: %s\n", plant->name);
printf("Family: %s\n", plant->family);
printf("Genus: %s\n", plant->genus);
printf("Species: %s\n", plant->species);
printf("Description: %s\n", plant->description);
printf("--------------------\n");
}
void print_list(struct Plant* head) {
struct Plant* current_plant = head;
while (current_plant) {
print_plant(current_plant);
current_plant = current_plant->next;
}
}
int main() {
struct Plant* plant_list = NULL;
FILE* fp = fopen("data_edit/plant.txt", "r");
if (fp == NULL) {
printf("Failed to open file\n");
return 0;
}
char line[300], name[50], family[50], genus[50], species[50], description[200];
while (fgets(line, 300, fp)) {
sscanf(line, "%[^,],%[^,],%[^,],%[^,],%[^\n]", name, family, genus, species, description);
add_plant(&plant_list, name, family, genus, species, description);
}
fclose(fp);
// 添加新植物信息
add_plant(&plant_list, "Lavender", "Lamiaceae", "Lavandula", "angustifolia", "A fragrant herb used in perfumes and soaps.");
// 打印植物信息链表
print_list(plant_list);
return 0;
}
```
这段代码会读取 `data_edit/plant.txt` 文件,将其中的植物信息读入一个链表中,然后使用 `add_plant()` 函数向链表中添加新的植物信息,最后使用 `print_list()` 函数打印整个植物信息链表。请注意,为了使代码更加清晰易懂,我做了一些简化,你需要根据实际情况进行修改。
相关推荐
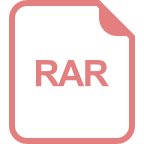
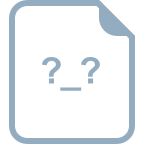















