解释一下这段代码def __init__(self, feature_scale=4, n_classes=21, is_deconv=True, in_channels=3, is_batchnorm=True):super(unet_3D_dv_semi, self).__init__() self.is_deconv = is_deconv self.in_channels = in_channels self.is_batchnorm = is_batchnorm self.feature_scale = feature_scale filters = [64, 128, 256, 512, 1024] filters = [int(x / self.feature_scale) for x in filters]
时间: 2024-04-20 16:24:11 浏览: 112
这段代码定义了`unet_3D_dv_semi`类的初始化方法(`__init__`)。初始化方法在创建类的实例时被调用,用于设置类的属性和进行一些初始化操作。
该初始化方法接受一些参数,包括`feature_scale`(特征缩放比例,默认为4)、`n_classes`(类别数量,默认为21)、`is_deconv`(是否使用反卷积层,默认为True)、`in_channels`(输入通道数,默认为3)、`is_batchnorm`(是否使用批归一化层,默认为True)。
在初始化方法中,首先调用父类`nn.Module`的初始化方法,以确保正确地初始化基类的属性。
然后,将传入的参数赋值给类的属性,例如`is_deconv`、`in_channels`、`is_batchnorm`和`feature_scale`。
接下来,创建了一个名为`filters`的列表,其中包含了一系列的数字。这些数字表示用于构建Unet网络的不同层的滤波器数量。通过将这些滤波器数量除以特征缩放比例,可以根据需要调整网络的容量。
最后,返回了初始化后的类实例。
相关问题
class StrainNetF(nn.Module): expansion = 1 def __init__(self,batchNorm=True): super(StrainNetF,self).__init__() self.batchNorm = batchNorm self.conv1 = conv(self.batchNorm, 6, 64, kernel_size=7, stride=1) self.conv2 = conv(self.batchNorm, 64, 128, kernel_size=5, stride=1) self.conv3 = conv(self.batchNorm, 128, 256, kernel_size=5, stride=2) self.conv3_1 = conv(self.batchNorm, 256, 256) self.conv4 = conv(self.batchNorm, 256, 512, stride=2) self.conv4_1 = conv(self.batchNorm, 512, 512) self.conv5 = conv(self.batchNorm, 512, 512, stride=2) self.conv5_1 = conv(self.batchNorm, 512, 512) self.conv6 = conv(self.batchNorm, 512, 1024, stride=2) self.conv6_1 = conv(self.batchNorm,1024, 1024) self.deconv5 = deconv(1024,512) self.deconv4 = deconv(1026,256) self.deconv3 = deconv(770,128) self.deconv2 = deconv(386,64) self.predict_flow6 = predict_flow(1024) self.predict_flow5 = predict_flow(1026) self.predict_flow4 = predict_flow(770) self.predict_flow3 = predict_flow(386) self.predict_flow2 = predict_flow(194) self.upsampled_flow6_to_5 = nn.ConvTranspose2d(2, 2, 4, 2, 1, bias=False) self.upsampled_flow5_to_4 = nn.ConvTranspose2d(2, 2, 4, 2, 1, bias=False) self.upsampled_flow4_to_3 = nn.ConvTranspose2d(2, 2, 4, 2, 1, bias=False) self.upsampled_flow3_to_2 = nn.ConvTranspose2d(2, 2, 4, 2, 1, bias=False)
这段代码是一个名为StrainNetF的神经网络模型的定义。这个模型是基于PyTorch的nn.Module类构建的。
模型的构造函数__init__()接受一个布尔值batchNorm,用于指定是否应用批量归一化。模型的结构如下:
- conv1是一个卷积层,输入通道数为6,输出通道数为64,卷积核大小为7x7,步长为1。
- conv2是一个卷积层,输入通道数为64,输出通道数为128,卷积核大小为5x5,步长为1。
- conv3是一个卷积层,输入通道数为128,输出通道数为256,卷积核大小为5x5,步长为2。
- conv3_1是一个卷积层,输入通道数为256,输出通道数为256。
- conv4是一个卷积层,输入通道数为256,输出通道数为512,步长为2。
- conv4_1是一个卷积层,输入通道数为512,输出通道数为512。
- conv5是一个卷积层,输入通道数为512,输出通道数为512,步长为2。
- conv5_1是一个卷积层,输入通道数为512,输出通道数为512。
- conv6是一个卷积层,输入通道数为512,输出通道数为1024,步长为2。
- conv6_1是一个卷积层,输入通道数为1024,输出通道数为1024。
接下来,模型定义了几个反卷积层(deconv)用于上采样。这些层的输出通道数分别为512、256、128和64。
模型还定义了几个预测流的层(predict_flow),用于生成光流的预测结果。这些层的输出通道数都为2。
最后,模型定义了几个转置卷积层(upsampled_flow)用于将光流上采样到更高的分辨率。
这个模型的具体用途和背景信息没有提供,所以无法给出更详细的解释。如果有任何关于模型的问题,请继续提问。
