python路径规划走网格
时间: 2024-08-07 08:01:35 浏览: 110
Python中的路径规划通常涉及到在网格图上找到从起点到终点的最佳路线的问题,这在自动驾驶、游戏AI、机器人导航等领域有着广泛的应用。以下是基于A*算法(A-Star算法)的一个简单的Python示例,用于解决基本的二维网格路径规划问题:
### A* 算法概述
A* 算法是一种启发式搜索算法,在寻找最短路径时非常有效。它结合了Dijkstra算法的优点,并引入了一个启发式函数来估计剩余路径长度,使得算法效率更高。
### 实现步骤
#### 1. 初始化数据结构
- 开放列表(open_list)存放待探索节点。
- 封闭列表(closed_list)存放已探索过节点。
- 使用字典存储每个节点的最小代价到当前节点的距离以及该节点的前驱节点。
#### 2. 定义启发式函数
- **曼哈顿距离**是最常见的启发式函数之一,计算起点到目标点的直线距离。
```python
def heuristic(a, b):
return abs(b - a) + abs(b - a)
```
#### 3. 找到并处理下一个最佳节点
- 首先,选择开放列表中f值(g+启发式h值)最低的节点作为下一个访问的节点,其中g是从起点到当前节点的实际路径长度,h是启发式函数估计的目标到当前节点的距离。
#### 4. 更新邻接节点的状态
- 对于当前节点的所有未访问过的邻居,计算到达它们的总代价,如果这个代价比之前记录的更小,则更新其g值、父节点信息以及将该节点添加到开放列表中。
#### 5. 终止条件与结果回溯
- 当目标节点被加入到封闭列表时,终止循环,然后从目标节点开始逆向遍历其父节点,构建从起点到终点的路径。
### Python 示例代码
```python
import heapq
# 定义网格大小和障碍物位置
grid_size = (10, 10)
obstacles = [(2, 3), (5, 7)]
start = (0, 0)
end = (9, 9)
def is_valid(x, y):
if 0 <= x < grid_size and 0 <= y < grid_size:
return True
else:
return False
def neighbors(node):
x, y = node
for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
next_x, next_y = x + dx, y + dy
if is_valid(next_x, next_y) and (next_x, next_y) not in obstacles:
yield (next_x, next_y)
def reconstruct_path(came_from, current):
path = []
while current in came_from:
path.append(current)
current = came_from[current]
return list(reversed(path))
def astar_search(start, end):
open_list = []
closed_list = set()
g_scores = {start: 0}
f_scores = {start: heuristic(start, end)}
heapq.heappush(open_list, (f_scores[start], start))
came_from = {}
while open_list:
_, current = heapq.heappop(open_list)
if current == end:
return reconstruct_path(came_from, end)
closed_list.add(current)
for neighbor in neighbors(current):
tentative_g_score = g_scores[current] + 1
if tentative_g_score < g_scores.get(neighbor, float('inf')):
came_from[neighbor] = current
g_scores[neighbor] = tentative_g_score
f_scores[neighbor] = tentative_g_score + heuristic(neighbor, end)
if neighbor not in closed_list:
heapq.heappush(open_list, (f_scores[neighbor], neighbor))
# 运行搜索
path = astar_search(start, end)
print("Path found:", path)
```
### 相关问题:
1. **如何优化路径规划算法以提高效率?**
通过优化启发式函数、减少不必要的节点检查和利用更高效的数据结构可以提升A*算法的性能。
2. **在实际应用中,如何处理复杂的障碍环境?**
可以采用高级地图表示(如栅格地图、矢量地图)、动态更新障碍物、或者引入多种障碍检测策略等方法。
3. **如何将路径规划算法应用于非平面空间(例如三维空间)?**
只需扩展网格搜索的概念至更多维度,同时修改启发式函数以适应新的几何特性即可。
阅读全文
相关推荐
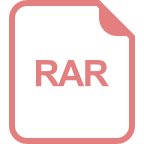
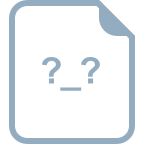
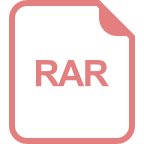




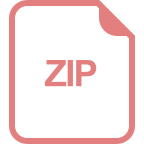
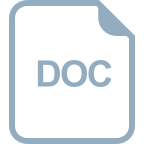
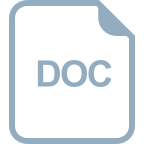
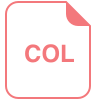







