用Python爬取https://www.aigei.com/music/class/?tab=file网页的下载链接
时间: 2023-12-17 20:05:11 浏览: 167
要爬取https://www.aigei.com/music/class/?tab=file网页的下载链接,可以使用Python的requests和BeautifulSoup库。
首先,需要用requests库发送GET请求获取网页的HTML代码。然后,使用BeautifulSoup库解析HTML代码,提取出需要的下载链接。
以下是示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 发送GET请求
url = 'https://www.aigei.com/music/class/?tab=file'
response = requests.get(url)
# 解析HTML代码,提取下载链接
soup = BeautifulSoup(response.text, 'html.parser')
download_links = []
for a in soup.find_all('a', {'class': 'down'}):
download_links.append(a['href'])
# 输出下载链接
print(download_links)
```
运行代码后,会输出所有下载链接。请注意,这些链接可能会经常变化,所以在实际使用中需要进行适当的处理。
相关问题
python爬取https://www.xuanxiaodi.com/ranks/2391-1.html排名
好的,针对您的问题,您可以使用Python的requests库和BeautifulSoup库来实现爬取。
首先,使用requests库发送HTTP请求获取网页内容:
```python
import requests
url = "https://www.xuanxiaodi.com/ranks/2391-1.html"
response = requests.get(url)
html = response.content.decode("utf-8")
```
然后,使用BeautifulSoup库解析HTML文档:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, "html.parser")
rank_list = soup.find("table", {"class": "table-rank"}).tbody.find_all("tr")
for rank in rank_list:
rank_name = rank.find("td", {"class": "rank-name"}).a.string.strip()
print(rank_name)
```
以上代码将输出该网页中所有排名的名称。您可以根据需要自行修改并完善代码。
使用python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集
好的,您可以按照以下步骤使用Python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集:
1. 导入要的库
```python
import requests
from bs4 import BeautifulSoup
import os
```
2. 发送请求并解析网页
```python
url = "https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
```
3. 查找下载链接
```python
download_link = soup.find("a", attrs={"id": "downloadButton"})['href']
```
4. 下载数据集
```python
response = requests.get(download_link, stream=True)
file_size = int(response.headers.get("Content-Length", 0))
filename = os.path.join(os.getcwd(), "oil_spill_detection.zip")
with open(filename, "wb") as f:
for data in response.iter_content(1024):
f.write(data)
```
这样,您就可以使用Python成功地爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection上的数据集。
阅读全文
相关推荐
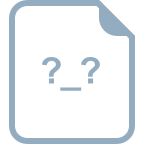













