python 写入mysql
时间: 2023-06-28 18:06:56 浏览: 51
在 Python 中,可以使用 MySQL Connector 模块来连接 MySQL 数据库,并使用 SQL 语句来实现对数据库的读写操作。以下是一个简单的示例,演示如何将数据写入 MySQL 数据库中:
1. 安装 MySQL Connector 模块
```
pip install mysql-connector-python
```
2. 连接 MySQL 数据库
```
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
mycursor = mydb.cursor()
```
3. 创建数据表(如果数据表不存在)
```
mycursor.execute("CREATE TABLE customers (name VARCHAR(255), address VARCHAR(255))")
```
4. 插入数据
```
sql = "INSERT INTO customers (name, address) VALUES (%s, %s)"
val = ("John", "Highway 21")
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record inserted.")
```
以上代码会在 customers 表中插入一条名为 John,地址为 Highway 21 的记录。
如果要插入多条记录,则可以将数据放到一个列表中,然后使用 executemany() 方法:
```
sql = "INSERT INTO customers (name, address) VALUES (%s, %s)"
val = [
('Peter', 'Lowstreet 4'),
('Amy', 'Apple st 652'),
('Hannah', 'Mountain 21'),
('Michael', 'Valley 345'),
('Sandy', 'Ocean blvd 2'),
('Betty', 'Green Grass 1'),
('Richard', 'Sky st 331'),
('Susan', 'One way 98'),
('Vicky', 'Yellow Garden 2'),
('Ben', 'Park Lane 38'),
('William', 'Central st 954'),
('Chuck', 'Main Road 989'),
('Viola', 'Sideway 1633')
]
mycursor.executemany(sql, val)
mydb.commit()
print(mycursor.rowcount, "was inserted.")
```
以上代码会在 customers 表中插入多条记录。
5. 关闭数据库连接
```
mydb.close()
```
以上就是一个简单的将数据写入 MySQL 数据库的 Python 示例。
相关推荐
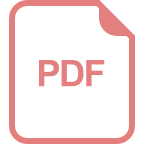
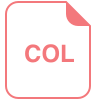
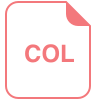
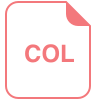
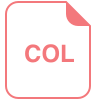
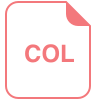









