编写一个python的五子棋代码
时间: 2024-03-02 18:25:04 浏览: 64
下面是一个简单的 Python 五子棋代码,仅供参考:
```python
import numpy as np
# 定义棋盘大小
BOARD_SIZE = 15
# 定义黑白棋子的标志
EMPTY = 0
BLACK = 1
WHITE = 2
# 定义一个棋盘类
class Board:
def __init__(self):
self.board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=int)
# 获取棋盘上指定位置的棋子颜色
def get(self, x, y):
return self.board[x][y]
# 在棋盘上放置棋子
def put(self, x, y, player):
self.board[x][y] = player
# 判断指定位置是否为空
def is_empty(self, x, y):
return self.board[x][y] == EMPTY
# 判断指定位置是否在棋盘内
def is_on_board(self, x, y):
return x >= 0 and x < BOARD_SIZE and y >= 0 and y < BOARD_SIZE
# 判断棋盘上是否有五子连珠
def is_win(self, player):
# 横向检查
for x in range(BOARD_SIZE):
for y in range(BOARD_SIZE - 4):
if self.board[x][y] == player and self.board[x][y+1] == player and self.board[x][y+2] == player and self.board[x][y+3] == player and self.board[x][y+4] == player:
return True
# 竖向检查
for x in range(BOARD_SIZE - 4):
for y in range(BOARD_SIZE):
if self.board[x][y] == player and self.board[x+1][y] == player and self.board[x+2][y] == player and self.board[x+3][y] == player and self.board[x+4][y] == player:
return True
# 斜向检查
for x in range(BOARD_SIZE - 4):
for y in range(BOARD_SIZE - 4):
if self.board[x][y] == player and self.board[x+1][y+1] == player and self.board[x+2][y+2] == player and self.board[x+3][y+3] == player and self.board[x+4][y+4] == player:
return True
for x in range(4, BOARD_SIZE):
for y in range(BOARD_SIZE - 4):
if self.board[x][y] == player and self.board[x-1][y+1] == player and self.board[x-2][y+2] == player and self.board[x-3][y+3] == player and self.board[x-4][y+4] == player:
return True
return False
# 定义一个游戏类
class Game:
def __init__(self):
self.board = Board()
self.current_player = BLACK
# 切换当前玩家
def switch_player(self):
if self.current_player == BLACK:
self.current_player = WHITE
else:
self.current_player = BLACK
# 玩家落子
def player_put(self, x, y):
if self.board.is_on_board(x, y) and self.board.is_empty(x, y):
self.board.put(x, y, self.current_player)
if self.board.is_win(self.current_player):
print("Player", self.current_player, "wins!!!")
return True
else:
self.switch_player()
return False
# 开始游戏
def play():
game = Game()
while True:
x, y = map(int, input("Player " + str(game.current_player) + ", please input your move (x, y): ").split())
if game.player_put(x, y):
break
if __name__ == '__main__':
play()
```
使用该代码,可以在控制台上玩五子棋。每次轮到玩家输入落子的位置,输入格式为 `x y`(中间有一个空格),例如 `7 7` 表示在第 7 行、第 7 列放置棋子。当有一方获得五子连珠时,游戏结束。
阅读全文
相关推荐
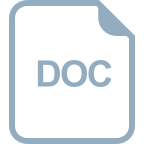
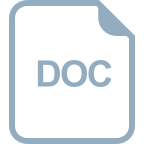
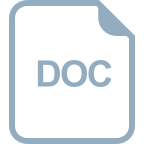








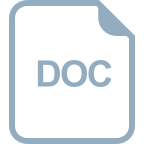
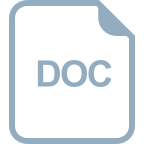
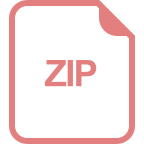
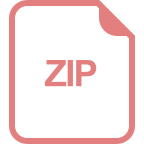
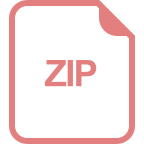
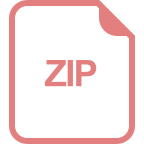
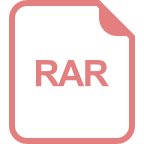