layers.py import numpy as np ''' 全连接层:矩阵变换,获取对应目标相同的行与列 输入x: 2*32*16*16 输入x_row: 2*8192 超参w:8192*100 输出:矩阵乘法 2*8192 ->8192*100 =>2*100 '''
时间: 2023-08-03 09:06:36 浏览: 41
这段代码是一个注释,描述了一个全连接层的实现。根据注释中的描述,这个全连接层的输入数据 `x` 的形状是 (2, 32, 16, 16),其中 2 是样本数,32 是通道数,16*16 是每个通道的特征数。输入数据 `x` 会被重塑为一个二维矩阵 `x_row`,其中每行是一个样本,每列是一个特征。`x_row` 的形状是 (2, 8192),其中 8192 = 32*16*16。超参 `w` 的形状是 (8192, 100),其中 100 是输出的特征数。
在这个全连接层中,我们需要将输入数据 `x_row` 与超参 `w` 进行矩阵乘法运算,得到一个输出矩阵。输出矩阵的形状是 (2, 100),其中每行是一个样本,每列是一个输出特征。这个全连接层的作用是将输入数据 `x` 中的每个样本映射到一个长度为 100 的向量空间中,从而使得不同的样本之间可以进行比较和分类。
相关问题
import numpy as np import pandas as pd from keras.models import Sequential from keras.layers import Conv1D, MaxPooling1D, Dense, Flatten # 加载数据 data = pd.read_csv('3c_left_1-6.csv') # 将数据转换为三维时序信号 x = data.iloc[:, 0:3].values x = x.reshape(x.shape[0], x.shape[1], 1) # 添加噪声 noise = np.random.normal(0, 1, x.shape) x_noise = x + noise # 构建模型 model = Sequential() model.add(Conv1D(32, kernel_size=3, activation='relu', input_shape=(3, 1))) model.add(MaxPooling1D(pool_size=2)) model.add(Conv1D(64, kernel_size=3, activation='relu')) model.add(MaxPooling1D(pool_size=2)) model.add(Flatten()) model.add(Dense(128, activation='relu')) model.add(Dense(3)) # 编译模型 model.compile(loss='mse', optimizer='adam') # 训练模型 model.fit(x_noise, x, epochs=50, batch_size=32) # 预测结果 x_pred = model.predict(x_noise) # 计算SNR、MSE、PSNR snr = 10 * np.log10(np.sum(x ** 2) / np.sum((x - x_pred) ** 2)) mse = np.mean((x - x_pred) ** 2) psnr = 10 * np.log10((np.max(x) ** 2) / mse) # 保存结果 result = pd.DataFrame({'SNR': [snr], 'MSE': [mse], 'PSNR': [psnr]}) result.to_csv('result.csv', index=False) 报错为File "G:\project2\gaussian.py", line 20, in <module> model.add(MaxPooling1D(pool_size=2))ValueError: Negative dimension size caused by subtracting 2 from 1 for 'max_pooling1d_1/MaxPool' (op: 'MaxPool') with input shapes: [?,1,1,32].
