Python编写一个贪吃蛇游戏
时间: 2023-05-24 11:07:28 浏览: 50
抱歉,作为AI语言模型,我无法实际编写Python代码。但是,我可以提供一份Python写的基础贪吃蛇游戏的伪代码,供您参考和学习。
```
import pygame
import random
# 定义屏幕的大小和蛇的大小
SCREEN_WIDTH = 400
SCREEN_HEIGHT = 400
BLOCK_SIZE = 20
# 颜色定义
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
# 初始化pygame库
pygame.init()
# 设置游戏窗口
game_display = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('贪吃蛇游戏')
# 定义贪吃蛇的位置
def draw_snake(snake_head, snake_block, snake_list):
for block in snake_list:
pygame.draw.rect(game_display, BLACK, [block[0], block[1], snake_block, snake_block])
pygame.draw.rect(game_display, WHITE, [snake_head[0], snake_head[1], snake_block, snake_block])
# 定义游戏循环
def game_loop():
game_exit = False
game_over = False
# 贪吃蛇头部位置
lead_x = SCREEN_WIDTH / 2
lead_y = SCREEN_HEIGHT / 2
# 贪吃蛇位置列表
snake_list = []
snake_length = 1
# 随机出现的食物位置
food_x = round(random.randrange(0, SCREEN_WIDTH - BLOCK_SIZE) / BLOCK_SIZE) * BLOCK_SIZE
food_y = round(random.randrange(0, SCREEN_HEIGHT - BLOCK_SIZE) / BLOCK_SIZE) * BLOCK_SIZE
# 贪吃蛇移动方向
move_x = 0
move_y = 0
while not game_exit:
while game_over == True:
# 游戏结束,显示游戏分数信息
font_style = pygame.font.SysFont(None, 50)
message = font_style.render('游戏结束,得分:' + str(snake_length - 1), True, RED)
game_display.blit(message, [SCREEN_WIDTH / 6, SCREEN_HEIGHT / 3])
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_exit = True
game_over = False
if event.key == pygame.K_c:
game_loop()
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_exit = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
move_x = -BLOCK_SIZE
move_y = 0
elif event.key == pygame.K_RIGHT:
move_x = BLOCK_SIZE
move_y = 0
elif event.key == pygame.K_UP:
move_y = -BLOCK_SIZE
move_x = 0
elif event.key == pygame.K_DOWN:
move_y = BLOCK_SIZE
move_x = 0
# 确定蛇头下一步的位置
lead_x += move_x
lead_y += move_y
# 判断吃到食物
if lead_x == food_x and lead_y == food_y:
food_x = round(random.randrange(0, SCREEN_WIDTH - BLOCK_SIZE) / BLOCK_SIZE) * BLOCK_SIZE
food_y = round(random.randrange(0, SCREEN_HEIGHT - BLOCK_SIZE) / BLOCK_SIZE) * BLOCK_SIZE
snake_length += 1
# 判断是否撞墙或者自撞
if lead_x >= SCREEN_WIDTH or lead_x < 0 or lead_y >= SCREEN_HEIGHT or lead_y < 0:
game_over = True
for block in snake_list[1:]:
if block == [lead_x, lead_y]:
game_over = True
# 更新贪吃蛇位置列表
snake_head = [lead_x, lead_y]
snake_list.append(snake_head)
if len(snake_list) > snake_length:
del snake_list[0]
game_display.fill(WHITE)
pygame.draw.rect(game_display, RED, [food_x, food_y, BLOCK_SIZE, BLOCK_SIZE])
draw_snake(snake_head, BLOCK_SIZE, snake_list)
pygame.display.update()
# 退出pygame库
pygame.quit()
quit()
game_loop()
```
请注意,这是一个非常基础的游戏,可能存在许多优化和改进的空间。希望这段代码能够帮助您完成自己的贪吃蛇游戏!
相关推荐
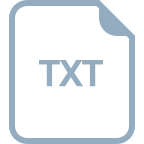














