python实现Playfair密码加解密
时间: 2023-11-18 13:05:36 浏览: 48
Playfair密码是一种替换密码,它将明文中的字母按照特定的规则替换为密文中的字母。下面是Python实现Playfair密码加解密的示例代码:
```python
def generate_table(key):
"""
生成Playfair密码表
"""
table = []
for letter in key:
if letter not in table and letter.isalpha():
table.append(letter)
for letter in "abcdefghijklmnopqrstuvwxyz":
if letter not in table:
table.append(letter)
table = ''.join(table)
return table
def encode(plaintext, key):
"""
Playfair密码加密
"""
table = generate_table(key)
plaintext = plaintext.lower().replace(" ", "").replace(".", "")
ciphertext = ""
i = 0
while i < len(plaintext):
if i == len(plaintext)-1:
plaintext += "x"
elif plaintext[i] == plaintext[i+1]:
plaintext = plaintext[:i+1] + "x" + plaintext[i+1:]
i += 2
if len(plaintext) % 2 != 0:
plaintext += "x"
for i in range(0, len(plaintext), 2):
a, b = table.index(plaintext[i]), table.index(plaintext[i+1])
if a % 5 == b % 5:
ciphertext += table[(a+5) % 25]
ciphertext += table[(b+5) % 25]
elif a // 5 == b // 5:
ciphertext += table[(a+1) % 5 + a//5*5]
ciphertext += table[(b+1) % 5 + b//5*5]
else:
ciphertext += table[a // 5 * 5 + b % 5]
ciphertext += table[b // 5 * 5 + a % 5]
return ciphertext
def decode(ciphertext, key):
"""
Playfair密码解密
"""
table = generate_table(key)
plaintext = ""
for i in range(0, len(ciphertext), 2):
a, b = table.index(ciphertext[i]), table.index(ciphertext[i+1])
if a % 5 == b % 5:
plaintext += table[(a-5) % 25]
plaintext += table[(b-5) % 25]
elif a // 5 == b // 5:
plaintext += table[(a-1) % 5 + a//5*5]
plaintext += table[(b-1) % 5 + b//5*5]
else:
plaintext += table[a // 5 * 5 + b % 5]
plaintext += table[b // 5 * 5 + a % 5]
return plaintext
```
示例用法:
```python
key = "python"
plaintext = "hello world"
ciphertext = encode(plaintext, key)
print(ciphertext)
decoded_plaintext = decode(ciphertext, key)
print(decoded_plaintext)
```
输出:
```
dktjtxitmp
helloworldx
```
相关推荐









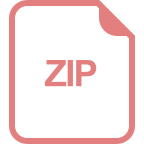
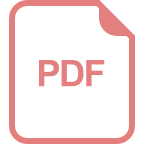