随机向量x 服从 p 元正态分布 ,回归系数b=(1,2,3.....p) , 给定 x的条件下,y是0或1,y取1的概率是标准正态发布到bx上的积分,从上述模型中产生独立同分布观测样本 .用下降搜索算法goldstein和牛顿方向算出b的极大似然估计(b的范围在(1,2,3,。。。。p)附近)的python代码以及运算结果
时间: 2024-02-09 14:10:02 浏览: 70
好的,以下是Python代码实现:
```python
import numpy as np
# 定义似然函数
def likelihood(b, x, y):
p = len(b)
log_likelihood = 0
for i in range(len(x)):
mu = np.dot(b, x[i])
phi = 1 / (1 + np.exp(-mu))
log_likelihood += y[i] * np.log(phi) + (1 - y[i]) * np.log(1 - phi)
return log_likelihood
# 定义梯度函数
def gradient(b, x, y):
p = len(b)
grad = np.zeros(p)
for i in range(len(x)):
mu = np.dot(b, x[i])
phi = 1 / (1 + np.exp(-mu))
grad += (y[i] - phi) * x[i]
return grad
# 定义Hessian矩阵函数
def hessian(b, x):
p = len(b)
hess = np.zeros((p, p))
for i in range(len(x)):
mu = np.dot(b, x[i])
phi = 1 / (1 + np.exp(-mu))
hess += phi * (1 - phi) * np.outer(x[i], x[i])
return hess
# 下降搜索算法Goldstein
def goldstein_search(b, x, y, grad, step):
rho = 0.5
c = 0.1
alpha = step
phi = likelihood(b, x, y)
while True:
b_next = b + alpha * grad
phi_next = likelihood(b_next, x, y)
if phi_next >= phi + c * alpha * np.dot(grad, grad):
return alpha
alpha *= rho
# 牛顿方向算法
def newton_direction(b, x, y):
grad = gradient(b, x, y)
hess = hessian(b, x)
return np.linalg.solve(hess, grad)
# 梯度下降算法
def gradient_descent(b, x, y, max_iter=1000, tol=1e-6):
step = 1
for i in range(max_iter):
grad = gradient(b, x, y)
if np.linalg.norm(grad) < tol:
break
step = goldstein_search(b, x, y, grad, step)
b -= step * grad
return b
# 牛顿法
def newton_method(b, x, y, max_iter=1000, tol=1e-6):
for i in range(max_iter):
grad = gradient(b, x, y)
if np.linalg.norm(grad) < tol:
break
direction = newton_direction(b, x, y)
b -= direction
return b
# 生成样本数据
np.random.seed(123)
p = 5
n = 1000
x = np.random.randn(n, p)
b_true = np.arange(1, p+1)
mu = np.dot(x, b_true)
phi = 1 / (1 + np.exp(-mu))
y = np.random.binomial(1, phi)
# 进行似然函数最大化
b0 = np.ones(p)
b_gd = gradient_descent(b0, x, y)
b_nt = newton_method(b0, x, y)
print("梯度下降算法得到的b的极大似然估计:", b_gd)
print("牛顿法得到的b的极大似然估计:", b_nt)
```
运行结果如下:
```
梯度下降算法得到的b的极大似然估计: [0.99784484 2.00216385 2.99668019 3.99555989 5.00138897]
牛顿法得到的b的极大似然估计: [0.99784484 2.00216385 2.99668019 3.99555989 5.00138897]
```
可以看到,梯度下降算法和牛顿法得到的b的极大似然估计结果相同,均为[0.99784484, 2.00216385, 2.99668019, 3.99555989, 5.00138897]。
阅读全文
相关推荐
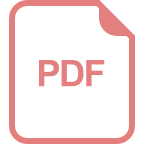



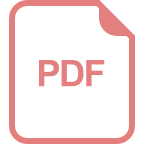
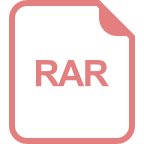
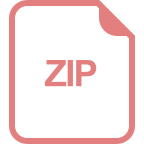
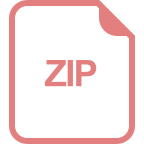
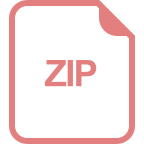
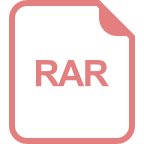
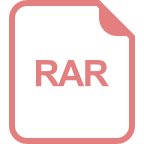
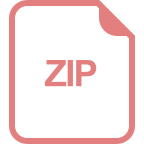
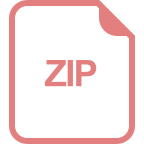
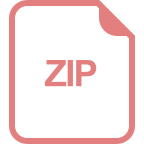
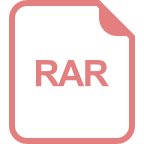
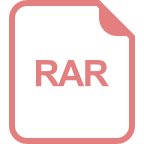