用51单片机与openmv写一个数字识别
时间: 2024-05-05 18:21:00 浏览: 10
要实现数字识别,可以将OpenMV作为图像处理模块,使用51单片机进行控制和通信。
下面是一个简单的流程:
1. 用OpenMV拍摄一张包含数字的图片。
2. 在OpenMV上运行图像处理算法,将数字从图像中提取出来并进行识别。
3. OpenMV将识别结果通过串口发送给51单片机。
4. 51单片机接收到数据后进行相应的处理,例如控制LCD显示识别结果。
以下是一个示例代码:
OpenMV代码:
```python
import sensor, image, time
from pyb import UART
# 初始化串口通信
uart = UART(3, 9600)
# 设置图像传感器分辨率
sensor.reset()
sensor.set_pixformat(sensor.GRAYSCALE)
sensor.set_framesize(sensor.QVGA)
# 设置数字识别算法参数
thresholds = [(0, 64)]
roi = (100, 50, 60, 60)
digits = "0123456789"
# 开始捕捉图像
while(True):
img = sensor.snapshot()
# 对ROI内的图像进行二值化处理
img.binary(thresholds)
# 找到图像中所有的数字
for r in img.find_rects(thresholds=thresholds, roi=roi):
# 从ROI中提取数字图像
digit_img = img.crop(r)
# 缩放图像到固定大小
digit_img = digit_img.resize(20, 20)
# 将图像转换为一维数组
digit_array = digit_img.to_bytes()
# 将数字数组转换为字符串
digit_str = ""
for pixel in digit_array:
digit_str += digits[pixel // 26]
# 将数字字符串发送给51单片机
uart.write(digit_str)
```
51单片机代码:
```c
#include <reg52.h>
#include <stdio.h>
// 定义LCD引脚
sbit RS = P2^0;
sbit RW = P2^1;
sbit EN = P2^2;
sbit D4 = P0^4;
sbit D5 = P0^5;
sbit D6 = P0^6;
sbit D7 = P0^7;
// 定义数字接收缓冲区
char digit_buffer[5] = {0};
// LCD初始化函数
void init_lcd()
{
delay(20);
lcd_cmd(0x02);
lcd_cmd(0x28);
lcd_cmd(0x0C);
lcd_cmd(0x01);
delay(20);
}
// LCD写命令函数
void lcd_cmd(char cmd)
{
RS = 0;
RW = 0;
EN = 1;
P0 = cmd;
delay(1);
EN = 0;
}
// LCD写数据函数
void lcd_data(char dat)
{
RS = 1;
RW = 0;
EN = 1;
P0 = dat;
delay(1);
EN = 0;
}
// LCD清屏函数
void lcd_clear()
{
lcd_cmd(0x01);
}
// LCD设置光标位置函数
void lcd_set_cursor(char row, char col)
{
char pos = 0x80;
if(row == 1)
pos += 0x40;
pos += col;
lcd_cmd(pos);
}
// 延时函数
void delay(unsigned int t)
{
unsigned int i, j;
for(i = 0; i < t; i++)
for(j = 0; j < 120; j++);
}
// 串口接收中断函数
void serial_isr() interrupt 4
{
static int digit_index = 0;
char digit = SBUF;
if(digit >= '0' && digit <= '9')
{
digit_buffer[digit_index++] = digit;
if(digit_index >= 5)
{
digit_buffer[5] = '\0';
digit_index = 0;
lcd_set_cursor(1, 0);
lcd_data(digit_buffer[0]);
lcd_data(digit_buffer[1]);
lcd_data(digit_buffer[2]);
lcd_data(digit_buffer[3]);
lcd_data(digit_buffer[4]);
}
}
}
// 主函数
void main()
{
ES = 1; // 开启串口中断
EA = 1; // 开启总中断
init_lcd(); // 初始化LCD
lcd_clear(); // 清屏
while(1);
}
```
在上述代码中,串口接收中断函数将接收到的数字存储在缓冲区中,并在缓冲区填满后将数字显示在LCD屏幕上。您可以根据需要修改代码以满足您的应用需求。
相关推荐
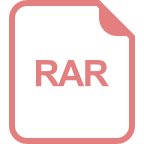














