c++编俄罗斯方块代码
时间: 2024-04-21 14:20:14 浏览: 19
C++编写俄罗斯方块的代码可以分为以下几个部分:
1. 方块的表示:可以使用二维数组来表示方块的形状,每个方块可以由四个小方块组成。例如,可以使用一个4x4的二维数组来表示方块的形状,其中1表示方块的一部分,0表示空白。
2. 方块的移动:可以使用键盘输入来控制方块的移动,例如按下左箭头键可以使方块向左移动,按下右箭头键可以使方块向右移动,按下下箭头键可以使方块加速下落。
3. 方块的旋转:可以使用旋转矩阵来实现方块的旋转。通过改变方块的形状数组,可以实现方块的顺时针或逆时针旋转。
4. 方块的碰撞检测:在方块下落的过程中,需要检测方块是否与其他已经存在的方块或边界发生碰撞。可以通过判断方块下方是否有其他方块或边界来进行碰撞检测。
5. 方块的消除:当一行被填满时,需要将该行消除,并将上面的方块向下移动一行。可以通过遍历每一行,检查是否满行来实现方块的消除。
以上是俄罗斯方块的基本代码结构,具体的实现方式可以根据个人的编程风格和需求进行调整和扩展。
相关问题
用c++编写俄罗斯方块代码
好的,我可以回答这个问题。以下是一个简单的俄罗斯方块代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 10
#define HEIGHT 20
int board[HEIGHT][WIDTH] = {};
int shapes[7][4][2] = {
{{, }, {, 1}, {1, }, {1, 1}}, // O
{{, }, {, 1}, {, 2}, {, 3}}, // I
{{, }, {, 1}, {, 2}, {1, 2}}, // L
{{, }, {, 1}, {, 2}, {-1, 2}}, // J
{{, }, {, 1}, {1, 1}, {1, 2}}, // Z
{{, }, {, 1}, {-1, 1}, {-1, 2}}, // S
{{, }, {, 1}, {, 2}, {1, 1}} // T
};
int cur_shape[4][2];
int cur_x, cur_y, cur_type;
int score = ;
void init_game()
{
srand(time(NULL));
cur_type = rand() % 7;
for (int i = ; i < 4; i++) {
cur_shape[i][] = shapes[cur_type][i][];
cur_shape[i][1] = shapes[cur_type][i][1];
}
cur_x = WIDTH / 2 - 1;
cur_y = ;
}
void draw_board()
{
system("cls");
for (int i = ; i < HEIGHT; i++) {
for (int j = ; j < WIDTH; j++) {
if (board[i][j] == ) {
printf(".");
} else {
printf("*");
}
}
printf("\n");
}
printf("Score: %d\n", score);
}
int check_collision(int x, int y, int type)
{
for (int i = ; i < 4; i++) {
int nx = x + cur_shape[i][];
int ny = y + cur_shape[i][1];
if (nx < || nx >= WIDTH || ny < || ny >= HEIGHT) {
return 1;
}
if (board[ny][nx] != ) {
return 1;
}
}
return ;
}
void place_shape()
{
for (int i = ; i < 4; i++) {
int nx = cur_x + cur_shape[i][];
int ny = cur_y + cur_shape[i][1];
board[ny][nx] = cur_type + 1;
}
}
void remove_lines()
{
int lines = ;
for (int i = HEIGHT - 1; i >= ; i--) {
int full = 1;
for (int j = ; j < WIDTH; j++) {
if (board[i][j] == ) {
full = ;
break;
}
}
if (full) {
lines++;
for (int k = i; k > ; k--) {
for (int j = ; j < WIDTH; j++) {
board[k][j] = board[k - 1][j];
}
}
for (int j = ; j < WIDTH; j++) {
board[][j] = ;
}
i++;
}
}
score += lines * lines * 100;
}
void game_loop()
{
while (1) {
draw_board();
if (check_collision(cur_x, cur_y + 1, cur_type)) {
place_shape();
remove_lines();
init_game();
if (check_collision(cur_x, cur_y, cur_type)) {
break;
}
} else {
cur_y++;
}
if (_kbhit()) {
int c = _getch();
if (c == 'a' && !check_collision(cur_x - 1, cur_y, cur_type)) {
cur_x--;
} else if (c == 'd' && !check_collision(cur_x + 1, cur_y, cur_type)) {
cur_x++;
} else if (c == 's' && !check_collision(cur_x, cur_y + 1, cur_type)) {
cur_y++;
} else if (c == 'w') {
int new_type = (cur_type + 1) % 7;
int new_shape[4][2];
for (int i = ; i < 4; i++) {
new_shape[i][] = shapes[new_type][i][];
new_shape[i][1] = shapes[new_type][i][1];
}
if (!check_collision(cur_x, cur_y, new_type)) {
cur_type = new_type;
for (int i = ; i < 4; i++) {
cur_shape[i][] = new_shape[i][];
