pythony旅行商问题代码
时间: 2024-06-17 13:02:25 浏览: 93
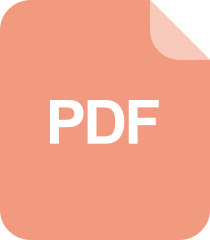
Pythony运维入门之Socket网络编程详解
旅行商问题(Traveling Salesman Problem,TSP)是一个经典的组合优化问题,目标是找到一条路径,使得旅行商能够访问一系列城市并返回起始城市,同时路径的总长度最小。
以下是一个使用Python解决TSP问题的示例代码:
```python
import numpy as np
from itertools import permutations
def tsp(cities):
# 计算两个城市之间的距离
def distance(city1, city2):
return np.linalg.norm(np.array(city1) - np.array(city2))
# 计算路径的总长度
def path_length(path):
length = 0
for i in range(len(path) - 1):
length += distance(path[i], path[i+1])
return length
# 获取所有城市的排列组合
all_permutations = permutations(cities)
# 初始化最短路径和最短长度
shortest_path = None
shortest_length = float('inf')
# 遍历所有排列组合
for path in all_permutations:
length = path_length(path)
if length < shortest_length:
shortest_length = length
shortest_path = path
return shortest_path, shortest_length
# 示例用法
cities = [(0, 0), (1, 1), (2, 3), (5, 2)]
shortest_path, shortest_length = tsp(cities)
print("最短路径:", shortest_path)
print("最短长度:", shortest_length)
```
这段代码使用了numpy库来计算两个城市之间的距离,并使用itertools库的permutations函数获取所有城市的排列组合。然后,通过遍历所有排列组合,计算路径的总长度,并找到最短路径和最短长度。
阅读全文
相关推荐
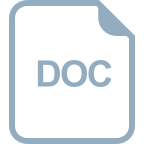
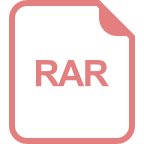
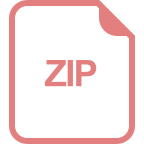
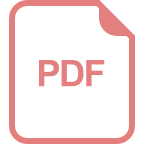
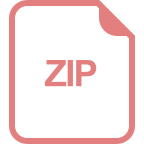











