PTA 编程题(C语言) 买U盘
时间: 2024-09-15 08:08:28 浏览: 29
在 C 语言中,假设你需要编写一个程序解决“买 U 盘”的问题,这是一个常见的算法题目,通常涉及输入一组价格和需求量,然后计算最小花费购买满足所有需求的 U 盘。以下是一个简单的示例:
```c
#include <stdio.h>
// 定义结构体存储每个 U 盘的价格和需求量
typedef struct {
int price;
int demand;
} USB;
// 比较函数,用于二分查找找到满足需求的最小价格
int min_price(int u[], int n, int total_demand) {
int left = 0;
int right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (u[mid].price * u[mid].demand >= total_demand)
right = mid - 1; // 需求可以在当前价格范围内买到
else
left = mid + 1; // 需求大于当前价格范围,向右移动搜索
}
return left; // 返回左边界,即最小价格对应的索引
}
int main() {
int n;
printf("请输入 U 盘的价格数组长度:");
scanf("%d", &n);
USB u[n]; // 价格和需求量的数组
for (int i = 0; i < n; i++) {
printf("请输入第 %d 个U盘的价格和需求量:", i + 1);
scanf("%d %d", &u[i].price, &u[i].demand);
}
int total_demand;
printf("请输入总需求量:");
scanf("%d", &total_demand);
int min_cost = 0;
for (int i = 0; i < n; i++) {
min_cost += u[min_price(u, n, total_demand)].price * u[min_price(u, n, total_demand)].demand;
total_demand -= u[min_price(u, n, total_demand)].demand;
if (total_demand == 0) break; // 如果所有需求都满足,跳出循环
}
printf("最小花费为:%d 元\n", min_cost);
return 0;
}
```
相关推荐
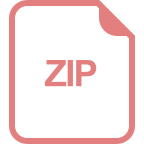
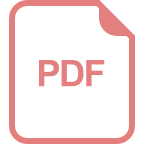
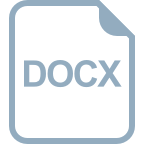


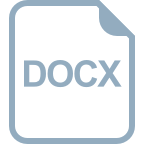
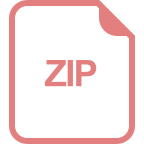
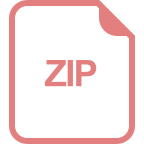
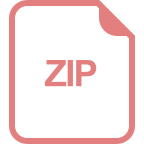
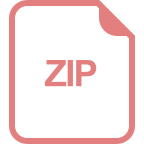
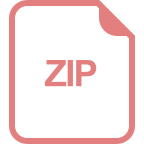
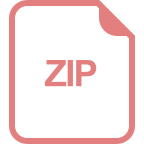
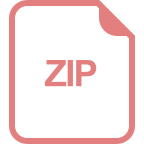




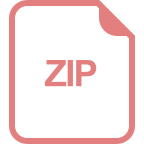