用C语言查找链表最后一个节点 struct node {int ch; 结构节点*下一个;}; 定义函数遍历一个不带头节点的链表,返回链表上最后一个节点的数据成员的值。
时间: 2024-04-13 19:29:59 浏览: 19
你可以使用以下的C语言代码来遍历不带头节点的链表,并返回最后一个节点的数据成员的值:
```c
#include<stdio.h>
#include<stdlib.h>
struct node {
int data;
struct node *next;
};
int getLastNodeValue(struct node *head) {
// 检查链表是否为空
if (head == NULL) {
printf("链表为空\n");
return -1;
}
struct node *current = head;
// 遍历链表直到最后一个节点
while (current->next != NULL) {
current = current->next;
}
// 返回最后一个节点的数据成员的值
return current->data;
}
int main() {
// 创建链表
struct node *head = NULL;
struct node *second = NULL;
struct node *third = NULL;
head = (struct node *)malloc(sizeof(struct node));
second = (struct node *)malloc(sizeof(struct node));
third = (struct node *)malloc(sizeof(struct node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
// 调用函数并打印最后一个节点的值
int lastNodeValue = getLastNodeValue(head);
printf("最后一个节点的值: %d\n", lastNodeValue);
// 释放内存
free(head);
free(second);
free(third);
return 0;
}
```
这段代码中,我们首先定义了一个`node`结构体,包含一个整型数据成员`data`和一个指向下一个节点的指针`next`。然后,我们通过定义`getLastNodeValue`函数来遍历链表并返回最后一个节点的值。
在`getLastNodeValue`函数中,我们首先检查链表是否为空。然后,我们使用一个指针`current`来追踪当前节点,从头节点开始。我们通过遍历链表,不断将`current`指针指向下一个节点,直到最后一个节点。最后,我们返回最后一个节点的数据成员的值。
在`main`函数中,我们创建了一个简单的链表,并调用`getLastNodeValue`函数来获取最后一个节点的值,并打印出来。最后,我们释放了动态分配的内存。
请注意,这段代码假设链表至少有一个节点。如果链表为空,则会打印出相应的提示信息并返回-1作为错误值。
相关推荐
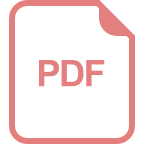












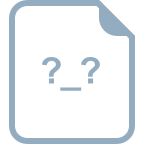
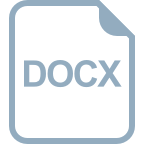