这段代码怎么拆分成单独的层class resnet50_Decoder(nn.Module): def __init__(self, inplanes, bn_momentum=0.1): super(resnet50_Decoder, self).__init__() self.bn_momentum = bn_momentum self.inplanes = inplanes self.deconv_with_bias = False #----------------------------------------------------------# # 16,16,2048 -> 32,32,256 -> 64,64,128 -> 128,128,64 # 利用ConvTranspose2d进行上采样。 # 每次特征层的宽高变为原来的两倍。 #----------------------------------------------------------# self.deconv_layers = self._make_deconv_layer( num_layers=3, num_filters=[256, 128, 64], num_kernels=[4, 4, 4], ) def _make_deconv_layer(self, num_layers, num_filters, num_kernels): layers = [] for i in range(num_layers): kernel = num_kernels[i] planes = num_filters[i] layers.append( nn.ConvTranspose2d( in_channels=self.inplanes, out_channels=planes, kernel_size=kernel, stride=2, padding=1, output_padding=0, bias=self.deconv_with_bias)) layers.append(nn.BatchNorm2d(planes, momentum=self.bn_momentum)) layers.append(nn.ReLU(inplace=True)) self.inplanes = planes return nn.Sequential(*layers) def forward(self, x): return self.deconv_layers(x)
可以将这段代码拆分成以下两个类:
1. DeconvLayer(nn.Module):此类用于实现ConvTranspose2d、BatchNorm2d和ReLU激活函数的组合,即一层上采样层。其构造函数需要传入in_channels、out_channels、kernel_size、stride、padding、output_padding和bn_momentum等参数。
2. ResNet50Decoder(nn.Module):此类用于实现ResNet50的解码器部分,即利用DeconvLayer对ResNet50的特征图进行上采样,得到最终的输出结果。其构造函数需要传入inplanes和bn_momentum等参数。
以下是代码示例:
```python
class DeconvLayer(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, stride, padding, output_padding, bn_momentum=0.1, bias=False):
super(DeconvLayer, self).__init__()
self.conv_transpose = nn.ConvTranspose2d(in_channels, out_channels, kernel_size, stride, padding, output_padding, bias=bias)
self.bn = nn.BatchNorm2d(out_channels, momentum=bn_momentum)
self.relu = nn.ReLU(inplace=True)
def forward(self, x):
x = self.conv_transpose(x)
x = self.bn(x)
x = self.relu(x)
return x
class ResNet50Decoder(nn.Module):
def __init__(self, inplanes, bn_momentum=0.1):
super(ResNet50Decoder, self).__init__()
self.bn_momentum = bn_momentum
self.inplanes = inplanes
self.deconv_with_bias = False
self.deconv_layers = self._make_deconv_layer(
num_layers=3,
num_filters=[256, 128, 64],
num_kernels=[4, 4, 4],
)
def _make_deconv_layer(self, num_layers, num_filters, num_kernels):
layers = []
for i in range(num_layers):
kernel = num_kernels[i]
planes = num_filters[i]
layers.append(DeconvLayer(
in_channels=self.inplanes,
out_channels=planes,
kernel_size=kernel,
stride=2,
padding=1,
output_padding=0,
bn_momentum=self.bn_momentum,
bias=self.deconv_with_bias
))
self.inplanes = planes
return nn.Sequential(*layers)
def forward(self, x):
return self.deconv_layers(x)
```
阅读全文
相关推荐
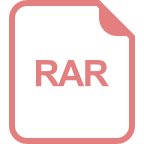
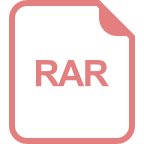
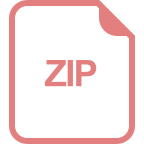
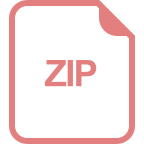
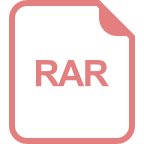
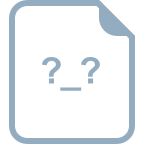
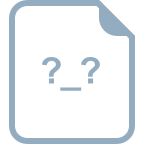
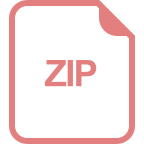




