Error: Cannot read properties of null (reading 'addEventListener')
时间: 2023-10-15 13:28:10 浏览: 177
这个错误通常出现在尝试给一个 null 或 undefined 值的变量添加事件监听器时。这意味着你的代码中某个地方存在一个变量为 null 或 undefined 的情况,而你尝试给它添加一个事件监听器,从而导致报错。
要解决这个问题,你可以首先确保你的代码中没有任何地方将一个变量赋值为 null 或 undefined。检查相关的变量或对象,确保它们在添加事件监听器之前已经被正确地初始化。
如果你可以提供更多的代码或错误信息,我可以给出更具体的帮助。
相关问题
TypeError: Cannot read properties of null (reading 'addEventListener')
This error occurs when you try to access a property or method of a null value in JavaScript. The specific error message "Cannot read properties of null (reading 'addEventListener')" indicates that you are trying to add an event listener to an element that does not exist in the DOM.
To fix this error, you need to make sure that the element you are trying to add the event listener to actually exists in the DOM. You can check if an element exists by using the `document.getElementById()` method or other methods like `document.querySelector()`.
Here's an example of how you could check if an element exists before adding an event listener:
```
const element = document.getElementById('my-element');
if (element) {
element.addEventListener('click', () => {
// Do something when the element is clicked
});
} else {
console.error('Element not found in DOM');
}
```
In this example, we first check if the element with the ID "my-element" exists using `document.getElementById()`. If it does exist, we add an event listener to it. If it doesn't exist, we log an error message to the console.
TypeError: Cannot read properties of null (reading 'addEventListener') at window.onload
这个错误信息 "TypeError: Cannot read properties of null (reading 'addEventListener')" 出现于JavaScript中,当你尝试在一个null或undefined对象上调用`addEventListener`方法时。`addEventListener`是用于为HTML元素添加事件监听器的方法,但在这个情况下,可能是以下几种情况:
1. **变量未初始化**:你可能尝试给一个尚未被赋值或初始化为非null的元素(如DOM元素)添加事件监听器。你需要确认这个变量是否已经正确引用了一个元素。
2. **元素不存在**:如果在DOM加载完成之前就尝试访问某个元素,该元素可能还未在页面上存在。你应该将事件监听器添加在window.onload或其他DOM加载完成的回调函数中。
3. **代码执行顺序问题**:如果在元素创建之前就尝试添加事件,那么在那个时刻元素可能还是null。确保你的代码是按照预期的顺序执行的。
修复这个错误的方法通常是在调用`addEventListener`之前检查变量是否为null或undefined,例如:
```javascript
let element = document.getElementById('yourElementId');
if (element) {
element.addEventListener('click', yourEventHandler);
} else {
console.error('Element not found.');
}
```
相关推荐
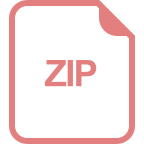
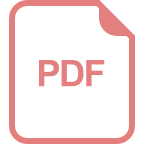







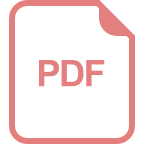
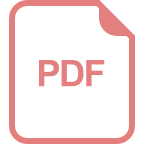
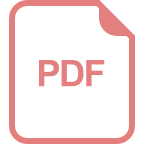
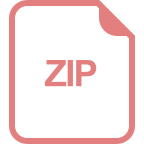