使用c++编写一个俄罗斯方块游戏
时间: 2023-06-17 22:04:16 浏览: 96
好的,以下是一个简单的俄罗斯方块游戏的代码。代码中使用了Windows API库,如果是在其他平台上实现,需要修改相关的库和函数。
```c++
#include <iostream>
#include <conio.h>
#include <Windows.h>
using namespace std;
const int WIDTH = 10;
const int HEIGHT = 20;
const int BLOCK_SIZE = 20;
const int MAX_BLOCK_TYPE = 7;
int BLOCK_DATA[MAX_BLOCK_TYPE][4][4][4] = {
// I
{
{
{0,0,0,0},
{1,1,1,1},
{0,0,0,0},
{0,0,0,0}
},
{
{0,0,1,0},
{0,0,1,0},
{0,0,1,0},
{0,0,1,0}
},
{
{0,0,0,0},
{0,0,0,0},
{1,1,1,1},
{0,0,0,0},
},
{
{0,1,0,0},
{0,1,0,0},
{0,1,0,0},
{0,1,0,0}
}
},
// J
{
{
{1,0,0,0},
{1,1,1,0},
{0,0,0,0},
{0,0,0,0}
},
{
{0,1,1,0},
{0,1,0,0},
{0,1,0,0},
{0,0,0,0}
},
{
{0,0,0,0},
{1,1,1,0},
{0,0,1,0},
{0,0,0,0}
},
{
{0,1,0,0},
{0,1,0,0},
{1,1,0,0},
{0,0,0,0}
}
},
// L
{
{
{0,0,1,0},
{1,1,1,0},
{0,0,0,0},
{0,0,0,0}
},
{
{0,1,0,0},
{0,1,0,0},
{0,1,1,0},
{0,0,0,0}
},
{
{0,0,0,0},
{1,1,1,0},
{1,0,0,0},
{0,0,0,0}
},
{
{1,1,0,0},
{0,1,0,0},
{0,1,0,0},
{0,0,0,0}
}
},
// O
{
{
{0,0,0,0},
{0,1,1,0},
{0,1,1,0},
{0,0,0,0}
},
{
{0,0,0,0},
{0,1,1,0},
{0,1,1,0},
{0,0,0,0}
},
{
{0,0,0,0},
{0,1,1,0},
{0,1,1,0},
{0,0,0,0}
},
{
{0,0,0,0},
{0,1,1,0},
{0,1,1,0},
{0,0,0,0}
}
},
// S
{
{
{0,1,1,0},
{1,1,0,0},
{0,0,0,0},
{0,0,0,0}
},
{
{0,1,0,0},
{0,1,1,0},
{0,0,1,0},
{0,0,0,0}
},
{
{0,0,0,0},
{0,1,1,0},
{1,1,0,0},
{0,0,0,0}
},
{
{1,0,0,0},
{1,1,0,0},
{0,1,0,0},
{0,0,0,0}
}
},
// T
{
{
{0,1,0,0},
{1,1,1,0},
{0,0,0,0},
{0,0,0,0}
},
{
{0,1,0,0},
{0,1,1,0},
{0,1,0,0},
{0,0,0,0}
},
{
{0,0,0,0},
{1,1,1,0},
{0,1,0,0},
{0,0,0,0}
},
{
{0,1,0,0},
{1,1,0,0},
{0,1,0,0},
{0,0,0,0}
}
},
// Z
{
{
{1,1,0,0},
{0,1,1,0},
{0,0,0,0},
{0,0,0,0}
},
{
{0,0,1,0},
{0,1,1,0},
{0,1,0,0},
{0,0,0,0}
},
{
{0,0,0,0},
{1,1,0,0},
{0,1,1,0},
{0,0,0,0}
},
{
{0,1,0,0},
{1,1,0,0},
{1,0,0,0},
{0,0,0,0}
}
}
};
struct Point {
int x, y;
};
class Tetris {
public:
Tetris() {
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
map[i][j] = 0;
}
}
curBlock = getRandomBlock();
curPos = { WIDTH / 2, 0 };
gameOver = false;
}
void run() {
while (!gameOver) {
update();
draw();
Sleep(500);
}
}
private:
void update() {
if (isCollided(curBlock, curPos.x, curPos.y + 1)) {
mergeBlock();
removeFullLines();
curBlock = getRandomBlock();
curPos = { WIDTH / 2, 0 };
if (isCollided(curBlock, curPos.x, curPos.y)) {
gameOver = true;
}
}
else {
curPos.y++;
}
}
void draw() {
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (map[i][j]) {
drawBlock(j, i);
}
else {
drawEmptyBlock(j, i);
}
}
cout << endl;
}
}
void drawBlock(int x, int y) {
HANDLE hOut = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleCursorPosition(hOut, { x * BLOCK_SIZE, y });
SetConsoleTextAttribute(hOut, 15);
cout << "■";
}
void drawEmptyBlock(int x, int y) {
HANDLE hOut = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleCursorPosition(hOut, { x * BLOCK_SIZE, y });
SetConsoleTextAttribute(hOut, 7);
cout << "□";
}
void mergeBlock() {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (curBlock[i][j]) {
map[curPos.y + i][curPos.x + j] = 1;
}
}
}
}
bool isCollided(int block[4][4], int x, int y) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[i][j]) {
int nx = x + j;
int ny = y + i;
if (nx < 0 || nx >= WIDTH || ny >= HEIGHT) {
return true;
}
if (ny >= 0 && map[ny][nx]) {
return true;
}
}
}
}
return false;
}
void removeFullLines() {
int lines = 0;
for (int i = HEIGHT - 1; i >= 0; i--) {
bool full = true;
for (int j = 0; j < WIDTH; j++) {
if (!map[i][j]) {
full = false;
break;
}
}
if (full) {
lines++;
for (int k = i; k > 0; k--) {
for (int j = 0; j < WIDTH; j++) {
map[k][j] = map[k - 1][j];
}
}
for (int j = 0; j < WIDTH; j++) {
map[0][j] = 0;
}
i++;
}
}
}
int getRandomBlock() {
return BLOCK_DATA[rand() % MAX_BLOCK_TYPE];
}
private:
int map[HEIGHT][WIDTH];
int curBlock[4][4];
Point curPos;
bool gameOver;
};
int main() {
Tetris game;
game.run();
return 0;
}
```
这个代码实现的俄罗斯方块游戏比较简单,没有实现计分和难度逐渐增加等功能,但是可以作为一个基础的实现来学习。
阅读全文
相关推荐
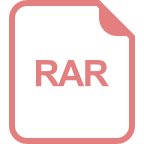

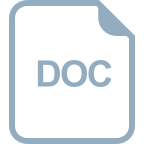



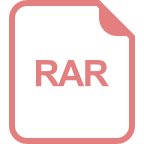
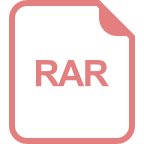
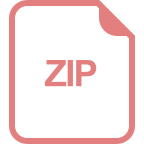
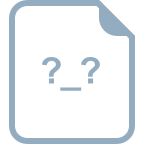





