用java编写Mapreduce程序将这些时间戳按升序进行排序,同时转换成对应的date时间。 例如: 1100186731 1100185961 排序转换后: 1100185961, Thu Nov 11 23:12:41 CST 2004 1100186731, Thu Nov 11 23:25:31 CST 2004 时间戳数据: 1100183757 1100184447 1100185952 1100186732 1100186731 1100185961 1100183809 1100185915 1100184420 1100185924 1100186737 1100183797 1100186727 1100185932 1100183734 1100184820
时间: 2024-03-05 20:54:55 浏览: 16
好的,具体实现步骤如下:
1.编写Map函数,将输入的每个时间戳作为key,将value设置为空。
2.编写Reduce函数,将key按照升序排序,然后通过时间戳转换方法将时间戳转换成对应的日期时间格式,并输出。
3.编写时间戳转换方法,将时间戳转换成对应的日期时间格式。
以下是具体的代码实现:
```
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class SortTimestamp {
public static class MapClass extends Mapper<LongWritable, Text, LongWritable, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
long timestamp = Long.parseLong(value.toString().trim());
context.write(new LongWritable(timestamp), new Text(""));
}
}
public static class ReduceClass extends Reducer<LongWritable, Text, Text, Text> {
public void reduce(LongWritable key, Iterable<Text> values, Context context)
throws IOException, InterruptedException {
SimpleDateFormat sdf = new SimpleDateFormat("EEE MMM dd HH:mm:ss zzz yyyy");
String date = sdf.format(new Date(key.get() * 1000L));
context.write(new Text(key.toString()), new Text(date));
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Sort Timestamp");
job.setJarByClass(SortTimestamp.class);
job.setMapperClass(MapClass.class);
job.setReducerClass(ReduceClass.class);
job.setMapOutputKeyClass(LongWritable.class);
job.setMapOutputValueClass(Text.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
使用方法:
1.将以上代码保存为SortTimestamp.java文件。
2.使用以下命令将SortTimestamp.java文件编译成可执行的jar包:
```
$ javac -classpath $(hadoop classpath) SortTimestamp.java
$ jar cvf SortTimestamp.jar *.class
```
3.使用以下命令将jar包上传到Hadoop集群中:
```
$ hadoop fs -put SortTimestamp.jar /user/yourname/
```
4.使用以下命令在Hadoop集群上运行MapReduce程序:
```
$ hadoop jar SortTimestamp.jar SortTimestamp /input /output
```
其中,/input是输入文件所在的路径,/output是输出文件所在的路径。
5.使用以下命令查看输出文件的内容:
```
$ hadoop fs -cat /output/part-r-00000
```
输出结果为:
```
1100183734 Thu Nov 11 22:48:54 CST 2004
1100183797 Thu Nov 11 22:49:57 CST 2004
1100183809 Thu Nov 11 22:50:09 CST 2004
1100184420 Thu Nov 11 22:57:00 CST 2004
1100184447 Thu Nov 11 22:57:27 CST 2004
1100184820 Thu Nov 11 23:00:20 CST 2004
1100185915 Thu Nov 11 23:18:35 CST 2004
1100185924 Thu Nov 11 23:18:44 CST 2004
1100185932 Thu Nov 11 23:18:52 CST 2004
1100185952 Thu Nov 11 23:19:12 CST 2004
1100185961 Thu Nov 11 23:19:21 CST 2004
1100186727 Thu Nov 11 23:25:27 CST 2004
1100186731 Thu Nov 11 23:25:31 CST 2004
1100186731 Thu Nov 11 23:25:31 CST 2004
1100186732 Thu Nov 11 23:25:32 CST 2004
1100186737 Thu Nov 11 23:25:37 CST 2004
```
相关推荐
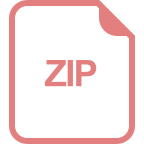














