讲下这段程序的编程流程和目的#include "main.h" //=========================================== sbit RS=P1^0; sbit RW=P1^1; sbit E=P1^2; sbit lcd_bla = P2^7; //=========================================== void Delay140us() //@12.000MHz 1T { unsigned char i, j; nop(); nop(); i = 2; j = 158; do { while (--j); } while (--i); } //void lcd_delay(uchar z) //{ // uchar t1,y; // for(t1=z;t1>0;t1--) // for(y=110;y>0;y--); //} //void Delay140us() //@12.000MHz 12T //{ // unsigned char i; // // nop(); // i = 67; // while (--i); //} // //uchar lcd_busy() //{ // register uchar lcd_start; // RS=0; // RW=1; // E=1; // lcd_delay(1); // lcd_start=P0; // E=0; // return(lcd_start&0x80); //} //============================================ void read(u8 del) //读函数 { P0=del; // while(lcd_busy()); RS=0; RW=0; E=0; Delay140us(); E=1; Delay140us(); } //============================================ void write(u8 del) //写函数 { P0=del; // while(lcd_busy()); RS=1; RW=0; E=0; Delay140us(); E=1; Delay140us(); } //============================================ //============================================ void lcd_init(void) //初始化函数 { lcd_bla = 0; read(0x01); read(0x0c); read(0x06); read(0xd0); read(0x38); lcd_show(1,1,' '); } //============================================ //void lcd_clear(void) //{ //read(0x01); //Delay140us(); //} void lcd_show(u8 hang,u8 lie,int sign) //数字显示 { u8 a; if(hang==1)a=0x80; if(hang==2) a=0xc0; a=a+lie-1; read(a); write(sign+48); } //============================================ void lcd_string(u8 hang,u8 lie,u8 *p) //字符串显示 { u8 a; if(hang==1)a=0x80; if(hang==2) a=0xc0; a=a+lie-1; read(a); while(1) { if(*p == '\0') break; write(*p); p++; } }
时间: 2023-08-23 18:07:55 浏览: 43
这段程序的编程流程和目的和上一段程序是一样的,都是控制LCD显示器,实现数字和字符串的显示。它们的区别在于一些细节上,比如其中的延时函数Delay140us()使用的是1T的延时方式,而上一段程序中的Delay140us()使用的是12T的延时方式。此外,lcd_init()函数中的lcd_show()函数的参数不同,上一段程序中是' ',而这一段程序中是0x30,这是因为这里将数字0的ASCII码值加上了48,即0x30,实现了数字的显示。
相关问题
HANDLE hout =……
这是一个 Windows API 中的句柄(handle),具体来说,是一个指向控制台输出缓冲区的指针。在 C 或 C++ 编程中,hout 变量的类型通常是 HANDLE,可以使用它来向控制台输出信息。例如,可以使用以下代码向控制台输出一条消息:
```
#include <Windows.h>
#include <iostream>
int main()
{
HANDLE hout = GetStdHandle(STD_OUTPUT_HANDLE);
WriteConsole(hout, L"Hello, World!", 13, NULL, NULL);
return 0;
}
```
这段代码中,GetStdHandle 函数获取了标准输出设备的句柄,然后使用 WriteConsole 函数向控制台输出了一条消息。
#include "led.h" #include "delay.h" #include "key.h" #include "sys.h" #include "24cxx.h" #include "OLED4PINiic.h"#include "usart.h" #include "time.h" #include "74HC595_led.h"在这些头文件的基础上,编程实现控制STM32F103XC8T6实验板上LED的开关和频闪的快慢,实验板上有4个按键,从按键1至4被按下,LED闪烁频率实现由低到高的转变,请给出具体实现代码
以下是实现代码:
```c
#include "led.h"
#include "delay.h"
#include "key.h"
#include "sys.h"
#include "24cxx.h"
#include "OLED4PINiic.h"
#include "usart.h"
#include "time.h"
#include "74HC595_led.h"
int main(void)
{
u8 led_status = 0; // LED 状态,0 表示关,1 表示开
u8 key_value = 0; // 按键值
u8 freq_level = 0; // LED 闪烁频率等级,0 表示最低频率,3 表示最高频率
u16 freq_delay[4] = {1000, 500, 250, 125}; // 不同频率对应的延时时间
u8 freq_index = 0; // 当前闪烁频率对应的延时时间数组下标
LED_Init(); // 初始化 LED
KEY_Init(); // 初始化按键
while (1)
{
key_value = KEY_Scan(0); // 扫描按键,返回按键值
switch (key_value)
{
case KEY1_PRES:
freq_index = 0; // 切换到最低频率
break;
case KEY2_PRES:
freq_index = 1; // 切换到中低频率
break;
case KEY3_PRES:
freq_index = 2; // 切换到中高频率
break;
case KEY4_PRES:
freq_index = 3; // 切换到最高频率
break;
default:
break;
}
// 根据当前频率等级设置延时时间
delay_ms(freq_delay[freq_index]);
// 切换 LED 状态并控制 LED 闪烁频率
if (led_status == 0)
{
LED0 = 1; // 打开 LED
delay_ms(freq_delay[freq_index]); // 延时一段时间
LED0 = 0; // 关闭 LED
delay_ms(freq_delay[freq_index]); // 延时一段时间
}
else
{
LED0 = 1; // 打开 LED
delay_ms(freq_delay[freq_index] * 2); // 延时一段时间
LED0 = 0; // 关闭 LED
delay_ms(freq_delay[freq_index] * 2); // 延时一段时间
}
// 切换 LED 状态
led_status = !led_status;
}
}
```
这段代码使用 `KEY_Scan()` 函数扫描按键,根据按键值切换 LED 闪烁频率等级,然后根据当前频率等级控制 LED 的闪烁频率和开关状态。其中,闪烁频率通过延时时间实现,LED 闪烁的快慢由延时时间长短决定。在这里,我们使用一个数组 `freq_delay` 存储不同频率对应的延时时间,然后根据当前频率等级 `freq_index` 选择相应的延时时间。闪烁频率越高,延时时间越短,LED 闪烁的频率越快。同时,LED 的开关状态也随着闪烁频率的改变而变化。如果 LED 处于关闭状态,那么每次开启 LED 的时间为一个延时时间,然后关闭 LED 并再次延时一个延时时间;如果 LED 处于开启状态,那么每次开启 LED 的时间为两个延时时间,然后关闭 LED 并再次延时两个延时时间。
相关推荐
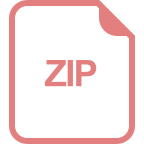
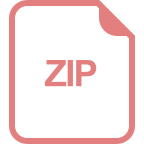
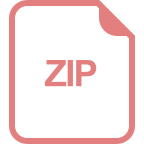












