基于SSM框架的文件上传下载代码
时间: 2024-06-19 13:04:31 浏览: 17
基于SSM框架的文件上传下载代码,可以分为两部分:前端页面和后端代码。
前端页面:
1. 文件上传页面(upload.jsp)
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>文件上传</title>
</head>
<body>
<form method="post" action="fileUpload" enctype="multipart/form-data">
<input type="file" name="file"/>
<input type="submit" value="上传"/>
</form>
</body>
</html>
```
2. 文件下载页面(download.jsp)
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>文件下载</title>
</head>
<body>
<table>
<thead>
<tr>
<th>文件名</th>
<th>大小</th>
<th>下载</th>
</tr>
</thead>
<tbody>
<c:forEach items="${files}" var="file">
<tr>
<td>${file.name}</td>
<td>${file.size}</td>
<td><a href="fileDownload?fileName=${file.name}">下载</a></td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
后端代码:
1. 文件上传Controller(FileUploadController.java)
```
@Controller
public class FileUploadController {
@RequestMapping(value = "/fileUpload", method = RequestMethod.POST)
@ResponseBody
public String fileUpload(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return "请选择文件";
}
String fileName = file.getOriginalFilename();
String filePath = "C:\\upload\\";
File dest = new File(filePath + fileName);
try {
file.transferTo(dest);
return "文件上传成功";
} catch (IOException e) {
return "文件上传失败";
}
}
}
```
2. 文件下载Controller(FileDownloadController.java)
```
@Controller
public class FileDownloadController {
@RequestMapping(value = "/fileDownload")
public void fileDownload(HttpServletRequest request, HttpServletResponse response,
@RequestParam("fileName") String fileName) {
String filePath = "C:\\upload\\";
File file = new File(filePath + fileName);
if (file.exists()) {
response.setContentType("application/octet-stream");
response.setContentLength((int) file.length());
response.setHeader("Content-Disposition", "attachment;filename=" + fileName);
try {
FileInputStream in = new FileInputStream(file);
OutputStream out = response.getOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
in.close();
out.flush();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
相关推荐
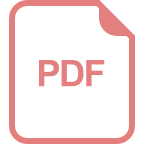
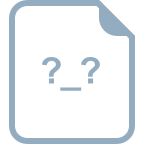
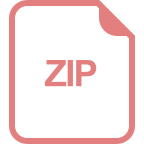
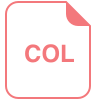
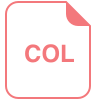
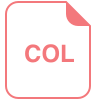
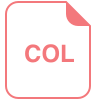
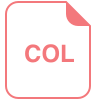









