c++前序遍历汉诺塔递归算法
时间: 2023-11-18 17:53:26 浏览: 36
好的,以下是 C++ 前序遍历汉诺塔递归算法的代码:
```c++
#include <iostream>
using namespace std;
void hanoi(int n, char A, char B, char C) {
if (n == 1) {
cout << A << "->" << C << endl;
return;
}
hanoi(n - 1, A, C, B);
cout << A << "->" << C << endl;
hanoi(n - 1, B, A, C);
}
int main() {
int n;
cout << "请输入汉诺塔的层数:";
cin >> n;
hanoi(n, 'A', 'B', 'C');
return 0;
}
```
相关问题
汉诺塔递归算法c++
汉诺塔问题是一个经典的递归问题,它可以用来展示递归算法的思想和应用。汉诺塔问题的规则如下:有三根柱子,标记为A、B、C,其中A柱子上有n个盘子,盘子的大小不同,大的在下面,小的在上面。现在要将A柱子上的盘子移动到C柱子上,移动过程中可以借助B柱子。移动的规则是每次只能移动一个盘子,并且大盘子不能放在小盘子上面。
以下是汉诺塔递归算法的C++实现:
```cpp
#include <iostream>
using namespace std;
void hanoi(int n, char source, char target, char auxiliary) {
if (n == 1) {
cout << "Move disk 1 from " << source << " to " << target << endl;
return;
}
hanoi(n - 1, source, auxiliary, target);
cout << "Move disk " << n << " from " << source << " to " << target << endl;
hanoi(n - 1, auxiliary, target, source);
}
int main() {
int n;
cout << "Enter the number of disks: ";
cin >> n;
hanoi(n, 'A', 'C', 'B');
return 0;
}
```
在这段代码中,`hanoi`函数是递归函数,它接受四个参数:n表示盘子的数量,source表示源柱子,target表示目标柱子,auxiliary表示辅助柱子。当n等于1时,直接将盘子从源柱子移动到目标柱子;否则,先将n-1个盘子从源柱子移动到辅助柱子,然后将第n个盘子从源柱子移动到目标柱子,最后将n-1个盘子从辅助柱子移动到目标柱子。
希望以上代码和解释对你有帮助!
汉诺塔递归算法python
汉诺塔是一个经典的递归算法问题,其思路是将n个盘子从起始柱子通过中间柱子移动到目标柱子,保证大盘子在下面,小盘子在上面。在移动过程中,不能将大盘子放在小盘子上面。下面是汉诺塔递归算法的Python实现:
```python
def hanoi(n, start, end, mid):
if n == 1:
print(start, '->', end)
else:
hanoi(n-1, start, mid, end)
print(start, '->', end)
hanoi(n-1, mid, end, start)
```
其中,n表示盘子的数量,start表示起始柱子,end表示目标柱子,mid表示中间柱子。当n等于1时,直接将起始柱子上的盘子移动到目标柱子上;当n大于1时,先将n-1个盘子从起始柱子移动到中间柱子上,再将最后一个盘子从起始柱子移动到目标柱子上,最后将n-1个盘子从中间柱子移动到目标柱子上。
相关推荐
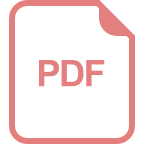
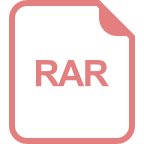
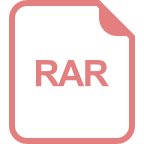
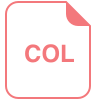
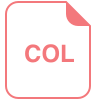
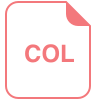
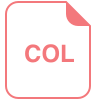
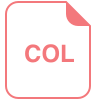







