用C#写一个贪吃蛇游戏
时间: 2023-11-23 19:04:38 浏览: 45
好的,以下是一个简单的贪吃蛇游戏的C#代码:
```csharp
using System;
using System.Collections.Generic;
namespace SnakeGame
{
class Program
{
static void Main(string[] args)
{
Console.CursorVisible = false;
Console.SetWindowSize(40, 20);
// 初始化蛇的起始位置
List<Position> snake = new List<Position>()
{
new Position(20, 10),
new Position(19, 10),
new Position(18, 10)
};
// 初始化食物位置
Position food = new Position();
Random random = new Random();
food.X = random.Next(0, Console.WindowWidth);
food.Y = random.Next(0, Console.WindowHeight);
// 初始化移动方向
Direction direction = Direction.Right;
// 游戏开始
while (true)
{
Console.Clear();
// 绘制蛇和食物
Console.ForegroundColor = ConsoleColor.Yellow;
Console.SetCursorPosition(food.X, food.Y);
Console.Write("$");
Console.ForegroundColor = ConsoleColor.Green;
foreach (Position p in snake)
{
Console.SetCursorPosition(p.X, p.Y);
Console.Write("#");
}
// 蛇移动
Position head = snake[0];
Position newHead = new Position(head.X, head.Y);
switch (direction)
{
case Direction.Up:
newHead.Y--;
break;
case Direction.Down:
newHead.Y++;
break;
case Direction.Left:
newHead.X--;
break;
case Direction.Right:
newHead.X++;
break;
}
snake.Insert(0, newHead);
snake.RemoveAt(snake.Count - 1);
// 检查是否吃到食物
if (head.X == food.X && head.Y == food.Y)
{
// 增加蛇的长度,并重新生成食物位置
snake.Add(new Position(food.X, food.Y));
food.X = random.Next(0, Console.WindowWidth);
food.Y = random.Next(0, Console.WindowHeight);
}
// 检查是否游戏结束
if (head.X < 0 || head.X >= Console.WindowWidth || head.Y < 0 || head.Y >= Console.WindowHeight)
{
Console.SetCursorPosition(10, 10);
Console.ForegroundColor = ConsoleColor.Red;
Console.Write("Game Over!");
break;
}
for (int i = 1; i < snake.Count; i++)
{
if (head.X == snake[i].X && head.Y == snake[i].Y)
{
Console.SetCursorPosition(10, 10);
Console.ForegroundColor = ConsoleColor.Red;
Console.Write("Game Over!");
return;
}
}
// 检查键盘输入并改变移动方向
if (Console.KeyAvailable)
{
ConsoleKeyInfo key = Console.ReadKey(true);
switch (key.Key)
{
case ConsoleKey.UpArrow:
if (direction != Direction.Down)
direction = Direction.Up;
break;
case ConsoleKey.DownArrow:
if (direction != Direction.Up)
direction = Direction.Down;
break;
case ConsoleKey.LeftArrow:
if (direction != Direction.Right)
direction = Direction.Left;
break;
case ConsoleKey.RightArrow:
if (direction != Direction.Left)
direction = Direction.Right;
break;
}
}
// 控制游戏速度
System.Threading.Thread.Sleep(100);
}
}
// 枚举移动方向
enum Direction
{
Up,
Down,
Left,
Right
}
// 位置类
class Position
{
public int X { get; set; }
public int Y { get; set; }
public Position(int x, int y)
{
X = x;
Y = y;
}
public Position()
{
X = 0;
Y = 0;
}
}
}
}
```
上述代码使用了 `List` 类型来表示蛇的身体,使用了 `Random` 类型生成食物的位置,使用了 `Console` 类型来控制控制台的输出。在游戏过程中,使用了 `Thread.Sleep` 方法来控制游戏速度。游戏的结束条件是蛇碰到边界或者碰到自己的身体。
相关推荐
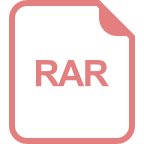














