请用C#写贪吃蛇游戏
时间: 2023-08-10 17:11:40 浏览: 205
以下是使用C#编写的简单贪吃蛇游戏代码,仅供参考:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Threading;
namespace ConsoleApp1
{
class Program
{
static int width = 20;
static int height = 20;
static int score = 0;
static int[,] grid = new int[width, height];
static List<Point> snake = new List<Point>();
static Point food = new Point();
static Random rnd = new Random();
static void Main(string[] args)
{
InitGame();
while (true)
{
DrawGrid();
DrawSnake();
DrawFood();
MoveSnake();
if (CheckCollision())
{
Console.Clear();
Console.WriteLine("Game over!");
Console.WriteLine("Score: " + score);
Console.ReadKey();
break;
}
Thread.Sleep(100);
}
}
static void InitGame()
{
snake.Add(new Point(width / 2, height / 2));
grid[snake[0].x, snake[0].y] = 1;
food = GenerateFood();
}
static void DrawGrid()
{
Console.Clear();
for (int i = 0; i < height + 2; i++)
{
for (int j = 0; j < width + 2; j++)
{
if (i == 0 || i == height + 1 || j == 0 || j == width + 1)
Console.Write("#");
else
Console.Write(" ");
}
Console.WriteLine();
}
Console.WriteLine("Score: " + score);
}
static void DrawSnake()
{
foreach (Point p in snake)
{
Console.SetCursorPosition(p.x + 1, p.y + 1);
Console.Write("*");
}
}
static void DrawFood()
{
Console.SetCursorPosition(food.x + 1, food.y + 1);
Console.Write("@");
}
static void MoveSnake()
{
Point head = new Point(snake[0].x, snake[0].y);
switch (Console.ReadKey().Key)
{
case ConsoleKey.LeftArrow:
head.x--;
break;
case ConsoleKey.RightArrow:
head.x++;
break;
case ConsoleKey.UpArrow:
head.y--;
break;
case ConsoleKey.DownArrow:
head.y++;
break;
}
snake.Insert(0, head);
if (head.x == food.x && head.y == food.y)
{
score++;
food = GenerateFood();
}
else
{
snake.RemoveAt(snake.Count - 1);
}
}
static bool CheckCollision()
{
if (snake[0].x == 0 || snake[0].x == width + 1 || snake[0].y == 0 || snake[0].y == height + 1)
return true;
for (int i = 1; i < snake.Count; i++)
{
if (snake[0].x == snake[i].x && snake[0].y == snake[i].y)
return true;
}
return false;
}
static Point GenerateFood()
{
Point p = new Point(rnd.Next(1, width + 1), rnd.Next(1, height + 1));
while (grid[p.x, p.y] == 1)
{
p.x = rnd.Next(1, width + 1);
p.y = rnd.Next(1, height + 1);
}
return p;
}
class Point
{
public int x;
public int y;
public Point(int x, int y)
{
this.x = x;
this.y = y;
}
}
}
}
```
阅读全文
相关推荐
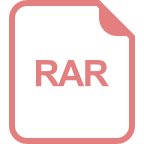
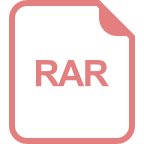
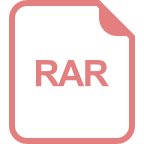
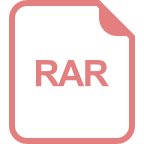
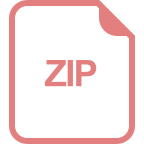
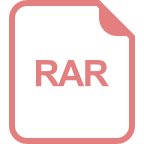
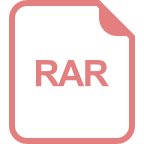
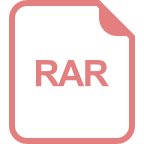
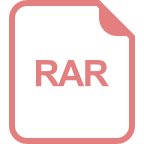
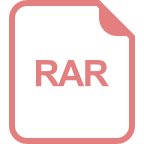
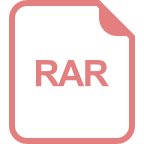
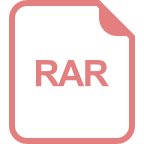
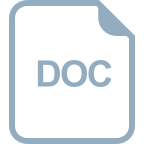
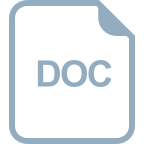
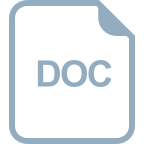
