gd32通过高级定时器中断控制bldc的代码
时间: 2023-06-14 19:06:16 浏览: 172
以下是使用GD32高级定时器中断控制BLDC的基本代码框架。在这个例子中,我们使用了TIM1来控制BLDC的三相电机。
首先,需要在初始化函数中配置TIM1高级定时器,将它配置为PWM模式,并且设置计数器自动重载值。然后,配置TIM1的三个通道,使它们输出PWM波形。
然后,我们需要定义一个中断处理函数,在中断处理函数中,我们将更新三个PWM通道的占空比,以控制BLDC电机的转速。同时,我们还需要处理BLDC电机的正反转和停止操作。
最后,在主函数中,我们可以调用一个函数,使BLDC电机开始旋转。在这个例子中,我们使用了无定时器的方式来控制BLDC电机的转速,因此可以通过改变PWM占空比来改变电机的转速。
```
#include "gd32f30x.h"
#define PWM_FREQ 20000 // PWM频率
#define PWM_DUTY_CYCLE 50 // PWM占空比
// BLDC电机状态
enum {
STOP,
CW,
CCW
} bldc_state = STOP;
// BLDC电机相序表
const uint8_t bldc_phases[6] = {1, 5, 4, 6, 2, 3};
// BLDC电机相序
uint8_t bldc_phase = 0;
// 中断处理函数
void TIMER1_IRQHandler(void)
{
// 清除中断标志位
timer_interrupt_flag_clear(TIMER1, TIMER_INT_FLAG_CH0 | TIMER_INT_FLAG_CH1 | TIMER_INT_FLAG_CH2);
// 更新PWM占空比
if (bldc_state == CW) {
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_0, PWM_DUTY_CYCLE * 10);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_1, 0);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_2, 0);
} else if (bldc_state == CCW) {
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_0, 0);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_1, PWM_DUTY_CYCLE * 10);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_2, 0);
} else {
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_0, 0);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_1, 0);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_2, 0);
}
// 更新BLDC电机相序
if (bldc_phase < 6) {
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_3, PWM_DUTY_CYCLE * bldc_phases[bldc_phase]);
} else {
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_3, 0);
}
bldc_phase++;
if (bldc_phase > 11) {
bldc_phase = 0;
}
}
// 初始化函数
void init(void)
{
// 使能GPIO和TIMER1时钟
rcu_periph_clock_enable(RCU_GPIOA);
rcu_periph_clock_enable(RCU_TIMER1);
// 配置GPIO
gpio_init(GPIOA, GPIO_MODE_AF_PP, GPIO_OSPEED_50MHZ, GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11);
// 配置TIMER1
timer_deinit(TIMER1);
timer_oc_parameter_struct timer_ocinitpara;
timer_parameter_struct timer_initpara;
timer_struct_para_init(&timer_initpara);
timer_initpara.prescaler = SystemCoreClock / (PWM_FREQ * 1000) - 1;
timer_initpara.alignedmode = TIMER_COUNTER_EDGE_ALIGNED_PWM_MODE;
timer_initpara.counterdirection = TIMER_COUNTER_UP;
timer_initpara.period = 100 - 1;
timer_initpara.clockdivision = TIMER_CKDIV_DIV1;
timer_init(TIMER1, &timer_initpara);
timer_struct_para_init(&timer_ocinitpara);
timer_ocinitpara.ocpolarity = TIMER_OC_POLARITY_HIGH;
timer_ocinitpara.ocnpolarity = TIMER_OCN_POLARITY_HIGH;
timer_ocinitpara.outputstate = TIMER_CCX_ENABLE;
timer_ocinitpara.outputnstate = TIMER_CCXN_DISABLE;
timer_ocinitpara.ocidlestate = TIMER_OC_IDLE_STATE_LOW;
timer_ocinitpara.ocnidlestate = TIMER_OCN_IDLE_STATE_LOW;
timer_channel_output_config(TIMER1, TIMER_CH_0, &timer_ocinitpara);
timer_channel_output_config(TIMER1, TIMER_CH_1, &timer_ocinitpara);
timer_channel_output_config(TIMER1, TIMER_CH_2, &timer_ocinitpara);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_0, 0);
timer_channel_output_mode_config(TIMER1, TIMER_CH_0, TIMER_OC_MODE_PWM0);
timer_channel_output_shadow_config(TIMER1, TIMER_CH_0, TIMER_OC_SHADOW_DISABLE);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_1, 0);
timer_channel_output_mode_config(TIMER1, TIMER_CH_1, TIMER_OC_MODE_PWM0);
timer_channel_output_shadow_config(TIMER1, TIMER_CH_1, TIMER_OC_SHADOW_DISABLE);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_2, 0);
timer_channel_output_mode_config(TIMER1, TIMER_CH_2, TIMER_OC_MODE_PWM0);
timer_channel_output_shadow_config(TIMER1, TIMER_CH_2, TIMER_OC_SHADOW_DISABLE);
timer_channel_output_config(TIMER1, TIMER_CH_3, &timer_ocinitpara);
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_3, 0);
timer_channel_output_mode_config(TIMER1, TIMER_CH_3, TIMER_OC_MODE_PWM0);
timer_channel_output_shadow_config(TIMER1, TIMER_CH_3, TIMER_OC_SHADOW_DISABLE);
// 配置中断
nvic_irq_enable(TIMER1_IRQn, 0, 0);
timer_interrupt_enable(TIMER1, TIMER_INT_CH0 | TIMER_INT_CH1 | TIMER_INT_CH2);
// 启动TIMER1
timer_enable(TIMER1);
}
// BLDC电机启动函数
void bldc_start(void)
{
bldc_state = CW;
}
// BLDC电机停止函数
void bldc_stop(void)
{
bldc_state = STOP;
bldc_phase = 0;
timer_channel_output_pulse_value_config(TIMER1, TIMER_CH_3, 0);
}
// BLDC电机反转函数
void bldc_reverse(void)
{
if (bldc_state == CW) {
bldc_state = CCW;
} else if (bldc_state == CCW) {
bldc_state = CW;
}
}
int main(void)
{
init();
bldc_start();
while (1) {
// 等待中断
}
}
```
阅读全文
相关推荐
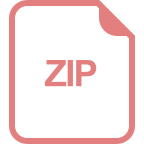


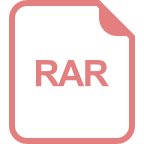
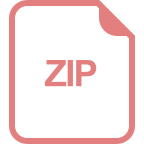
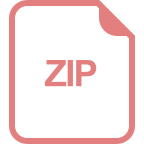
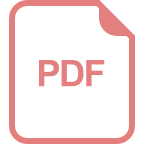
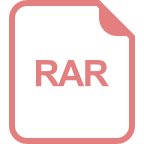
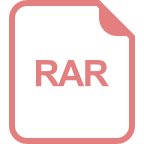
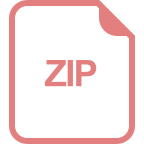
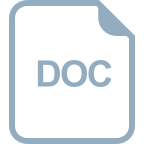
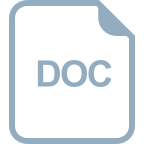


