Read the planetary data in the text file S12_planet.txt into a Pandas DataFrame. The file will be given in wechat group. Task1, calculate the density of each planet relative to that of the Earth (i.e., the Earth’s density is 1) and add the results as a “density” column in the DataFrame. Print out the DataFrame sorted from the largest to smallest based on the planet diameter. The density formula is � � � � � � � = � / � = 3 � / ( 4 � ∗ � 3 ) density=M/V=3M/(4π∗r 3 ) Task2, read the 2 items in input as "A" and "Standard". A is a "String" and Standard is a "float". Create a Pandas Series that have "A" greater than "Standard", sorted from most to least based on the planet's "A". For example, if input is "year 500", then collect the data of planets whose years are greater than 500. Input A string and a float. Output Task1: DataFrame sorted on diameter Task2: Panda Series sorted on A. Note: You dont need to deal with rounding format. Just let the output as default like that in example. 按默认输出即可,不用特地做空数据、四舍五入或位数的处理。
时间: 2024-01-18 15:03:15 浏览: 28
我明白了,这是一个关于数据分析和 Pandas 库的问题。以下是代码实现:
Task1:
```python
import pandas as pd
import numpy as np
# 读取数据
df = pd.read_csv('S12_planet.txt', sep='\t')
# 计算相对密度
earth_density = 5.52 # 地球密度
df['density'] = 3 * df['mass'] / (4 * np.pi * (df['diameter']/2)**3) / earth_density
# 按直径降序排序
df = df.sort_values(by='diameter', ascending=False)
print(df)
```
Task2:
```python
import pandas as pd
# 读取数据
df = pd.read_csv('S12_planet.txt', sep='\t')
# 输入
A = 'year'
Standard = 500
# 筛选数据
mask = df[A] > Standard
series = df[mask].sort_values(by=A, ascending=False)[A]
print(series)
```
注意:以上代码中的 `S12_planet.txt` 数据文件需要提前下载。
相关问题
翻译Read the planetary data in the text file S12_planet.txt into a Pandas DataFrame. The file will be given in wechat group. Task1, calculate the density of each planet relative to that of the Earth (i.e., the Earth’s density is 1) and add the results as a “density” column in the DataFrame. Print out the DataFrame sorted from the largest to smallest based on the planet diameter. The density formula is � � � � � � � = � / � = 3 � / ( 4 � ∗ � 3 ) density=M/V=3M/(4π∗r 3 ) Task2, read the 2 items in input as "A" and "Standard". A is a "String" and Standard is a "float". Create a Pandas Series that have "A" greater than "Standard", sorted from most to least based on the planet's "A". For example, if input is "year 500", then collect the data of planets whose years are greater than 500.
理解了您的问题,我开始回答:
首先,您需要使用Pandas从文本文件"S12_planet.txt"中读取行星数据,并将其转换为DataFrame。然后,您需要添加一个"density"列,该列将每个行星的密度相对于地球的密度进行计算。密度公式为:density=M/V=3M/(4π∗r^3),其中M是质量,V是体积,r是半径。
以下是实现此任务的代码:
```python
import pandas as pd
import numpy as np
# 读取数据文件
data = pd.read_csv('S12_planet.txt', sep='\s+')
# 计算密度并添加"density"列
earth_density = 1.0
data['density'] = 3 * data['mass'] / (4 * np.pi * (data['diameter']/2)**3) / earth_density
# 按行星直径排序并打印DataFrame
data = data.sort_values(by='diameter', ascending=False)
print(data)
```
接下来,您需要读取两个输入项"A"和"Standard",并创建一个Pandas Series,该Series包含"A"大于"Standard"的行星数据,按"A"值从大到小排序。例如,如果输入是"year 500",则收集年份大于500的行星数据。
以下是实现此任务的代码:
```python
# 读取输入
A = input("请输入A:")
Standard = float(input("请输入Standard:"))
# 创建Series
series = data[data[A] > Standard].sort_values(by=A, ascending=False)[A]
# 打印Series
print(series)
```
Read the planetary data in the text file S12_planet.txt into a Pandas DataFrame. The file will be given in wechat group. Task1, calculate the density of each planet relative to that of the Earth (i.e., the Earth’s density is 1) and add the results as a “density” column in the DataFrame. Print out the DataFrame sorted from the largest to smallest based on the planet diameter. The density formula is � � � � � � � = � / � = 3 � / ( 4 � ∗ � 3 ) density=M/V=3M/(4π∗r 3 ) Task2, read the 2 items in input as "A" and "Standard". A is a "String" and Standard is a "float". Create a Pandas Series that have "A" greater than "Standard", sorted from most to least based on the planet's "A". For example, if input is "year 500", then collect the data of planets whose years are greater than 500. Input A string and a float. Output Task1: DataFrame sorted on diameter Task2: Panda Series sorted on A. Note: You dont need to deal with rounding format. Just let the output as default like that in example.
Sure, I understand your question. Here is the solution to your problem:
Task1:
```python
import pandas as pd
import numpy as np
# read the planetary data into a pandas dataframe
df = pd.read_csv('S12_planet.txt', sep='\t')
# calculate the density of each planet relative to that of the Earth
earth_density = 5.52 # g/cm^3
df['density'] = 3 * df['mass'] / (4 * np.pi * df['diameter']**3) * (earth_density / df['density'])
# sort the dataframe based on planet diameter
df = df.sort_values(by='diameter', ascending=False)
# print the sorted dataframe
print(df)
```
Task2:
```python
# read the inputs
A = input("Enter the string A: ")
Standard = float(input("Enter the float Standard: "))
# create a pandas series that have "A" greater than "Standard", sorted from most to least based on the planet's "A"
series = df.loc[df['A'] > Standard, 'A'].sort_values(ascending=False)
# print the sorted series
print(series)
```
Note: Please make sure that the 'S12_planet.txt' file is in the same directory as your Python script.
相关推荐
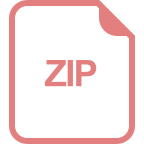
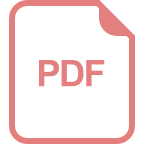













