python的拼图游戏
时间: 2023-06-22 09:44:30 浏览: 59
好的,Python的拼图游戏可以使用Pygame这个游戏开发库来实现。下面是一个简单的例子:
首先,需要导入Pygame库和其他必要的库:
```python
import pygame
import random
import os
```
然后,我们需要定义一些常量,比如游戏窗口大小、拼图块的大小等:
```python
WIDTH = 480
HEIGHT = 480
TILE_SIZE = 80
TILES_PER_ROW = WIDTH // TILE_SIZE
TILES_PER_COL = HEIGHT // TILE_SIZE
```
接下来,我们需要加载拼图图片并将其切分成拼图块:
```python
def load_image(file_name):
file_path = os.path.join('images', file_name)
return pygame.image.load(file_path)
def create_tiles(image):
tiles = []
for i in range(TILES_PER_ROW):
for j in range(TILES_PER_COL):
rect = pygame.Rect(i*TILE_SIZE, j*TILE_SIZE, TILE_SIZE, TILE_SIZE)
tile = image.subsurface(rect)
tiles.append(tile)
return tiles
image = load_image('puzzle.jpg')
tiles = create_tiles(image)
```
然后,我们需要定义一些函数来处理拼图块的移动和交换:
```python
def get_tile_index(pos):
x, y = pos
row = y // TILE_SIZE
col = x // TILE_SIZE
return row * TILES_PER_ROW + col
def get_tile_pos(index):
row = index // TILES_PER_ROW
col = index % TILES_PER_ROW
x = col * TILE_SIZE
y = row * TILE_SIZE
return (x, y)
def move_tile(index1, index2):
tiles[index1], tiles[index2] = tiles[index2], tiles[index1]
def swap_tiles(index1, index2):
move_tile(index1, index2)
```
接着,我们需要定义一些事件处理函数,比如处理鼠标点击事件:
```python
def handle_mouse_click(pos):
index = get_tile_index(pos)
blank_index = get_tile_index(get_tile_pos(len(tiles)-1))
if index == blank_index:
return
if index % TILES_PER_ROW > 0 and index - 1 == blank_index:
swap_tiles(index, blank_index)
elif index % TILES_PER_ROW < TILES_PER_ROW - 1 and index + 1 == blank_index:
swap_tiles(index, blank_index)
elif index - TILES_PER_ROW == blank_index:
swap_tiles(index, blank_index)
elif index + TILES_PER_ROW == blank_index:
swap_tiles(index, blank_index)
```
最后,我们需要定义主循环和游戏结束的处理:
```python
def main():
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('Puzzle')
clock = pygame.time.Clock()
game_over = False
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
handle_mouse_click(event.pos)
screen.fill((255, 255, 255))
for i, tile in enumerate(tiles):
pos = get_tile_pos(i)
screen.blit(tile, pos)
pygame.display.flip()
clock.tick(60)
pygame.quit()
if __name__ == '__main__':
main()
```
这个例子中,我们使用随机交换拼图块的位置来生成一个随机的拼图游戏。如果你想要更加复杂的拼图游戏,可以使用A*算法等来实现自动解谜功能。
相关推荐
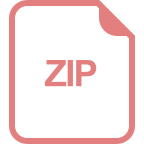













