ffmpeg c++多线程 rgb转h264
时间: 2023-10-05 10:11:07 浏览: 316
你可以使用 FFmpeg 的 C++ 接口来实现 RGB 转 H.264 的多线程编码。下面是一个简单的示例代码,它将一系列 RGB 图像转换为 H.264 视频:
```cpp
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <vector>
#include <sstream>
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
}
using namespace std;
// 定义互斥量和条件变量,用于多线程同步
mutex mtx;
condition_variable cv;
// 定义全局变量,用于存储编码后的视频数据
vector<uint8_t> video_buffer;
// 定义编码器上下文和帧对象
AVCodecContext *encoder_ctx = NULL;
AVFrame *frame = NULL;
// 定义编码器线程
void encode_thread()
{
AVPacket pkt;
int ret;
while (true) {
unique_lock<mutex> lock(mtx);
cv.wait(lock, [] { return !video_buffer.empty(); });
// 从全局变量中取出一帧 RGB 图像
vector<uint8_t> rgb_image = video_buffer.back();
video_buffer.pop_back();
// 把 RGB 图像转换为编码器所需的格式
av_image_fill_arrays(frame->data, frame->linesize, rgb_image.data(),
encoder_ctx->pix_fmt, encoder_ctx->width, encoder_ctx->height, 1);
// 发送图像数据到编码器
ret = avcodec_send_frame(encoder_ctx, frame);
if (ret < 0) {
cerr << "Error sending a frame to the encoder: " << av_err2str(ret) << endl;
continue;
}
// 接收编码后的数据包
while (ret >= 0) {
ret = avcodec_receive_packet(encoder_ctx, &pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
cerr << "Error encoding a frame: " << av_err2str(ret) << endl;
continue;
}
// 把编码后的数据包存储到全局变量中
video_buffer.insert(video_buffer.begin(), pkt.data, pkt.data + pkt.size);
av_packet_unref(&pkt);
}
}
}
int main()
{
// 初始化 FFmpeg 库
av_register_all();
avformat_network_init();
// 打开编码器并设置编码参数
AVCodec *codec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (!codec) {
cerr << "Codec not found" << endl;
return -1;
}
encoder_ctx = avcodec_alloc_context3(codec);
if (!encoder_ctx) {
cerr << "Could not allocate video codec context" << endl;
return -1;
}
encoder_ctx->bit_rate = 400000;
encoder_ctx->width = 640;
encoder_ctx->height = 480;
encoder_ctx->time_base = {1, 25};
encoder_ctx->gop_size = 10;
encoder_ctx->max_b_frames = 1;
encoder_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
if (avcodec_open2(encoder_ctx, codec, NULL) < 0) {
cerr << "Could not open codec" << endl;
return -1;
}
// 创建帧对象并分配内存
frame = av_frame_alloc();
if (!frame) {
cerr << "Could not allocate video frame" << endl;
return -1;
}
frame->format = encoder_ctx->pix_fmt;
frame->width = encoder_ctx->width;
frame->height = encoder_ctx->height;
if (av_frame_get_buffer(frame, 0) < 0) {
cerr << "Could not allocate the video frame data" << endl;
return -1;
}
// 创建编码器线程
thread t(encode_thread);
// 读入 RGB 图像并发送到编码器线程进行编码
for (int i = 1; i <= 100; i++) {
stringstream ss;
ss << "rgb" << i << ".raw";
FILE *f = fopen(ss.str().c_str(), "rb");
if (!f) {
cerr << "Could not open file: " << ss.str() << endl;
return -1;
}
int size = encoder_ctx->width * encoder_ctx->height * 3;
vector<uint8_t> rgb_image(size);
fread(rgb_image.data(), 1, size, f);
fclose(f);
{
lock_guard<mutex> lock(mtx);
video_buffer.push_back(rgb_image);
}
cv.notify_one();
}
// 等待编码器线程退出
t.join();
// 释放资源
avcodec_free_context(&encoder_ctx);
av_frame_free(&frame);
return 0;
}
```
在这个示例代码中,我们使用了一个互斥量和一个条件变量来实现多线程同步。编码器线程等待条件变量的通知,然后从全局变量中取出一帧 RGB 图像,把它转换为编码器所需的格式,并发送到编码器进行编码。编码器线程把编码后的数据包存储到全局变量中,然后再次等待条件变量的通知。主线程读入一系列 RGB 图像,并把它们存储到全局变量中,然后通过条件变量通知编码器线程进行编码。最后,主线程等待编码器线程退出,并释放资源。
注意,这个示例代码中只使用了一个编码器线程。如果你想进一步提高编码速度,可以使用多个编码器线程,并且把 RGB 图像分割成多块,让每个编码器线程处理一块图像。你也可以使用 OpenMP 或其他线程库来实现多线程编码。
阅读全文
相关推荐
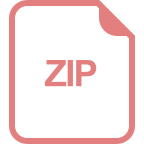
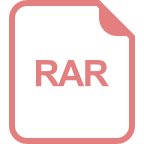
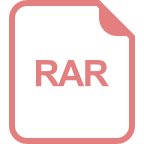
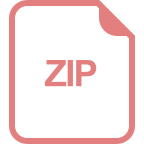
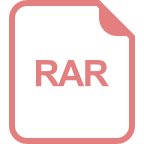
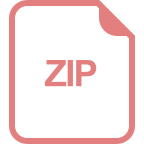
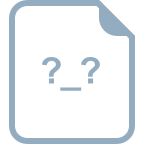
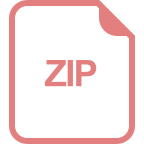
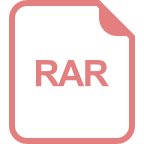
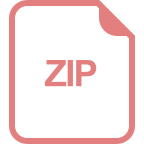
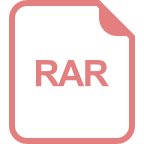
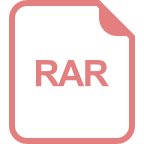
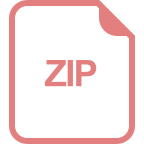
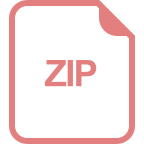
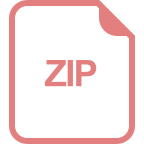
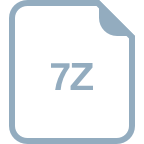