C++用数组汉诺塔显示横向纵向显示移动过程
时间: 2024-05-05 07:22:09 浏览: 124
以下是使用数组实现汉诺塔移动过程的C++代码,包括横向和纵向显示移动过程:
```c++
#include <iostream>
using namespace std;
const int MAXN = 100;
int n; // 汉诺塔的层数
char a[MAXN], b[MAXN], c[MAXN]; // a,b,c三个塔
int cnt = 0; // 移动次数
// 将第n个盘子从a柱移动到c柱
void move(char a[], char b[], char c[], int n) {
if (n == 1) {
// 只有一个盘子,直接移动
cnt++;
c[++c[0]] = a[a[0]--];
// 横向显示
cout << "Move " << cnt << ": ";
for (int i = 1; i <= n; i++) {
cout << a[i] << " ";
}
cout << "-> ";
for (int i = 1; i <= n; i++) {
cout << c[i] << " ";
}
cout << endl;
// 纵向显示
for (int i = n; i >= 1; i--) {
for (int j = 1; j <= 3; j++) {
if (a[i] == 'A' && j == 1) {
cout << " " << a[i] << " ";
} else if (b[i] == 'B' && j == 2) {
cout << " " << b[i] << " ";
} else if (c[i] == 'C' && j == 3) {
cout << " " << c[i] << " ";
} else {
cout << " ";
}
}
cout << endl;
}
cout << endl;
} else {
// 先将n-1个盘子从a柱移动到b柱
move(a, c, b, n - 1);
// 再将第n个盘子从a柱移动到c柱
move(a, b, c, 1);
// 最后将n-1个盘子从b柱移动到c柱
move(b, a, c, n - 1);
}
}
int main() {
// 输入汉诺塔层数
cout << "Please input the number of disks:";
cin >> n;
// 初始化汉诺塔
a[0] = n;
for (int i = 1; i <= n; i++) {
a[i] = 'A';
b[i] = 'B';
c[i] = 'C';
}
// 显示初始状态
cout << "Initial State:" << endl;
for (int i = n; i >= 1; i--) {
for (int j = 1; j <= 3; j++) {
if (a[i] == 'A' && j == 1) {
cout << " " << a[i] << " ";
} else if (b[i] == 'B' && j == 2) {
cout << " " << b[i] << " ";
} else if (c[i] == 'C' && j == 3) {
cout << " " << c[i] << " ";
} else {
cout << " ";
}
}
cout << endl;
}
cout << endl;
// 开始移动汉诺塔
move(a, b, c, n);
// 显示移动次数
cout << "Total moves: " << cnt << endl;
return 0;
}
```
在横向显示移动过程时,每次移动一个盘子后,都会显示当前三个塔的状态。
在纵向显示移动过程时,每次移动一个盘子后,都会显示当前三个塔的状态,其中用空格表示该位置没有盘子,用字母A、B、C表示该位置有盘子,字母的大小表示盘子的大小。
相关推荐
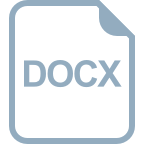
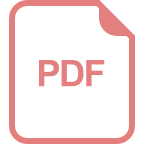
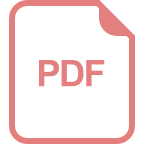














