opencv 测量卡尺
时间: 2023-09-29 09:10:49 浏览: 151
OpenCV 提供了测量图像中线段长度和角度的函数,可以用来实现测量卡尺的功能。下面是一个基本的流程:
1. 读取图像并转换为灰度图像。
2. 使用 Canny 边缘检测算法检测图像边缘。
3. 使用霍夫直线变换检测图像中的直线。
4. 对检测到的直线进行筛选,只保留符合条件的直线。
5. 计算直线的交点,这些交点就是卡尺的两个端点。
6. 计算卡尺的长度和角度。
以下是一个简单的代码示例:
```python
import cv2
# 读取图像并转换为灰度图像
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 检测直线
lines = cv2.HoughLines(edges, 1, cv2.cv2.PI/180, 200)
# 筛选直线
for line in lines:
rho, theta = line[0]
if abs(theta - cv2.cv2.PI/2) < 0.1 or abs(theta) < 0.1:
continue
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * a)
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * a)
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 计算交点
points = []
for i in range(len(lines)):
for j in range(i+1, len(lines)):
rho1, theta1 = lines[i][0]
rho2, theta2 = lines[j][0]
a1 = np.cos(theta1)
b1 = np.sin(theta1)
a2 = np.cos(theta2)
b2 = np.sin(theta2)
d = a1 * b2 - a2 * b1
if abs(d) < 1e-6:
continue
x = int((b2 * rho1 - b1 * rho2) / d)
y = int((-a2 * rho1 + a1 * rho2) / d)
points.append((x, y))
# 计算长度和角度
if len(points) == 2:
x1, y1 = points[0]
x2, y2 = points[1]
length = np.sqrt((x1-x2)**2 + (y1-y2)**2)
angle = np.arctan2(y2-y1, x2-x1) * 180 / np.pi
print('length:', length, 'angle:', angle)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,这只是一个基本的示例,实际应用中可能需要根据具体需求进行修改。
阅读全文
相关推荐
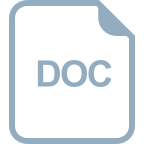
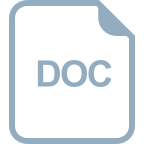
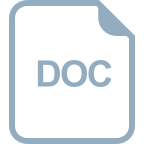






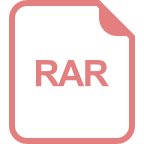







