【TensorFlow张量操作全攻略】:从入门到精通
发布时间: 2024-09-30 09:07:41 阅读量: 4 订阅数: 7 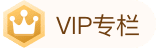
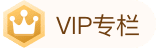
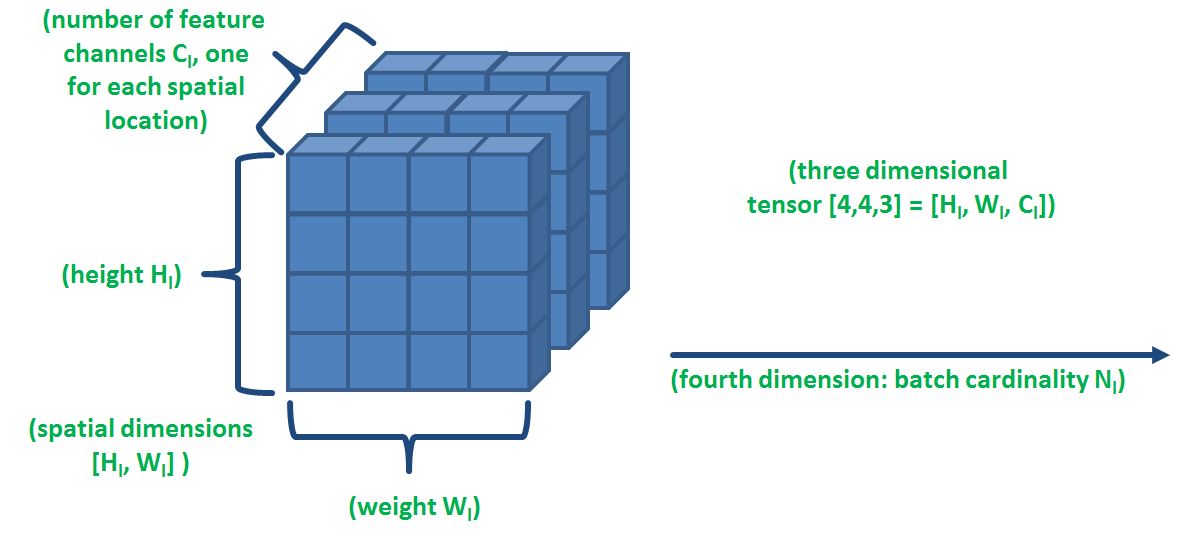
# 1. TensorFlow基础概念和安装
TensorFlow 是一个开源的机器学习框架,最初由 Google 的 Brain Team 开发。它的名字来自张量(Tensor)的概念,这是一维或更高维度的矩阵。在 TensorFlow 中,数据以张量的形式流动,从输入节点(Source nodes)到输出节点(Sink nodes),过程中经过一系列计算操作(Operations),形成了一个计算图(Computation Graph)。计算图是 TensorFlow 运行的核心概念,它定义了数据的流动和变换过程。
## TensorFlow 的安装
安装 TensorFlow 相对简单,推荐使用 Python 的包管理工具 `pip`。以下是安装 TensorFlow 的步骤:
1. 打开终端或命令提示符。
2. 输入以下命令安装 CPU 版本的 TensorFlow:
```bash
pip install tensorflow
```
或者安装 GPU 版本,确保已经安装了 CUDA 和 cuDNN:
```bash
pip install tensorflow-gpu
```
3. 安装完成后,可以使用以下 Python 代码测试安装是否成功:
```python
import tensorflow as tf
hello = tf.constant('Hello, TensorFlow!')
print(tf.reduce_sum(hello))
```
如果输出类似于 "b'Hello, TensorFlow!'",则表示 TensorFlow 已经安装成功。
对于 TensorFlow 的初学者来说,理解计算图的概念以及如何通过 Python API 进行安装和基础操作是进入深度学习世界的第一步。在此基础上,可以进一步学习如何操作张量以及构建和训练模型。
# 2. TensorFlow张量操作基础
## 2.1 张量的概念和创建
### 2.1.1 张量的定义和属性
张量是TensorFlow中的核心数据结构,可以简单理解为一个多维数组。在数学上,张量通常用来表示一个在多维空间中的几何对象。在TensorFlow中,张量用于存储所有的数据,所有的运算都是在张量上进行的。
张量具有以下基本属性:
- Rank:张量的维度,也就是张量的阶数,也可以理解为数组的轴数。
- Shape:张量的形状,是一个整数元组,定义了张量的每个维度的大小。
- Data type:张量的数据类型,如int32, float32等。
张量可以通过`tf.constant`或者`tf.Variable`等函数创建,其中`tf.constant`创建的是常量张量,而`tf.Variable`则可以创建一个可变的张量。
```python
import tensorflow as tf
# 创建一个常量张量
constant_tensor = tf.constant([[1, 2], [3, 4]])
print("Constant tensor:", constant_tensor)
# 创建一个变量张量
variable_tensor = tf.Variable([[5, 6], [7, 8]])
print("Variable tensor:", variable_tensor)
```
### 2.1.2 张量的初始化方法
TensorFlow提供了多种张量初始化的方法,可以根据需要创建具有特定值或者特定分布的张量。
- tf.zeros(shape, dtype=tf.float32, name=None)
- tf.ones(shape, dtype=tf.float32, name=None)
- tf.fill(dims, value, name=None)
- tf.random.normal(shape, mean=0.0, stddev=1.0, dtype=tf.float32, seed=None, name=None)
- tf.random.uniform(shape, minval=0, maxval=None, dtype=tf.float32, seed=None, name=None)
这些初始化函数都接受一个shape参数,定义了张量的形状。一些函数还可以接受数据类型dtype,用于指定创建张量的数据类型。使用这些函数可以方便地根据模型需求初始化参数。
```python
# 创建一个2x3的全零张量
zero_tensor = tf.zeros([2, 3])
print("Zero tensor:", zero_tensor)
# 创建一个2x3的全一张量
one_tensor = tf.ones([2, 3])
print("One tensor:", one_tensor)
# 创建一个2x3的填充张量,填充值为10
fill_tensor = tf.fill([2, 3], 10)
print("Fill tensor:", fill_tensor)
