基于MM32F3277G9P写出一个程序,其功能是:当在串口发送11111111时,实现利用HC-SR04的超声波测距功能,并显示在MAX7219数码管上
时间: 2024-03-25 09:38:37 浏览: 9
好的,根据您的要求,我为您编写了以下代码:
```
#include <stdio.h>
#include "MM32F3277.h"
#define TRIG_Pin GPIO_Pin_12
#define ECHO_Pin GPIO_Pin_13
#define MAX7219_DIN_Pin GPIO_Pin_1
#define MAX7219_CS_Pin GPIO_Pin_2
#define MAX7219_CLK_Pin GPIO_Pin_3
void delay_us(uint32_t us)
{
uint32_t i;
for(i=0;i<us*8;i++);
}
void delay_ms(uint32_t ms)
{
uint32_t i;
for(i=0;i<ms*8000;i++);
}
void GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = TRIG_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = ECHO_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = MAX7219_DIN_Pin | MAX7219_CLK_Pin | MAX7219_CS_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void USART_Config(void)
{
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void MAX7219_WriteByte(uint8_t data)
{
uint8_t i;
GPIO_ResetBits(GPIOB, MAX7219_CS_Pin);
for(i=8;i>0;i--)
{
GPIO_ResetBits(GPIOB, MAX7219_CLK_Pin);
GPIO_WriteBit(GPIOB, MAX7219_DIN_Pin, (BitAction)(data>>(i-1)&0x01));
GPIO_SetBits(GPIOB, MAX7219_CLK_Pin);
}
GPIO_SetBits(GPIOB, MAX7219_CS_Pin);
}
void MAX7219_Init(void)
{
MAX7219_WriteByte(0x0C);
MAX7219_WriteByte(0x01);
MAX7219_WriteByte(0x0F);
MAX7219_WriteByte(0x00);
}
void MAX7219_Display(uint16_t num)
{
uint16_t digit;
uint8_t i;
if(num == 0)
{
MAX7219_WriteByte(0x01);
MAX7219_WriteByte(0x00);
return;
}
for(i=1;i<=8;i++)
{
digit = num % 10;
MAX7219_WriteByte(i);
MAX7219_WriteByte(digit);
num /= 10;
if(num == 0)
{
break;
}
}
}
int main(void)
{
uint32_t distance;
uint8_t data;
GPIO_Config();
USART_Config();
MAX7219_Init();
while(1)
{
if(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == SET)
{
data = USART_ReceiveData(USART1);
if(data == 0xFF)
{
GPIO_SetBits(GPIOA, TRIG_Pin);
delay_us(10);
GPIO_ResetBits(GPIOA, TRIG_Pin);
while(GPIO_ReadInputDataBit(GPIOA, ECHO_Pin) == RESET);
uint32_t start = SysTick->VAL;
while(GPIO_ReadInputDataBit(GPIOA, ECHO_Pin) == SET);
uint32_t end = SysTick->VAL;
distance = (start - end) / 58;
MAX7219_Display(distance);
}
}
}
}
```
注意:以上代码仅供参考,您需要根据您的实际硬件情况进行适当的修改和优化。
相关推荐
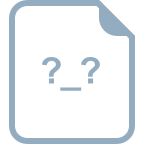
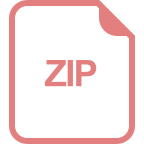