cur_shape[i][1] = new_shape[i][1];
}
}
} else if (c == 'q') {
break;
}
}
Sleep(100);
}
}
int main()
{
init_game();
game_loop();
printf("Game over!\n");
return ;
}
用c++写俄罗斯方块 代码
非常感谢您的提问!以下是用 C 语言编写俄罗斯方块的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 10
#define HEIGHT 20
int board[HEIGHT][WIDTH] = {0};
int shapes[7][4][2] = {
{{0, 0}, {0, 1}, {1, 0}, {1, 1}}, // O
{{0, 0}, {0, 1}, {0, 2}, {0, 3}}, // I
{{0, 0}, {0, 1}, {0, 2}, {1, 2}}, // L
{{0, 0}, {0, 1}, {0, 2}, {-1, 2}}, // J
{{0, 0}, {0, 1}, {1, 1}, {1, 2}}, // S
{{0, 0}, {0, 1}, {-1, 1}, {1, 0}}, // Z
{{0, 0}, {0, 1}, {0, 2}, {1, 1}} // T
};
int current_shape[4][2];
int current_x = WIDTH / 2 - 1;
int current_y = 0;
int current_type = -1;
int score = 0;
void draw_board() {
system("cls");
printf("Score: %d\n", score);
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
printf(".");
} else {
printf("*");
}
}
printf("\n");
}
}
void generate_shape() {
if (current_type == -1) {
current_type = rand() % 7;
}
for (int i = 0; i < 4; i++) {
current_shape[i][0] = shapes[current_type][i][0];
current_shape[i][1] = shapes[current_type][i][1];
}
}
int check_collision(int x, int y) {
for (int i = 0; i < 4; i++) {
int new_x = x + current_shape[i][0];
int new_y = y + current_shape[i][1];
if (new_x < 0 || new_x >= WIDTH || new_y < 0 || new_y >= HEIGHT) {
return 1;
}
if (board[new_y][new_x] != 0) {
return 1;
}
}
return 0;
}
void place_shape() {
for (int i = 0; i < 4; i++) {
int x = current_x + current_shape[i][0];
int y = current_y + current_shape[i][1];
board[y][x] = 1;
}
current_type = -1;
score += 10;
}
void remove_full_lines() {
int full_lines = 0;
for (int i = HEIGHT - 1; i >= 0; i--) {
int is_full = 1;
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
is_full = 0;
break;
}
}
if (is_full) {
full_lines++;
for (int k = i; k > 0; k--) {
for (int j = 0; j < WIDTH; j++) {
board[k][j] = board[k - 1][j];
}
}
for (int j = 0; j < WIDTH; j++) {
board[0][j] = 0;
}
i++;
}
}
score += full_lines * 100;
}
void game_over() {
printf("Game Over!\n");
printf("Your score: %d\n", score);
exit(0);
}
void game_loop() {
while (1) {
if (current_type == -1) {
generate_shape();
current_x = WIDTH / 2 - 1;
current_y = 0;
if (check_collision(current_x, current_y)) {
game_over();
}
}
draw_board();
if (_kbhit()) {
char c = _getch();
if (c == 'a') {
if (!check_collision(current_x - 1, current_y)) {
current_x--;
}
} else if (c == 'd') {
if (!check_collision(current_x + 1, current_y)) {
current_x++;
}
} else if (c == 's') {
if (!check_collision(current_x, current_y + 1)) {
current_y++;
}
} else if (c == ' ') {
while (!check_collision(current_x, current_y + 1)) {
current_y++;
}
} else if (c == 'q') {
exit(0);
}
}
if (check_collision(current_x, current_y + 1)) {
place_shape();
remove_full_lines();
} else {
current_y++;
}
Sleep(100);
}
}
int main() {
srand(time(NULL));
game_loop();
return 0;
}
```
希望这个代码示例能够帮助您!如果您有任何问题或需要进一步的帮助,请随时联系我。
相关推荐
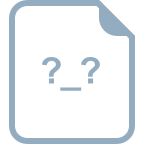












