如何使用pthread_create?
时间: 2023-09-12 14:08:50 浏览: 81
pthread_create是一个用于创建一个新线程的函数,其原型为:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
其中,参数解释如下:
- thread:新线程的标识符,类型为pthread_t。
- attr:新线程的属性,可以为NULL,表示使用默认属性。
- start_routine:新线程的函数指针,新线程将从此函数开始执行。
- arg:新线程的参数,可以为NULL,表示不传递参数。
使用pthread_create的步骤如下:
1. 定义一个函数作为新线程的入口函数,函数的参数为void *类型,返回值为void *类型。
2. 在主线程中调用pthread_create函数创建新线程,传递入口函数的指针和参数。
3. 在入口函数中进行新线程的具体操作,例如计算、输出等。
4. 在入口函数中使用pthread_exit函数退出线程。
下面是一个简单的例子:
```c
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg) {
int i;
for (i = 0; i < 5; ++i) {
printf("Thread: %d\n", i);
}
pthread_exit(NULL);
}
int main() {
pthread_t thread;
if (pthread_create(&thread, NULL, thread_func, NULL) != 0) {
printf("Error: Failed to create thread.\n");
return 1;
}
int i;
for (i = 0; i < 5; ++i) {
printf("Main: %d\n", i);
}
pthread_join(thread, NULL);
return 0;
}
```
该示例中,定义了一个函数thread_func作为新线程的入口函数,该函数循环输出5次“Thread: i”;在主线程中调用pthread_create函数创建新线程,传递入口函数的指针和参数;在主线程中循环输出5次“Main: i”;使用pthread_join函数等待新线程结束。运行该程序,可以看到新线程和主线程交替输出。
阅读全文
相关推荐
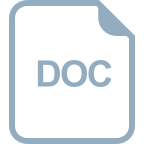
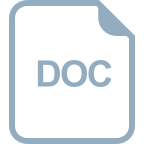
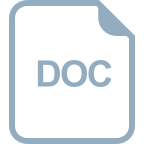















