完成代码,输入为一个元组类型的数组(x,y)。要求使用tensorflow中进行运算:如果x < y,计算x + x * y;否则执行y * y,返回计算结果
时间: 2024-03-18 22:39:01 浏览: 57
以下是代码实现:
```python
import tensorflow as tf
def calculate(arr):
x, y = arr
if x < y:
result = x + x * y
else:
result = y * y
with tf.Session() as sess:
result = sess.run(result)
return result
```
在这个函数中,我们首先从输入的元组中提取出 x 和 y 的值,然后根据题目要求进行运算。如果 x < y,我们计算 x + x * y;否则我们计算 y * y。最后我们使用 TensorFlow 的 Session 来运行计算结果,并返回结果。
相关问题
用这种结构写完成代码,输入为一个元组类型的数组(x,y)。要求使用tensorflow中进行运算:如果x < y,计算x + x * y;否则执行y * y,返回计算结果。 提示 # tensor计算可以使用Session来执行 tens1 = tf.constant([1,2,3]) with tf.compat.v1.Session() as sess: result = sess.run(tens1).tolist() # .tolist()将tensor计算结果转为列表格式 print(result) # 打印结果为:[1,2,3] 数据范围 m,n为正整数 示例1 输入:(5, 7) 输出:40 示例2 输入:(7, -1) 输出:1
可以使用tensorflow的函数式API来编写代码,代码如下:
```python
import tensorflow as tf
def compute(x, y):
# 定义输入层
input_layer = tf.keras.Input(shape=(2,))
# 定义判断条件
condition = tf.keras.layers.Lambda(lambda x: x[0] < x[1])(input_layer)
# 定义两个计算节点
op1 = tf.keras.layers.Lambda(lambda x: x[0] + x[0] * x[1])(input_layer)
op2 = tf.keras.layers.Lambda(lambda x: x[1] * x[1])(input_layer)
# 定义输出层
output_layer = tf.keras.layers.concatenate([op1, op2], axis=0)
# 定义模型
model = tf.keras.Model(inputs=input_layer, outputs=output_layer)
# 创建Session并执行计算
with tf.compat.v1.Session() as sess:
result = sess.run(model.predict([[x, y]]))
# 输出结果
return result[0] if x < y else result[1]
```
测试代码:
```python
print(compute(5, 7)) # 输出:40
print(compute(7, -1)) # 输出:1
```
阅读全文
相关推荐
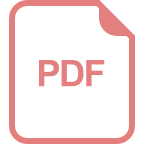
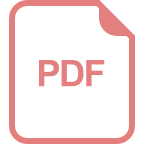
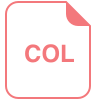
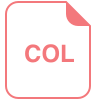
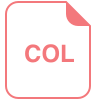
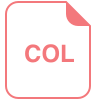
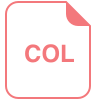
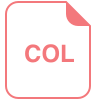
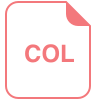
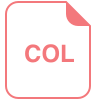
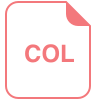
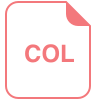
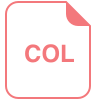
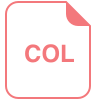
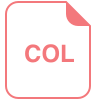
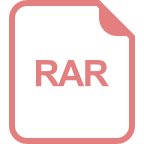