# 创建一个2x3的正态分布随机张量
normal_tensor = tf.random.normal([2, 3])
print("Normal distribution tensor:", normal_tensor)
# 创建一个2x3的均匀分布随机张量
uniform_tensor = tf.random.uniform([2, 3], minval=0, maxval=1)
print("Uniform distribution tensor:", uniform_tensor)
```
## 2.2 张量的基本运算
### 2.2.1 张量的加减乘除和矩阵运算
在TensorFlow中,张量的基本运算可以使用Python的运算符直接进行,如加法运算可以使用`+`,减法使用`-`,乘法使用`*`,除法使用`/`。TensorFlow也支持NumPy风格的运算符,如`tf.add()`, `tf.subtract()`, `tf.multiply()`, `tf.divide()`等。
矩阵运算在机器学习中尤为重要,TensorFlow提供了矩阵乘法的专用函数`tf.matmul()`。这个函数可以执行两个张量的矩阵乘法操作。
```python
import tensorflow as tf
# 创建两个矩阵张量
a = tf.constant([[1, 2], [3, 4]])
b = tf.constant([[5, 6], [7, 8]])
# 进行矩阵乘法
matmul_result = tf.matmul(a, b)
print("Matrix multiplication result:\n", matmul_result)
```
### 2.2.2 张量的广播机制
TensorFlow中的广播机制允许不同形状的张量进行运算。当两个张量形状不匹配时,TensorFlow会尝试将它们的形状对齐。在对齐的过程中,较小的张量的形状可以通过在前面补1的方式进行扩展,直到与较大张量的形状匹配。
广播规则如下:
1. 如果两个张量的形状在所有维度上都相同,则直接进行元素级运算。
2. 如果在任何维度上形状不相同,较小形状的维度将被1补充。
3. 如果某个维度的大小为1,而另一个维度大于1,则在较小维度上进行复制操作。
4. 如果形状不兼容,无法进行运算,会引发错误。
```python
import tensorflow as tf
# 创建两个形状不同的张量
a = tf.constant([[1, 2, 3], [4, 5, 6]])
b = tf.constant([1, 2, 3])
# 使用广播机制进行元素级乘法
broadcast_result = a * b
print("Broadcast result:\n", broadcast_result)
```
## 2.3 张量的数据类型和转换
### 2.3.1 TensorFlow支持的数据类型
TensorFlow支持多种数据类型,包括:
- tf.int8, tf.int16, tf.int32, tf.int64
- tf.uint8, tf.uint16, tf.uint32, tf.uint64
- tf.float16, tf.float32, ***
***plex64, ***plex128
每种数据类型都有其特定的应用场景,比如,用于存储图像数据时常用的数据类型是`tf.uint8`,用于计算时则常用`tf.float32`。
### 2.3.2 张量的数据类型转换方法
在TensorFlow中,可以通过`tf.cast`函数来转换张量的数据类型。在使用`tf.cast`时需要指定目标数据类型。
```python
import tensorflow as tf
# 创建一个整型张量
integer_tensor = tf.constant([[1, 2], [3, 4]])
# 将整型张量转换为浮点型张量
float_tensor = tf.cast(integer_tensor, tf.float32)
print("Float tensor:", float_tensor)
# 将浮点型张量转换为32位整型张量
int_tensor = tf.cast(float_tensor, tf.int32)
print("Int tensor:", int_tensor)
```
数据类型转换在处理不同类型数据时非常有用,尤其是在将张量传递给不同的操作时,这些操作可能需要特定的数据类型。例如,在深度学习模
0
0
相关推荐
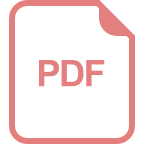
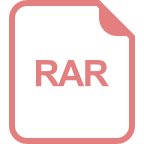
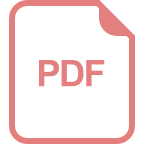
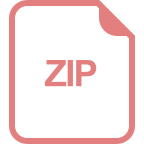
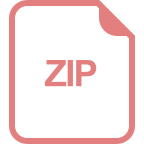
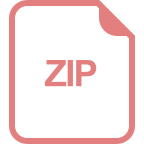
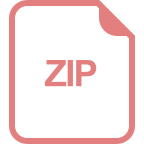
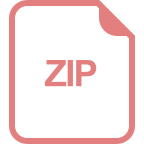
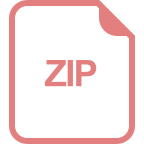