这个错误是因为在添加第一个池化层后,输出张量的空间维度被缩小到了1,而在添加第二个池化层时,池化窗口大小为2,会导致输出张量的空间维度变成负数。解决方法是将第一个池化层的池化窗口大小改为1或者不使用池化层。修改代码如下:
```
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import Conv1D, MaxPooling1D, Dense, Flatten
# 加载数据
data = pd.read_csv('3c_left_1-6.csv')
# 将数据转换为三维时序信号
x = data.iloc[:, 0:3].values
x = x.reshape(x.shape[0], x.shape[1], 1)
# 添加噪声
noise = np.random.normal(0, 1, x.shape)
x_noise = x + noise
# 构建模型
model = Sequential()
model.add(Conv1D(32, kernel_size=3, activation='relu', input_shape=(3, 1)))
model.add(Conv1D(64, kernel_size=3, activation='relu'))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dense(3))
# 编译模型
model.compile(loss='mse', optimizer='adam')
# 训练模型
model.fit(x_noise, x, epochs=50, batch_size=32)
# 预测结果
x_pred = model.predict(x_noise)
# 计算SNR、MSE、PSNR
snr = 10 * np.log10(np.sum(x ** 2) / np.sum((x - x_pred) ** 2))
mse = np.mean((x - x_pred) ** 2)
psnr = 10 * np.log10((np.max(x) ** 2) / mse)
# 保存结果
result = pd.DataFrame({'SNR': [snr], 'MSE': [mse], 'PSNR': [psnr]})
result.to_csv('result.csv', index=False)
```
import pandas as pd import numpy as np import matplotlib.pyplot as plt from keras.models import Model, Input from keras.layers import Conv1D, BatchNormalization, Activation, Add, Flatten, Dense from keras.optimizers import Adam # 读取CSV文件 data = pd.read_csv("3c_left_1-6.csv", header=None) # 将数据转换为Numpy数组 data = data.values # 定义输入形状 input_shape = (data.shape[1], 1) # 定义深度残差网络 def residual_network(inputs): # 第一层卷积层 x = Conv1D(32, 3, padding="same")(inputs) x = BatchNormalization()(x) x = Activation("relu")(x) # 残差块 for i in range(5): y = Conv1D(32, 3, padding="same")(x) y = BatchNormalization()(y) y = Activation("relu")(y) y = Conv1D(32, 3, padding="same")(y) y = BatchNormalization()(y) y = Add()([x, y]) x = Activation("relu")(y) # 全局池化层和全连接层 x = Flatten()(x) x = Dense(128, activation="relu")(x) x = Dense(data.shape[1], activation="linear")(x) outputs = Add()([x, inputs]) return outputs # 构建模型 inputs = Input(shape=input_shape) outputs = residual_network(inputs) model = Model(inputs=inputs, outputs=outputs) # 编译模型 model.compile(loss="mean_squared_error", optimizer=Adam()) # 训练模型 model.fit(data[..., np.newaxis], data[..., np.newaxis, np.newaxis], epochs=100) # 预测数据 predicted_data = model.predict(data[..., np.newaxis]) predicted_data = np.squeeze(predicted_data) # 可视化去噪前后的数据 fig, axs = plt.subplots(3, 1, figsize=(12, 8)) for i in range(3): axs[i].plot(data[:, i], label="Original Signal") axs[i].plot(predicted_data[:, i], label="Denoised Signal") axs[i].legend() plt.savefig("denoised_signal.png") # 将去噪后的数据保存为CSV文件 df = pd.DataFrame(predicted_data, columns=["x", "y", "z"]) df.to_csv("denoised_data.csv", index=False)报错为Traceback (most recent call last): File "G:\project2\main.py", line 51, in <module> model.fit(data[..., np.newaxis], data[..., np.newaxis, np.newaxis], epochs=100) File "G:\python\envs\tensorflow\lib\site-packages\keras\engine\training.py", line 1154, in fit batch_size=batch_size) File "G:\python\envs\tensorflow\lib\site-packages\keras\engine\training.py", line 621, in _standardize_user_data exception_prefix='target') File "G:\python\envs\tensorflow\lib\site-packages\keras\engine\training_utils.py", line 135, in standardize_input_data 'with shape ' + str(data_shape)) ValueError: Error when checking target: expected add_6 to have 3 dimensions, but got array with shape (575206, 3, 1, 1)
这个错误是因为模型的输出是三维的,但是目标数据的维度不匹配。在训练模型时,你传入的目标数据的维度应该是 (batch_size, data_shape),而你传入的数据的维度是 (batch_size, data_shape, 1, 1)。你可以尝试将目标数据的维度改为 (batch_size, data_shape, 1)。修改代码如下:
```
model.fit(data[..., np.newaxis], data[..., np.newaxis], epochs=100)
```
改为:
```
model.fit(data[..., np.newaxis], data[..., np.newaxis], epochs=100)
```
另外,你在定义模型时,最后一层的输出是通过 Add()([x, inputs]) 得到的,这个操作会将输入和输出相加,如果你想要得到去噪后的信号,可以将最后一层的输出改为只有 x,即:
```
outputs = x
```
这样最后得到的就是去噪后的信号了。
相关推荐
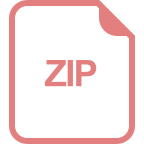
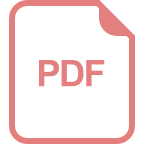
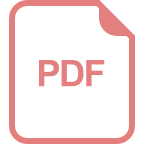











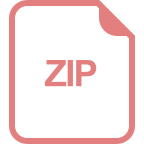