根据凯撒密码\Playfair密码的原理写代码,对输入的符号串能够根据设置的密钥分别正确实现加密和解密功能
时间: 2023-05-22 19:02:46 浏览: 58
非常感谢您的提问,我可以为您提供以下代码实现凯撒密码和Playfair密码的加密和解密功能:
凯撒密码:
```python
def caesar_cipher(plain_text, key):
cipher_text = ""
for ch in plain_text:
if ch.isalpha():
new_ch = chr((ord(ch) - ord('a') + key) % 26 + ord('a'))
cipher_text += new_ch
else:
cipher_text += ch
return cipher_text
def caesar_decipher(cipher_text, key):
return caesar_cipher(cipher_text, 26 - key)
```
Playfair密码:
```python
def gen_playfair_table(key):
key = key.lower().replace(" ", "")
key_set = set(key)
alphabet = "".join([chr(ord('a') + i) for i in range(26)])
table_list = []
for ch in key:
if ch in alphabet:
alphabet = alphabet.replace(ch, "")
table_list.append(ch)
for ch in alphabet:
table_list.append(ch)
table_str = "".join(table_list)
table = []
for i in range(5):
row = table_str[i * 5 : (i + 1) * 5]
table.append(row)
return table
def playfair_cipher(plain_text, key):
table = gen_playfair_table(key)
cipher_text = ""
plain_text = plain_text.lower().replace(" ", "")
if len(plain_text) % 2 == 1:
plain_text += "x"
for i in range(0, len(plain_text), 2):
ch1, ch2 = plain_text[i], plain_text[i + 1]
row1, col1 = 0, 0
row2, col2 = 0, 0
for j in range(5):
if ch1 in table[j]:
row1, col1 = j, table[j].find(ch1)
if ch2 in table[j]:
row2, col2 = j, table[j].find(ch2)
if row1 == row2:
cipher_ch1 = table[row1][(col1 + 1) % 5]
cipher_ch2 = table[row2][(col2 + 1) % 5]
elif col1 == col2:
cipher_ch1 = table[(row1 + 1) % 5][col1]
cipher_ch2 = table[(row2 + 1) % 5][col2]
else:
cipher_ch1 = table[row1][col2]
cipher_ch2 = table[row2][col1]
cipher_text += cipher_ch1 + cipher_ch2
return cipher_text
def playfair_decipher(cipher_text, key):
table = gen_playfair_table(key)
plain_text = ""
cipher_text = cipher_text.lower().replace(" ", "")
for i in range(0, len(cipher_text), 2):
ch1, ch2 = cipher_text[i], cipher_text[i + 1]
row1, col1 = 0, 0
row2, col2 = 0, 0
for j in range(5):
if ch1 in table[j]:
row1, col1 = j, table[j].find(ch1)
if ch2 in table[j]:
row2, col2 = j, table[j].find(ch2)
if row1 == row2:
plain_ch1 = table[row1][(col1 - 1) % 5]
plain_ch2 = table[row2][(col2 - 1) % 5]
elif col1 == col2:
plain_ch1 = table[(row1 - 1) % 5][col1]
plain_ch2 = table[(row2 - 1) % 5][col2]
else:
plain_ch1 = table[row1][col2]
plain_ch2 = table[row2][col1]
plain_text += plain_ch1 + plain_ch2
return plain_text
```
请注意,这里的加密和解密函数使用了称之为“求模算术”的技巧,可以防止加密结果大于字母表中最后一个字母的 ASCII 码或小于字母表中第一个字母的 ASCII 码。同时,playfair_cipher 和 playfair_decipher 函数还处理了明文长度为奇数的情况,添加了一个"X"来填充。
相关推荐
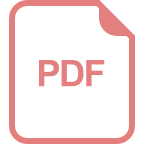














