#include<stdio.h> #include<math.h> int max(int x,int y){ if(x>y) return x; } const int MAXN=1e6; int Max[MAXN][21]; inline int read(){ int x=0,f=1;char ch=getchar(); while(ch<'0'||ch>'9'){ if (ch=='-') f=-1;ch=getchar(); } while(ch>='0'&&ch<='9'){ x=x*10+ch-48;ch=getchar(); } return x*f; } int Query(int l,int r){ int k=log(r-l+1)/log(2); return max(Max[l][k],Max[r-(1<<k)+1][k]); } int main(){ int i,j,n,m; n=read(),m=read(); for(i=1;i<=n;i++){ Max[i][0]=read(); } printf("%d %d\n",n,m); for(i=1;i<=n;i++){ printf("%d",Max[i][0]); } printf("\n"); for(j=1;j<=log(n)/log(2);j++) for(i=1;i<=n-(1<<j)+1;i++) Max[i][j]=max(Max[i][j-1],Max[i+(1<<(j-1))][j-1]); for(i=1;i<=m;i++){ int l=read(),r=read(); printf("%d\n",Query(l,r)); } return 0; }
时间: 2024-04-17 16:23:22 浏览: 81
这是一个简单的查询区间最大值的程序。首先,它定义了一个`max`函数用于比较两个数的大小。然后,它定义了一个常量`MAXN`作为数组的大小。接下来,它使用`read`函数读取输入的数组大小和查询次数,并将数组的值存储在`Max`数组中。然后,它使用动态规划的方法预处理数组,计算出每个区间的最大值。最后,它使用`Query`函数来查询指定区间的最大值,并将结果打印出来。
相关问题
#include <stdio.h> #include <math.h> #define __1__ x*x-5.5*x+sin(x) int main() { float x,max; max=__2__; for(x=0.5;x<=10;___3___) if(f(x)>max) ___4___; printf("%f\n",max); }
根据您提供的代码,我猜测您想让我填写四个空白处,使得程序能够正确地输出结果。下面是我的回答:
1. #define f(x) x*x-5.5*x+sin(x)
2. f(0.5)
3. x+=0.1
4. max=f(x)
解释如下:
1. 在第一个空白处,应该定义一个宏,用于计算函数 f(x) 的值。因为 f(x) 的计算公式被多次使用,可以用 define 来定义一个宏,方便计算。
2. 在第二个空白处,应该填写 f(0.5),这是因为需要求出函数 f(x) 在 x=0.5 时的值,并将其赋给变量 max。
3. 在第三个空白处,应该填写 x+=0.1,这是因为需要对变量 x 进行循环遍历,每次增加 0.1 的步长。
4. 在第四个空白处,应该填写 max=f(x),这是因为需要找到函数 f(x) 在 [0.5,10] 区间内的最大值,并将其赋给变量 max。
填完空白后,完整代码如下:
```c
#include <stdio.h>
#include <math.h>
#define f(x) x*x-5.5*x+sin(x)
int main() {
float x, max;
max = f(0.5);
for(x = 0.5; x <= 10; x += 0.1) {
if(f(x) > max)
max = f(x);
}
printf("%f\n", max);
return 0;
}
```
该程序先通过 f(0.5) 求出函数 f(x) 在 x=0.5 时的值,并将其赋给变量 max。然后,通过 for 循环遍历 [0.5,10] 区间内的每个 x 值,并计算函数 f(x) 的值。如果 f(x) 的值大于当前的 max 值,就将 f(x) 的值赋给 max。最终,程序输出 max 的值,即函数 f(x) 在 [0.5,10] 区间内的最大值。
#include<stdio.h> #include<stdlib.h> #include<math.h> #include<time.h> #define N 50 typedef struct process
你提到的代码片段似乎是在C语言中定义了一个结构体(struct)来表示进程(process)。在这个上下文中,`#include`指令引入了必要的库,如`stdio.h`用于输入输出,`stdlib.h`用于标准库函数,`math.h`用于数学运算,`time.h`用于时间处理。
`#define N 50` 定义了一个名为N的宏,通常用于设定数组或过程数量的上限,这里是50。
`typedef struct process` 是用来创建一种新的数据类型,可能是`process`类型的别名,但没有完全展开结构体的内容。在C语言中,可能如下所示:
```c
typedef struct {
// 进程相关的属性(如ID, 优先级, 时间戳等)
int id;
float priority;
time_t timestamp;
// 其他可能的成员
} Process;
```
要实际演示或使用这个结构体,你需要定义它的实例,操作其成员,比如创建进程、排序或管理它们:
```c
Process processes[N]; // 创建一个大小为N的进程数组
// 假设有一个函数用于初始化进程
void initialize_processes(Process processes[]) {
for (int i = 0; i < N; i++) {
processes[i].id = i + 1;
processes[i].priority = rand() / (float)RAND_MAX; // 随机优先级
processes[i].timestamp = time(NULL); // 设置当前时间
}
}
// 示例:找到具有最高优先级的进程
Process* find_highest_priority(Process processes[], int n) {
Process* max_priority_process = &processes;
for (int i = 1; i < n; i++) {
if (processes[i].priority > max_priority_process->priority) {
max_priority_process = &processes[i];
}
}
return max_priority_process;
}
int main() {
initialize_processes(processes);
Process* highest_priority = find_highest_priority(processes, N);
printf("Highest priority process: ID %d, Priority %.2f\n",
highest_priority->id, highest_priority->priority);
return 0;
}
```
阅读全文
相关推荐
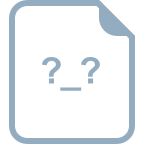
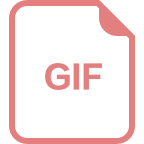















