用pycharm编写python语言时候,file_path的文件路径怎么写相对路径和绝对路径,请举例说明
时间: 2024-02-05 16:11:05 浏览: 101
在Pycharm中,可以使用相对路径或绝对路径来指定文件路径。下面分别举例说明:
### 相对路径
相对路径是相对于当前工作目录的路径,可以使用以下符号:
- `.` 代表当前目录
- `..` 代表上一级目录
假设我们有如下目录结构:
```
project/
|-- main.py
|-- data/
|-- input.txt
```
如果我们在 `main.py` 中需要读取 `input.txt` 文件,可以使用相对路径 `./data/input.txt`,其中 `.` 代表当前目录,`data/input.txt` 代表 `input.txt` 文件相对于当前目录的路径。
```python
file_path = "./data/input.txt"
```
### 绝对路径
绝对路径是从根目录开始的完整路径,可以使用操作系统提供的文件路径格式。假设我们的工程目录在 `C:\Users\username\project` 下,我们可以使用如下绝对路径来指定 `input.txt` 文件:
```python
file_path = "C:/Users/username/project/data/input.txt"
```
需要注意的是,在Windows系统中,要使用正斜杠 `/` 来表示路径分隔符,而不是反斜杠 `\`。
使用相对路径还是绝对路径取决于具体情况,一般来说,如果需要在多个不同的工程中使用同一个文件,建议使用绝对路径。如果只在当前工程中使用该文件,可以使用相对路径。
相关问题
import fitz # PyMuPDF库 import os # 读取PDF文件的内容 def read_pdf(file_path): doc = fitz.open(file_path) content = "" for page in doc: content += page.getText("text") doc.close() return content # 去除字符串中的空格和空行 def remove_spaces(text): return "\n".join([line.strip() for line in text.split("\n") if line.strip()]) # 将字符串按中文句号分割成多个语句 def split_sentences(text): sentences = [] for sentence in text.split("。"): sentence = sentence.strip() if sentence: sentences.append(sentence + "。") return sentences # 读取指定目录下所有PDF文件的内容并合并成一个字符串 def read_all_pdfs(dir_path): all_content = "" for file_name in os.listdir(dir_path): if file_name.endswith(".pdf"): file_path = os.path.join(dir_path, file_name) content = read_pdf(file_path) content = remove_spaces(content) all_content += content return all_content # 将字符串按中文句号分割成多个语句并打印出来 def print_sentences(text): sentences = split_sentences(text) for sentence in sentences: print(sentence) # 测试 dir_path = r"D:\点宽学院" all_content = read_all_pdfs(dir_path) print_sentences(all_content)这是我的代码显示Traceback (most recent call last): File "D:\python+pycharm\ceshi.py", line 44, in <module> all_content = read_all_pdfs(dir_path) File "D:\python+pycharm\ceshi.py", line 31, in read_all_pdfs content = read_pdf(file_path) File "D:\python+pycharm\ceshi.py", line 8, in read_pdf content += page.getText("text") AttributeError: 'Page' object has no attribute 'getText',如何修改呢
这个错误提示说明 `Page` 对象没有 `getText` 方法。可能是因为你使用的 PyMuPDF 版本不同于示例代码中使用的版本。建议尝试以下两种方法:
1. 使用 `extract_text()` 方法代替 `getText()` 方法,例如:
```python
content += page.extract_text()
```
2. 使用 PyMuPDF 最新版本(1.18.8),因为该版本支持 `getText()` 方法。可以通过以下命令安装最新版本:
```python
pip install PyMuPDF==1.18.8
```
希望能够帮到你!
import json import os #指定原始数据集和目标路径 json_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/ce_shi_label" txt_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/labels" #获取所有json文件的路径 json_files = [os.path.join(json_folder_path, f) for f in os.listdir(json_folder_path) if f.endswith('.json')] for json_file in json_files:#打开json文件 with open(json_file, 'r', encoding='utf-8') as f:data = json.load(f) image_name = data["img_name"] + ".jpg" label_name = data["img_name"] boxes = data["ann"] #将每个矩形框转换为txt格式 txt_data = "" txt_line_1 = f"{label_name}\n" for box in boxes: x1, y1,x2, y2,class_id = str(box[0]), str(box[1]), str(box[2]),str(box[3]),str(box[4]) txt_line =f"{x1} {y1} {x2} {y2} {class_id}\n" txt_data += txt_line #写入txt文件 txt_file = os.path.join(txt_folder_path, image_name.replace(".jpg", ".txt")) with open(txt_file, 'w', encoding='utf-8') as f:f.write(txt_line_1 + txt_data)将其中json文件的名称统一放在一一个新的txt文件中
可以在循环外部先创建一个空的列表,然后在循环中每次将json文件的名称添加到列表中。循环结束后再将列表中的元素写入到一个新的txt文件中即可。
示例代码如下:
```python
import json
import os
# 指定原始数据集和目标路径
json_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/ce_shi_label"
txt_folder_path = "D:/python/pycharm/pycharm_date/Oracle/OracleCS/labels"
# 获取所有json文件的路径
json_files = [os.path.join(json_folder_path, f) for f in os.listdir(json_folder_path) if f.endswith('.json')]
# 创建空的列表
json_names = []
for json_file in json_files:
# 打开json文件
with open(json_file, 'r', encoding='utf-8') as f:
data = json.load(f)
image_name = data["img_name"] + ".jpg"
label_name = data["img_name"]
boxes = data["ann"]
# 将json文件名称添加到列表中
json_names.append(label_name)
# 将每个矩形框转换为txt格式
txt_data = ""
txt_line_1 = f"{label_name}\n"
for box in boxes:
x1, y1,x2, y2,class_id = str(box[0]), str(box[1]), str(box[2]),str(box[3]),str(box[4])
txt_line =f"{x1} {y1} {x2} {y2} {class_id}\n"
txt_data += txt_line
# 写入txt文件
txt_file = os.path.join(txt_folder_path, image_name.replace(".jpg", ".txt"))
with open(txt_file, 'w', encoding='utf-8') as f:
f.write(txt_line_1 + txt_data)
# 将json文件名称写入新的txt文件中
json_names_file = os.path.join(txt_folder_path, "json_names.txt")
with open(json_names_file, 'w', encoding='utf-8') as f:
for name in json_names:
f.write(name + "\n")
```
这样,就可以将所有json文件的名称统一放在一个新的txt文件中了。
阅读全文
相关推荐
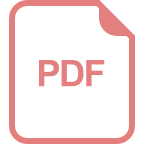
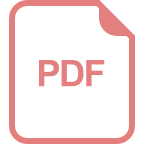
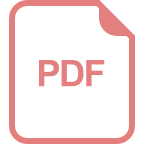
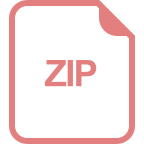
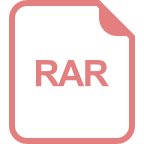
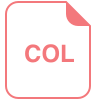
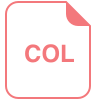









