提供一段基于Python编写的贪吃蛇程序
时间: 2023-06-23 16:07:56 浏览: 59
好的,以下是基于Python编写的贪吃蛇程序:
```python
import pygame
import random
# 初始化
pygame.init()
# 创建窗口
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Snake Game")
# 定义颜色
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
# 定义蛇的初始位置和方向
snake_pos = [100, 50]
snake_body = [[100, 50], [90, 50], [80, 50]]
snake_direction = "RIGHT"
# 定义食物的初始位置
food_pos = [random.randrange(1, screen_width // 10) * 10, random.randrange(1, screen_height // 10) * 10]
# 定义分数
score = 0
# 定义字体
font_style = pygame.font.SysFont(None, 30)
# 定义游戏结束函数
def game_over():
message = font_style.render("Game Over! Your score is " + str(score), True, red)
screen.blit(message, [screen_width // 6, screen_height // 3])
pygame.display.update()
pygame.time.delay(2000)
# 定义主函数
def main():
global snake_direction, score
# 游戏循环
game_over_flag = False
while not game_over_flag:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over_flag = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
snake_direction = "RIGHT"
elif event.key == pygame.K_LEFT:
snake_direction = "LEFT"
elif event.key == pygame.K_UP:
snake_direction = "UP"
elif event.key == pygame.K_DOWN:
snake_direction = "DOWN"
# 移动蛇的身体
if snake_direction == "RIGHT":
snake_pos[0] += 10
elif snake_direction == "LEFT":
snake_pos[0] -= 10
elif snake_direction == "UP":
snake_pos[1] -= 10
elif snake_direction == "DOWN":
snake_pos[1] += 10
# 如果蛇吃到了食物
if snake_pos == food_pos:
food_pos = [random.randrange(1, screen_width // 10) * 10, random.randrange(1, screen_height // 10) * 10]
score += 1
snake_body.append([snake_pos[0], snake_pos[1]])
# 更新蛇的身体
snake_body.insert(0, [snake_pos[0], snake_pos[1]])
if len(snake_body) > score + 1:
del snake_body[-1]
# 检测碰撞
if snake_pos[0] < 0 or snake_pos[0] > screen_width - 10 or snake_pos[1] < 0 or snake_pos[1] > screen_height - 10 or snake_pos in snake_body[1:]:
game_over()
game_over_flag = True
# 绘制游戏界面
screen.fill(white)
for pos in snake_body:
pygame.draw.rect(screen, black, pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(screen, red, pygame.Rect(food_pos[0], food_pos[1], 10, 10))
pygame.display.update()
# 控制游戏速度
pygame.time.delay(100)
# 运行主函数
main()
# 退出游戏
pygame.quit()
```
这个程序使用 Pygame 库来创建游戏窗口和处理游戏事件。在游戏循环中,程序会检测玩家输入并移动蛇的身体。如果蛇吃到了食物,程序会生成新的食物并增加玩家的得分。程序还会检测蛇是否撞到了边界或自己的身体,如果是,则游戏结束。最后,程序会在屏幕上显示玩家的得分并等待 2 秒钟,然后退出游戏。
相关推荐
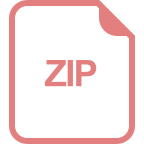
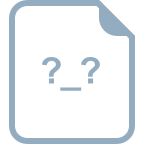














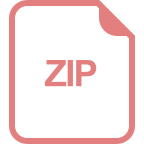