ffmpeg 使用多线程处理,例如将解码和后处理操作分开处理,提高处理效率,c++
时间: 2023-12-31 09:03:20 浏览: 17
是的,FFmpeg支持多线程处理,可以将解码和后处理操作分开处理,从而提高处理效率。以下是一个示例代码:
```c++
#include <iostream>
#include <thread>
#include "ffmpeg.h"
using namespace std;
// 解码线程
void decode_thread(AVCodecContext* codec_ctx, AVPacket* pkt, AVFrame* frame)
{
int ret = avcodec_send_packet(codec_ctx, pkt);
if (ret < 0) {
cerr << "Error sending packet to decoder: " << av_err2str(ret) << endl;
return;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
// 没有更多的输出帧
return;
} else if (ret < 0) {
cerr << "Error during decoding: " << av_err2str(ret) << endl;
return;
}
// 帧处理操作可以在此处添加
av_frame_unref(frame);
}
}
int main(int argc, char* argv[])
{
// 初始化FFmpeg
av_register_all();
avcodec_register_all();
// 打开输入文件
AVFormatContext* fmt_ctx = nullptr;
int ret = avformat_open_input(&fmt_ctx, argv[1], nullptr, nullptr);
if (ret < 0) {
cerr << "Error opening input file: " << av_err2str(ret) << endl;
return -1;
}
// 检索流信息
ret = avformat_find_stream_info(fmt_ctx, nullptr);
if (ret < 0) {
cerr << "Error finding stream information: " << av_err2str(ret) << endl;
avformat_close_input(&fmt_ctx);
return -1;
}
// 查找视频流
int video_stream_idx = av_find_best_stream(fmt_ctx, AVMEDIA_TYPE_VIDEO, -1, -1, nullptr, 0);
if (video_stream_idx < 0) {
cerr << "Error finding video stream: " << av_err2str(video_stream_idx) << endl;
avformat_close_input(&fmt_ctx);
return -1;
}
// 获取视频解码器
AVCodec* codec = avcodec_find_decoder(fmt_ctx->streams[video_stream_idx]->codecpar->codec_id);
if (!codec) {
cerr << "Error finding video codec" << endl;
avformat_close_input(&fmt_ctx);
return -1;
}
// 创建解码器上下文
AVCodecContext* codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
cerr << "Error allocating codec context" << endl;
avformat_close_input(&fmt_ctx);
return -1;
}
// 初始化解码器上下文
ret = avcodec_parameters_to_context(codec_ctx, fmt_ctx->streams[video_stream_idx]->codecpar);
if (ret < 0) {
cerr << "Error initializing codec context: " << av_err2str(ret) << endl;
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return -1;
}
// 打开解码器
ret = avcodec_open2(codec_ctx, codec, nullptr);
if (ret < 0) {
cerr << "Error opening codec: " << av_err2str(ret) << endl;
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return -1;
}
// 读取帧并进行解码
AVPacket pkt;
av_init_packet(&pkt);
AVFrame* frame = av_frame_alloc();
while (av_read_frame(fmt_ctx, &pkt) >= 0) {
if (pkt.stream_index == video_stream_idx) {
// 创建解码线程
thread t(decode_thread, codec_ctx, &pkt, frame);
// 等待线程完成
t.join();
}
av_packet_unref(&pkt);
}
// 释放资源
av_frame_free(&frame);
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return 0;
}
```
在上述示例代码中,我们使用一个单独的线程来处理解码操作,这样可以让主线程继续读取下一个数据包,提高了处理效率。你可以根据你的具体需求,将其他处理操作也放在不同的线程中。
相关推荐
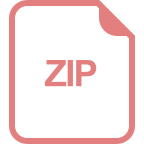
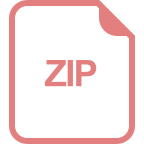














