本项目有一部分时间戳数据,请编写Mapreduce程序将这些时间戳按升序进行排序,同时转换成对应的date时间。 例如: 1100186731 1100185961 排序转换后: 1100185961, Thu Nov 11 23:12:41 CST 2004 1100186731, Thu Nov 11 23:25:31 CST 2004
时间: 2024-03-06 21:46:09 浏览: 32
以下是一个基本的MapReduce程序,用于按升序对时间戳进行排序,并将其转换为对应的date时间。
```java
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.TextInputFormat;
import org.apache.hadoop.mapreduce.lib.output.TextOutputFormat;
public class TimestampSorter {
public static class TimestampMapper
extends Mapper<LongWritable, Text, LongWritable, Text> {
private final SimpleDateFormat formatter = new SimpleDateFormat(
"EEE MMM dd HH:mm:ss zzz yyyy", Locale.US);
@Override
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
long timestamp = Long.parseLong(value.toString());
Date date = new Date(timestamp * 1000);
String dateString = formatter.format(date);
context.write(new LongWritable(timestamp), new Text(dateString));
}
}
public static class TimestampReducer
extends Reducer<LongWritable, Text, LongWritable, Text> {
@Override
public void reduce(LongWritable key, Iterable<Text> values, Context context)
throws IOException, InterruptedException {
for (Text value : values) {
context.write(key, value);
}
}
}
public static void main(String[] args)
throws IOException, ClassNotFoundException, InterruptedException {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Timestamp Sorter");
job.setJarByClass(TimestampSorter.class);
job.setMapperClass(TimestampMapper.class);
job.setReducerClass(TimestampReducer.class);
job.setOutputKeyClass(LongWritable.class);
job.setOutputValueClass(Text.class);
job.setInputFormatClass(TextInputFormat.class);
job.setOutputFormatClass(TextOutputFormat.class);
TextInputFormat.addInputPath(job, new Path(args[0]));
TextOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在该程序中,我们使用一个Mapper将时间戳转换为date时间,并使用时间戳作为键和date时间作为值将其写入上下文。然后,我们使用一个Reducer将这些键值对按升序排列,并将其写入输出。
要运行此程序,您需要将其打包为一个JAR文件,并将其提交到Hadoop集群。您可以使用以下命令来运行程序:
```bash
hadoop jar timestamp-sorter.jar TimestampSorter input output
```
其中,`input`是输入文件的路径,`output`是输出文件的路径。
程序将在完成后生成一个包含按升序排序的时间戳及其对应的date时间的输出文件。
相关推荐
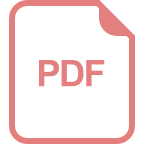
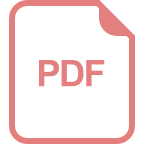
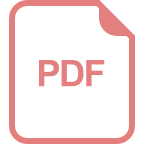






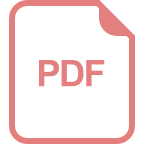
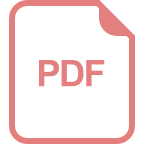
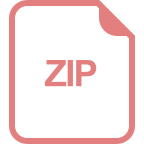
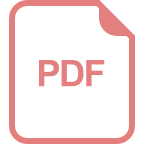
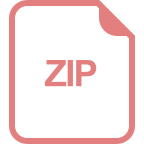