弹球游戏代码
时间: 2023-07-03 19:12:17 浏览: 63
以下是一个简单的Python弹球游戏的代码示例,仅供参考:
```python
import pygame
from pygame.locals import *
import random
# 初始化Pygame
pygame.init()
# 定义游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
# 定义弹珠和挡板的大小和颜色
BALL_RADIUS = 10
BALL_COLOR = (255, 255, 255)
PADDLE_WIDTH = 80
PADDLE_HEIGHT = 10
PADDLE_COLOR = (255, 255, 255)
# 定义砖块的大小和颜色
BRICK_WIDTH = 60
BRICK_HEIGHT = 20
BRICK_COLOR = (255, 255, 255)
# 定义游戏背景颜色
BG_COLOR = (0, 0, 0)
# 设置游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('弹珠小游戏')
# 定义全局变量
score = 0
lives = 3
# 定义弹珠和挡板的初始位置和速度
ball_x = WINDOW_WIDTH // 2
ball_y = WINDOW_HEIGHT // 2
ball_dx = 5
ball_dy = 5
paddle_x = (WINDOW_WIDTH - PADDLE_WIDTH) // 2
paddle_y = WINDOW_HEIGHT - PADDLE_HEIGHT
# 定义砖块的位置和数量
brick_rows = 5
brick_cols = 8
bricks = []
for row in range(brick_rows):
brick_row = []
for col in range(brick_cols):
brick_row.append(1)
bricks.append(brick_row)
# 定义字体
font = pygame.font.SysFont('Arial', 16)
# 定义游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == MOUSEMOTION:
paddle_x = event.pos[0]
# 绘制游戏界面
window.fill(BG_COLOR)
pygame.draw.circle(window, BALL_COLOR, (ball_x, ball_y), BALL_RADIUS)
pygame.draw.rect(window, PADDLE_COLOR, (paddle_x, paddle_y, PADDLE_WIDTH, PADDLE_HEIGHT))
for row in range(brick_rows):
for col in range(brick_cols):
if bricks[row][col] == 1:
brick_x = col * BRICK_WIDTH
brick_y = row * BRICK_HEIGHT
pygame.draw.rect(window, BRICK_COLOR, (brick_x, brick_y, BRICK_WIDTH, BRICK_HEIGHT))
# 更新弹珠位置
ball_x += ball_dx
ball_y += ball_dy
# 碰撞检测
if ball_x <= BALL_RADIUS or ball_x >= WINDOW_WIDTH - BALL_RADIUS:
ball_dx = -ball_dx
if ball_y <= BALL_RADIUS:
ball_dy = -ball_dy
elif ball_y >= WINDOW_HEIGHT - BALL_RADIUS - PADDLE_HEIGHT:
if ball_x >= paddle_x and ball_x <= paddle_x + PADDLE_WIDTH:
ball_dy = -ball_dy
score += 10
else:
lives -= 1
if lives == 0:
pygame.quit()
sys.exit()
ball_x = WINDOW_WIDTH // 2
ball_y = WINDOW_HEIGHT // 2
ball_dx = random.choice([-5, 5])
ball_dy = 5
# 砖块碰撞检测
row = ball_y // BRICK_HEIGHT
col = ball_x // BRICK_WIDTH
if row < brick_rows and col < brick_cols and bricks[row][col] == 1:
bricks[row][col] = 0
ball_dy = -ball_dy
score += 50
# 显示得分和生命值
score_text = font.render('得分:' + str(score), True, (255, 255, 255))
lives_text = font.render('生命:' + str(lives), True, (255, 255, 255))
window.blit(score_text, (10, 10))
window.blit(lives_text, (WINDOW_WIDTH - lives_text.get_width() - 10, 10))
# 更新窗口
pygame.display.update()
# 控制游戏帧率
pygame.time.Clock().tick(60)
```
以上是一个简单的Python弹球游戏的代码示例,仅供参考。实际开发中,还需进一步完善和优化代码。
相关推荐
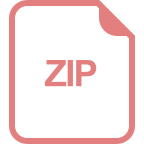














