axs = axs.flatten()
时间: 2023-09-24 09:07:29 浏览: 39
This code is used to flatten a multi-dimensional array into a one-dimensional array.
Here, `axs` is a multi-dimensional array of shape `(nrows, ncols)`. The `flatten()` function is called on `axs` to transform it into a one-dimensional array. The resulting one-dimensional array is then assigned back to `axs`.
This is commonly used in plotting multiple subplots using Matplotlib. The flattened array allows for easy indexing of each individual subplot.
相关问题
import numpy as np import matplotlib.pyplot as plt from scipy import signal t = np.linspace(0, 2 * np.pi, 128, endpoint=False) x = np.sin(2 * t) print(x) kernel1 = np.array([[-1, 0, 1], [-2, 0, 2], [-1, 0, 1]]) kernel2 = np.array([[1, 2, 1], [0, 0, 0], [-1, -2, -1]]) result1 = signal.convolve2d(x.reshape(1, -1), kernel1, mode='same') result2 = signal.convolve2d(x.reshape(1, -1), kernel2, mode='same') fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].imshow(kernel1) axs[1].set_title('Kernel 1') axs[2].imshow(kernel2) axs[2].set_title('Kernel 2') fig.tight_layout() fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].plot(t, result1.flatten()) axs[1].set_title('Result of convolution with kernel 1') axs[2].plot(t, result2.flatten()) axs[2].set_title('Result of convolution with kernel 2') fig.tight_layout() plt.show() # from scipy.signal import pool import numpy as np def pool(signal, window_size, mode='max'): if mode == 'max': return np.max(signal.reshape(-1, window_size), axis=1) elif mode == 'min': return np.min(signal.reshape(-1, window_size), axis=1) elif mode == 'mean': return np.mean(signal.reshape(-1, window_size), axis=1) else: raise ValueError("Invalid mode. Please choose 'max', 'min', or 'mean'.") # 对卷积结果进行最大池化 pool_size = 2 result1_pooled = pool(result1, pool_size, 'max') result2_pooled = pool(result2, pool_size, 'max') # 可视化结果 fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].plot(t, result1.flatten()) axs[1].set_title('Result of convolution with kernel 1') axs[2].plot(t[::2], result1_pooled.flatten()) axs[2].set_title('Result of max pooling after convolution with kernel 1') fig.tight_layout() plt.show()给这段代码添加全连接层
这段代码使用了卷积神经网络对一维信号进行特征提取和池化。要添加全连接层,可以在卷积层和池化层之后添加一个或多个全连接层,将池化层的输出展平为一维向量,并将其输入到全连接层中进行分类或回归。以下是一个简单的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv1D, MaxPooling1D, Flatten, Dense
# 生成数据
t = np.linspace(0, 2 * np.pi, 128, endpoint=False)
x = np.sin(2 * t)
# 定义卷积核
kernel1 = np.array([[-1, 0, 1], [-2, 0, 2], [-1, 0, 1]])
kernel2 = np.array([[1, 2, 1], [0, 0, 0], [-1, -2, -1]])
# 定义卷积神经网络
model = Sequential()
model.add(Conv1D(filters=16, kernel_size=3, activation='relu', input_shape=(128, 1)))
model.add(MaxPooling1D(pool_size=2))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
# 编译模型
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# 训练模型
x = x.reshape(-1, 128, 1)
model.fit(x, y, epochs=10, batch_size=16)
# 可视化结果
result1 = signal.convolve2d(x.reshape(1, -1), kernel1, mode='same')
result2 = signal.convolve2d(x.reshape(1, -1), kernel2, mode='same')
result1_pooled = pool(result1, pool_size, 'max')
result2_pooled = pool(result2, pool_size, 'max')
pred = model.predict(x)
fig, axs = plt.subplots(4, 1, figsize=(8, 8))
axs[0].plot(t, x.flatten())
axs[0].set_title('Original signal')
axs[1].plot(t, result1.flatten())
axs[1].set_title('Result of convolution with kernel 1')
axs[2].plot(t[::2], result1_pooled.flatten())
axs[2].set_title('Result of max pooling after convolution with kernel 1')
axs[3].plot(t, pred.flatten())
axs[3].set_title('Predictions')
fig.tight_layout()
plt.show()
```
这个示例代码中,我们定义了一个包含一个卷积层、一个池化层、两个全连接层的卷积神经网络,并使用二分类交叉熵作为损失函数进行训练。通过添加全连接层,我们可以将卷积层和池化层提取的特征进一步处理,从而提高模型的性能。
import pandas as pd import matplotlib.pyplot as plt file=pd.read_csv(r'D:\pythonProject\winequality-white.csv',delimiter=';') df = file def plot_hist(df1): feature_names = df1.columns[:-1] fig, axs = plt.subplots(nrows=3, ncols=4, figsize=(20, 15)) for i, feature_name in enumerate(feature_names): n, bins, patches = axs.flatten()[i].hist(df1[feature_name], bins=50, alpha=0.7,color='steelblue', edgecolor='black', linewidth=1.5) axs.flatten()[i].set_title(feature_name, fontsize=20) axs.flatten()[i].set_xlabel(feature_name, fontsize=15) axs.flatten()[i].set_ylabel('Count', fontsize=15) axs.flatten()[i].tick_params(axis='both', labelsize=12) for patch in patches: patch.set_linewidth(2) patch.set_edgecolor('black') plt.tight_layout() plt.savefig(r'D:\pythonProject\hist.png') plt.show() plot_hist(df)请详细地解释上述代码
这段代码实现了一个函数 `plot_hist`,其目的是绘制数据集中每个特征的直方图。具体实现步骤如下:
1. 导入了 pandas 和 matplotlib.pyplot 两个库。
2. 读取名为 "winequality-white.csv" 的文件,将其转换成 pandas 的 DataFrame 对象 `file`。
3. 将 `file` 复制到名为 `df` 的新变量中。
4. 定义了函数 `plot_hist(df1)`,其中参数 `df1` 表示输入的 DataFrame 对象。
5. 获取数据集中除了最后一列(即目标变量)以外的所有特征名,并存入 `feature_names` 变量中。
6. 创建一个 3 行 4 列的子图,子图大小为 20x15,存入 `fig` 和 `axs` 变量中。
7. 针对每个特征,分别绘制直方图,并将其放置在对应的子图中。
8. 设置每个子图的标题、横轴和纵轴标签以及刻度大小。
9. 对每个直方图的 patch(即每个柱子)进行设置,使其边框宽度为 2,颜色为黑色。
10. 调整子图间的间距,保存图片,并显示绘制结果。
需要注意的是,这段代码假设数据集中的特征名称都不包含空格,否则在设置横轴标签时可能会出错。此外,这段代码只适用于数据集中的特征都是数值型的情况,对于分类特征或文本特征需要进行相应的处理才能绘制直方图。
相关推荐









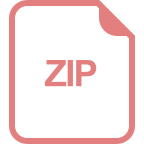